Understanding SynchronizedList: Common Concurrency Pitfalls
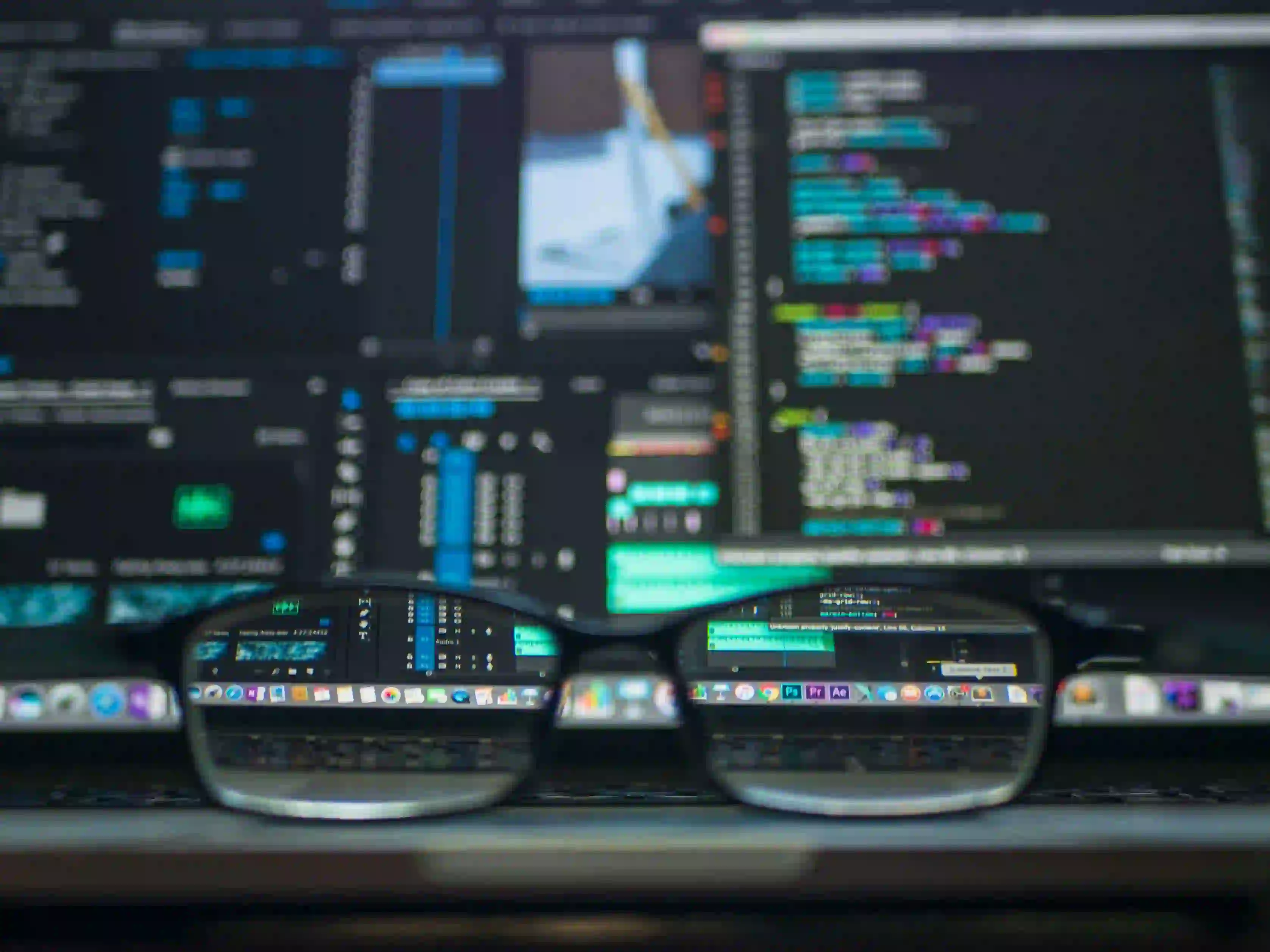
Understanding SynchronizedList
: Common Concurrency Pitfalls
In the world of multi-threaded programming, concurrency and thread safety are paramount. When multiple threads access shared resources, unpredictable problems can arise if these accesses are not managed correctly. In Java, the SynchronizedList
class provides a way to achieve thread safety for lists, but it is crucial to understand its implementation and potential pitfalls. In this blog post, we will explore SynchronizedList
, discuss its advantages and limitations, and provide code examples to help you understand how to use it effectively.
What is SynchronizedList
?
SynchronizedList
is part of the Java Collections Framework and can be obtained by using the Collections.synchronizedList(List<T> list)
method. This method returns a thread-safe list backed by the specified list. It synchronizes all operations that modify the list using a single lock.
Key Features:
- Thread Safety: Guarantees safe access from multiple threads.
- Wrapper: It acts as a wrapper around a regular List.
- Minimal Overhead: Offers a synchronized approach without the need for manual locks.
import java.util.*;
public class SynchronizedListExample {
public static void main(String[] args) {
List<String> list = Collections.synchronizedList(new ArrayList<>());
// Adding items to the list
list.add("Element 1");
list.add("Element 2");
System.out.println("Synchronized List: " + list);
}
}
Code Explanation:
The code initializes a synchronized list by wrapping an ArrayList
. This synchronization is essential when multiple threads access the list. Without it, operations like add()
may cause data corruption or exceptions when accessed concurrently.
How Does It Work?
Internally, SynchronizedList
utilizes intrinsic locks to synchronize access to its methods. Each method in the list is synchronized, ensuring only one thread can execute it at a time. However, this leads to a fundamental concurrency pitfall - while the methods are thread-safe, compound operations may not be safe.
// Performing compound operations
synchronized (list) {
// Check existence, then add
if (!list.contains("Element 3")) {
list.add("Element 3");
}
}
In the above example, the synchronization block around the compound operation ensures that no other thread can modify the list while you're checking for existence and then adding the item.
Common Pitfalls with SynchronizedList
1. Compound Operations Not Atomic
While each individual method call is synchronized and thus thread-safe, combining multiple operations that should be considered as atomic (like checking if an element exists and then adding it) can lead to race conditions. A simultaneous execution could lead to unexpected results.
2. Iteration Issues
When iterating over a SynchronizedList
, you may run into concurrency issues. Here’s a quick example to illustrate this:
for (String element : list) {
System.out.println(element);
// Not safe: another thread may modify the list here
if (element.equals("Element 2")) {
list.add("Element 4");
}
}
Exception Warning
This code may throw a ConcurrentModificationException
if another thread modifies the list while it is being iterated.
Safe Iteration
To safely iterate through a synchronized list, you must use an explicit synchronization block:
synchronized (list) {
for (String element : list) {
System.out.println(element);
// Safe to modify within synchronized block
}
}
3. Blocking Issues
Excessive use of SynchronizedList
can lead to performance bottlenecks as threads wait for access to the list. In highly concurrent environments, the overhead of synchronizing access can outweigh its benefits. Alternatives such as CopyOnWriteArrayList
or ConcurrentHashMap
may be better suited depending on your use case.
Choosing the Right Concurrent Collection
Java provides various concurrent collection classes that can offer better performance characteristics depending on the scenario. Here’s a brief overview:
| Collection Class | Description |
|--------------------------------|-----------------------------------------------------------------|
| CopyOnWriteArrayList
| Ideal for read-heavy operations. Modifications result in a new array copy. |
| ConcurrentHashMap
| Thread-safe map providing better scalability than SynchronizedList
. |
| BlockingQueue
| Designed for producer-consumer scenarios, allowing for safe queue operations. |
Best Practices for Using SynchronizedList
-
Minimize the Scope of Synchronization: Only synchronize critical sections of the code that manipulate the list.
-
Avoid Nested Synchronization: Be cautious when synchronizing multiple collections. Each additional lock increases the chance of deadlocks.
-
Redundant Synchronization: Do not synchronize on both the outer structure and the
SynchronizedList
. This can cause unwanted blocking.
// Not recommended
synchronized (outerLock) {
synchronized (list) {
list.add("Element 5");
}
}
-
Use Alternative Concurrent Collections: If you find yourself using
SynchronizedList
for complex interactions, consider using other concurrent data structures. -
Thorough Testing: Always test your multi-threaded code under various thread counts to uncover potential race conditions and anomalies.
A Final Look
Java's SynchronizedList
is a powerful tool for achieving thread safety in applications, but it is essential to understand its limitations and potential pitfalls. By adopting best practices and considering alternatives for specific use cases, developers can greatly enhance the reliability and performance of their multi-threaded Java applications.
For further reading on concurrency in Java, check out Java Concurrency in Practice and the official Java documentation.
Feel free to reach out with questions or share experiences you've had while working with synchronized lists, and happy coding!