Java Serialization: Overcoming Versioning Challenges
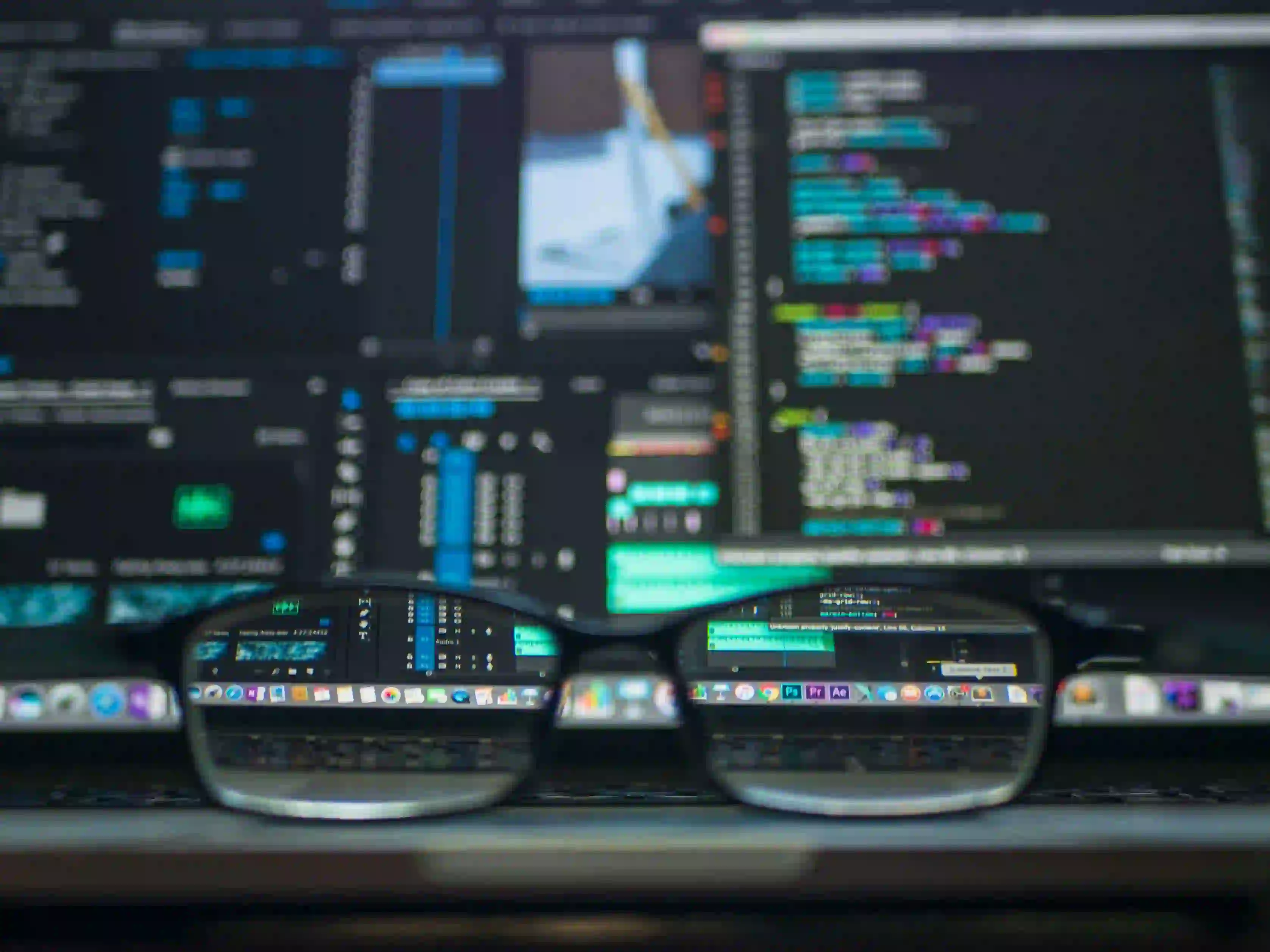
Java Serialization: Overcoming Versioning Challenges
In the world of software development, data preservation and transfer are key components. Java, as one of the leading programming languages, provides a mechanism known as serialization to convert objects into a byte stream, facilitating easy storage or transmission. However, while this process is invaluable, it poses its own unique challenges, particularly in the area of versioning. This post aims to elaborate on the intricacies of Java serialization and share best practices to navigate versioning challenges.
What is Serialization?
Serialization is the process of converting an object into a format that can be easily persisted or transmitted. In Java, serialization is accomplished using the Serializable
interface. When an object is serialized, its state is converted into a byte stream, which can then be saved to a file, a database, or transmitted over a network.
Here’s a simple example of a Java class that implements serialization:
import java.io.Serializable;
public class Person implements Serializable {
private static final long serialVersionUID = 1L; // Versioning
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and toString() method
public String getName() { return name; }
public int getAge() { return age; }
@Override
public String toString() {
return "Person{name='" + name + "', age=" + age + '}';
}
}
Why Use Serializable
?
- Ease of Storage: Objects can be easily stored in files or databases.
- Network Transmission: Objects can be transferred over the network in a binary format.
- Object Reconstitution: Later, the serialized objects can be reconstituted back into their original form.
The Importance of serialVersionUID
The serialVersionUID
is a unique identifier for each class. It is crucial in maintaining version compatibility. If a class definition changes, and the serialVersionUID
does not match the serialized object, a InvalidClassException
will be thrown upon deserialization. This is a common source of confusion in Java serialization.
private static final long serialVersionUID = 2L; // Change this when you modify the class
By explicitly declaring a serialVersionUID
, you define a version for your class, ensuring that changes to the class structure do not break serialization.
Versioning Challenges in Serialization
As applications evolve, classes undergo various changes such as field additions, removals, or modifications. This is where versioning challenges arise. Some typical versions of challenges include:
-
Field Addition or Removal: When fields are added or removed, it can affect the serialization process. New fields will have default values, and removed fields may cause exceptions.
-
Field Type Changes: Changing a field's data type can lead to deserialization errors. Java tries to match types, and if they are incompatible, it will fail.
-
Class Hierarchies: If you have subclasses, new versions of classes may need to manage dependencies.
Strategies for Managing Versioning Challenges
To effectively overcome versioning challenges, consider the following strategies:
1. Use Default
Values
When adding new fields, provide default values. This will help in cases where an object is deserialized that does not contain the new field.
private String email = "not.provided@example.com"; // Default value
2. Add readObject()
and writeObject()
Implementing custom serialization methods lets you control how objects are serialized and deserialized. This allows you to handle missing fields or provide default values.
Example:
private void writeObject(java.io.ObjectOutputStream out) throws IOException {
out.defaultWriteObject(); // Uses default serialization
out.writeInt(age); // Custom serialization of age
}
private void readObject(java.io.ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject(); // Uses default deserialization
if (age == 0) {
age = 18; // Provide default value for deserialized age
}
}
3. Using transient
Fields
If certain fields should not be serialized (perhaps for security reasons), you can mark them as transient
. They will be ignored during serialization.
private transient String password; // Will not be serialized
4. Consider Using Externalization
Instead of relying on Java’s default serialization, consider using the Externalizable
interface. This gives you full control over the serialization process. Externalizable requires you to implement writeExternal
and readExternal
.
Here’s an example:
import java.io.Externalizable;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
public class User implements Externalizable {
private String username;
private String email;
public User() { } // Default constructor for Externalizable
public User(String username, String email) {
this.username = username;
this.email = email;
}
@Override
public void writeExternal(ObjectOutput out) throws IOException {
out.writeObject(username);
out.writeObject(email);
}
@Override
public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException {
username = (String) in.readObject();
email = (String) in.readObject();
}
}
Additional Resources
To delve deeper into Java serialization and best practices, consider resources such as:
Lessons Learned
Java serialization is a powerful tool for object persistence and transmission, yet it is fraught with challenges, particularly concerning versioning. Through careful management of serialVersionUID
, thoughtful defaults, custom serialization methods, and the appropriate usage of transient or externalizable fields, developers can successfully navigate these challenges.
Be proactive about versioning. If your application evolves— and it will— the strategies outlined can help you minimize the risks associated with serialization. With these considerations in mind, your Java applications can effectively preserve state, ensuring that data remains intact and usable across different versions.
Serializing effectively, even during updates, is essential for robust software systems. Implement the practices discussed and ensure smooth transitions as your Java applications evolve.
By adhering to these practices, you can maintain the integrity of your serialized data while scaling your Java applications into the future. Happy coding!