Common Pitfalls in Java's Automatic Resource Management
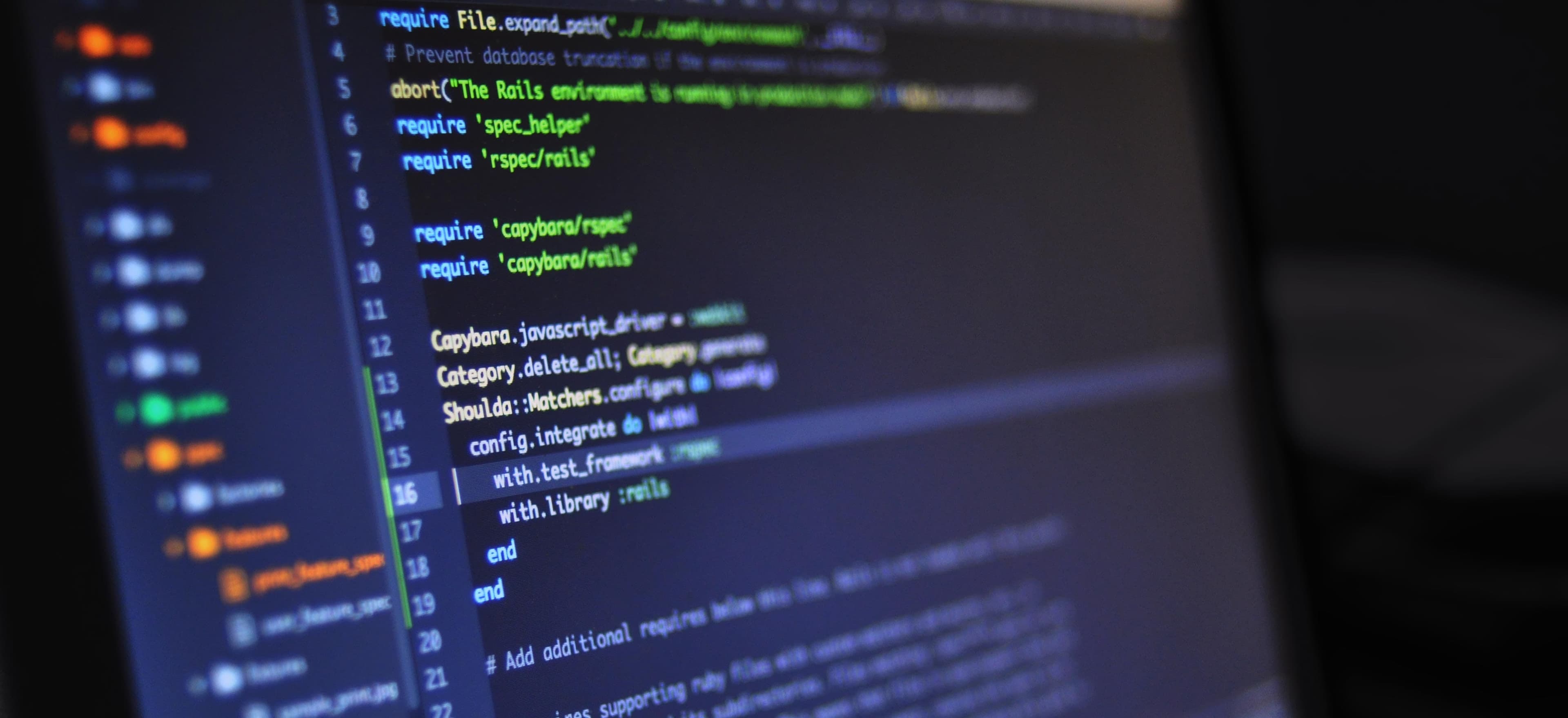
- Published on
Common Pitfalls in Java's Automatic Resource Management
Java's Automatic Resource Management (ARM) makes resource handling a lot easier and safer. However, like many features, it has its pitfalls that developers must navigate to avoid potential issues. In this blog post, we will explore the common pitfalls of ARM, providing insightful examples and best practices to ensure you make the most of this fantastic feature.
Understanding Automatic Resource Management
Automatic Resource Management in Java is primarily implemented through the try-with-resources statement, introduced in Java 7. This feature ensures that resources like files, sockets, and database connections are closed automatically.
Here’s a simple example:
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
In the above snippet, BufferedReader
will be closed automatically when the block ends, even if an exception occurs. This blocks the need for verbose finally statements to close resources.
Common Pitfalls in ARM
1. Ignoring Resource Types
Not all resources support automatic closing. Your class must implement the java.lang.AutoCloseable
or java.io.Closeable
interface. Failure to acknowledge this can lead to unexpected behavior.
// This class does not implement AutoCloseable
class MyResource {
public void doSomething() {
System.out.println("Doing something");
}
}
// The compiler will not let you use MyResource in try-with-resources
try (MyResource res = new MyResource()) {
res.doSomething();
} catch (Exception e) {
e.printStackTrace();
}
Why This Matters: Always ensure your resource implements the necessary interface to be closed automatically. Documentation is key; refer to the Java documentation for details.
2. Multiple Resources Handling
When using multiple resources in a single try-with-resources statement, it can be easy to overlook the order in which resources are closed. They are closed in the reverse order of their declaration, which might lead to dependency issues.
try (
InputStream in = new FileInputStream("input.txt");
BufferedReader reader = new BufferedReader(new InputStreamReader(in))
) {
// Accessing resources
} catch (IOException e) {
e.printStackTrace();
}
// In case of exceptions, if BufferedReader throws before InputStream is closed
Why This Matters: If InputStream
is closed first and an exception occurs in BufferedReader
, any operations dependent on InputStream
will fail. Be cautious and understand the dependencies among resources.
3. Handling Exceptions Properly
Another pitfall is mishandling exceptions during resource management. If an exception occurs in the try block, it can mask another exception thrown during resource closing.
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
// Deliberately causing an exception
String line = reader.readLine();
throw new RuntimeException("Exception in reading");
} catch (IOException e) {
// This will handle a separate closing exception
System.out.println("Closing exception: " + e.getMessage());
}
Why This Matters: You may not be aware of resource management issues if they don’t surface due to a prior exception. Always catch and print or log exceptions for better clarity.
4. Not Reusing Resources
One of the overlooked aspects of ARM is frequent creation and disposal of resources, which can lead to performance overhead. It’s advisable to manage long-lived resources carefully rather than creating them on-the-fly.
class ResourceManager {
private BufferedReader reader;
public void init() throws FileNotFoundException {
reader = new BufferedReader(new FileReader("file.txt"));
}
public void readFile() {
try (BufferedReader tempReader = reader) {
// Process the reader
} catch (IOException e) {
e.printStackTrace();
}
}
public void cleanup() throws IOException {
if (reader != null) {
reader.close();
}
}
}
Why This Matters: Instead of creating a new BufferedReader
each time, reusing a single instance can enhance performance significantly, particularly for larger applications or files.
5. Complex Resource Management Logic
It’s easy to get tangled in complex resource management scenarios, which can lead to either leaks or premature releases. Always strive for simplicity in your design.
try (
Connection conn = DriverManager.getConnection("url");
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM users")
) {
// ResultSet is handled here
} catch (SQLException e) {
e.printStackTrace();
} // Ensure all resources are released properly
Why This Matters: When using several interconnected resources, it's advisable to create utility classes that encapsulate these for better manageability and clarity. This can help separate concerns and clarify the resource's lifecycle.
Best Practices for Using ARM
-
Always Implement AutoCloseable: Ensure custom resources implement the
AutoCloseable
interface. -
Log Exceptions: Utilize logging best practices to manage exceptions thoroughly.
-
Use Smaller Try Blocks: Maintain smaller try blocks where possible. This can help isolate resource use and potential exceptions.
-
Separate Resource Initialization and Cleanup: Consider separating the logic for initialization and cleanup to improve readability and manageability.
-
Understand Closing Order: Always be aware of the order in which resources are closed, particularly when handling multiple resources.
To Wrap Things Up
Automatic Resource Management in Java enhanced resource handling significantly, reducing boilerplate code and the chances of resource leaks. However, developers must take care to avoid potential pitfalls and follow best practices to ensure optimal resource management.
By implementing robust error handling, being mindful of the resource types, and using coding patterns that prioritize clarity, the full benefits of ARM can be achieved. For more in-depth resources, refer to the Oracle’s Java Tutorials for a comprehensive guide on this topic.
Internalizing these best practices will allow developers to utilize ARM effectively, contributing to cleaner, more maintainable Java codebases. Happy coding!
Checkout our other articles