Handling Checked Exceptions in Java Lambdas: A Guide
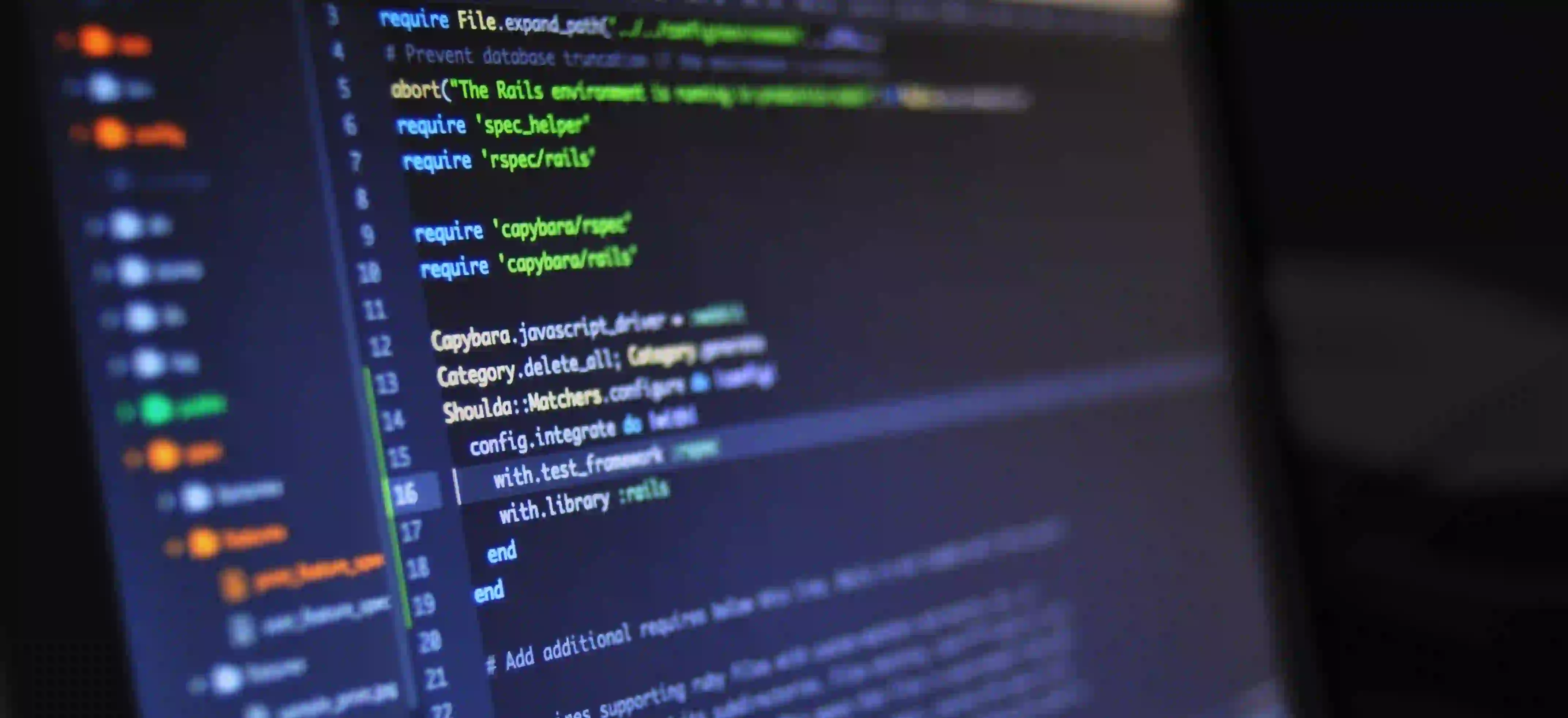
Handling Checked Exceptions in Java Lambdas: A Guide
Java is known for its strong type system and robust exception handling. However, combining these features with functional programming constructs like lambdas can create complexities, especially when dealing with checked exceptions. In this guide, we’ll explore how to effectively handle checked exceptions within Java lambdas.
What Are Checked Exceptions?
Checked exceptions are exceptions that must be either caught or declared in the method signature. They derive from the Exception
class but are not descendants of RuntimeException
. Examples include IOException
, SQLException
, and FileNotFoundException
. Failing to handle checked exceptions will prompt the Java compiler to throw an error.
Why is Exception Handling Important?
Proper exception handling ensures that applications remain stable and predictable. It can help in:
- Logging Errors: Capture critical information for troubleshooting.
- User Feedback: Provide meaningful messages to users when things go awry.
- Control Flow: Manage application flow during unexpected circumstances.
The Challenge with Lambda Expressions
Lambdas provide a concise way to write instances of functional interfaces using a single expression. However, they don’t work well with checked exceptions straight out of the box. Consider this example:
Runnable example = () -> {
throw new IOException(); // Compilation error
};
The above code will not compile because IOException
is a checked exception, causing a compilation error.
Solution: Handling Checked Exceptions
There are a few strategies to manage checked exceptions in lambda expressions effectively, as shown below:
1. Wrap Checked Exceptions in Unchecked Exceptions
One of the simplest ways to handle checked exceptions in lambdas is to wrap them in unchecked exceptions. This way, the lambda can throw exceptions without the need for explicit declaration.
Here is a practical example:
@FunctionalInterface
interface CheckedRunnable {
void run() throws Exception;
}
public class ExceptionHandling {
public static void main(String[] args) {
CheckedRunnable checkedRunnable = () -> {
throw new IOException("IO Exception occurred");
};
try {
execute(checkedRunnable);
} catch (RuntimeException e) {
System.out.println("Caught a runtime exception: " + e.getCause().getMessage());
}
}
public static void execute(CheckedRunnable checkedRunnable) {
try {
checkedRunnable.run();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
Here, CheckedRunnable
is a functional interface capable of throwing exceptions. When an exception occurs, we wrap it in a RuntimeException
, which allows us to handle it gracefully in the execute
method.
2. Using Custom Wrapper Functional Interfaces
Another strategy involves creating custom functional interfaces designed to handle checked exceptions. Let's define a functional interface for a method that can throw checked exceptions.
@FunctionalInterface
interface CheckedSupplier<T> {
T get() throws Exception;
}
Here’s how we can use this interface in practice:
public class CustomCheckedSupplier {
public static void main(String[] args) {
CheckedSupplier<String> supplier = () -> {
if (Math.random() > 0.5) {
throw new SQLException("Can't connect to the database.");
}
return "Connection Successful";
};
String result = safeExecute(supplier);
System.out.println(result);
}
public static <T> T safeExecute(CheckedSupplier<T> supplier) {
try {
return supplier.get();
} catch (Exception e) {
return "An error occurred: " + e.getMessage();
}
}
}
In this code snippet, CheckedSupplier
is used to encapsulate a method that can throw checked exceptions. The safeExecute
method handles any thrown exceptions and returns a fallback result.
3. Using Java 8 or Higher - Optionals
If applicable, you can leverage Optional
as a means of gracefully handling potential exceptions where the absence of a value needs to be communicated instead.
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
Optional<String> result = safeGetValue(() -> {
if (Math.random() > 0.5) throw new IOException("File not found");
return "Value retrieved successfully.";
});
result.ifPresent(System.out::println);
}
public static Optional<String> safeGetValue(CheckedSupplier<String> supplier) {
try {
return Optional.of(supplier.get());
} catch (Exception e) {
return Optional.empty();
}
}
}
The Optional
class provides a means of representing optional values (or the absence thereof) without explicitly dealing with exceptions in the calling code.
A Final Look
Java's checked exceptions can introduce challenges in lambda expressions, but with thoughtful design, they can also be effectively managed. By using custom functional interfaces, wrapping exceptions, and leveraging constructs like Optional
, you can make your code more robust and maintainable.
For further reading on Java lambdas and exception handling, check out Oracle’s official documentation and Java's Exception Handling guide.
Summary
- Checked Exceptions must be handled or declared.
- Lambdas do not allow checked exceptions directly.
- Solution strategies include wrapping checked exceptions in unchecked exceptions or using custom functional interfaces.
- Ensure to provide valuable feedback and avoid program crashes through proper handling.
By embracing these strategies, you'll build safer, cleaner, and more maintainable Java applications that adeptly manage exceptions both within traditional and functional paradigms. Happy coding!