Resolving the Java NoClassDefFoundError: A Complete Guide
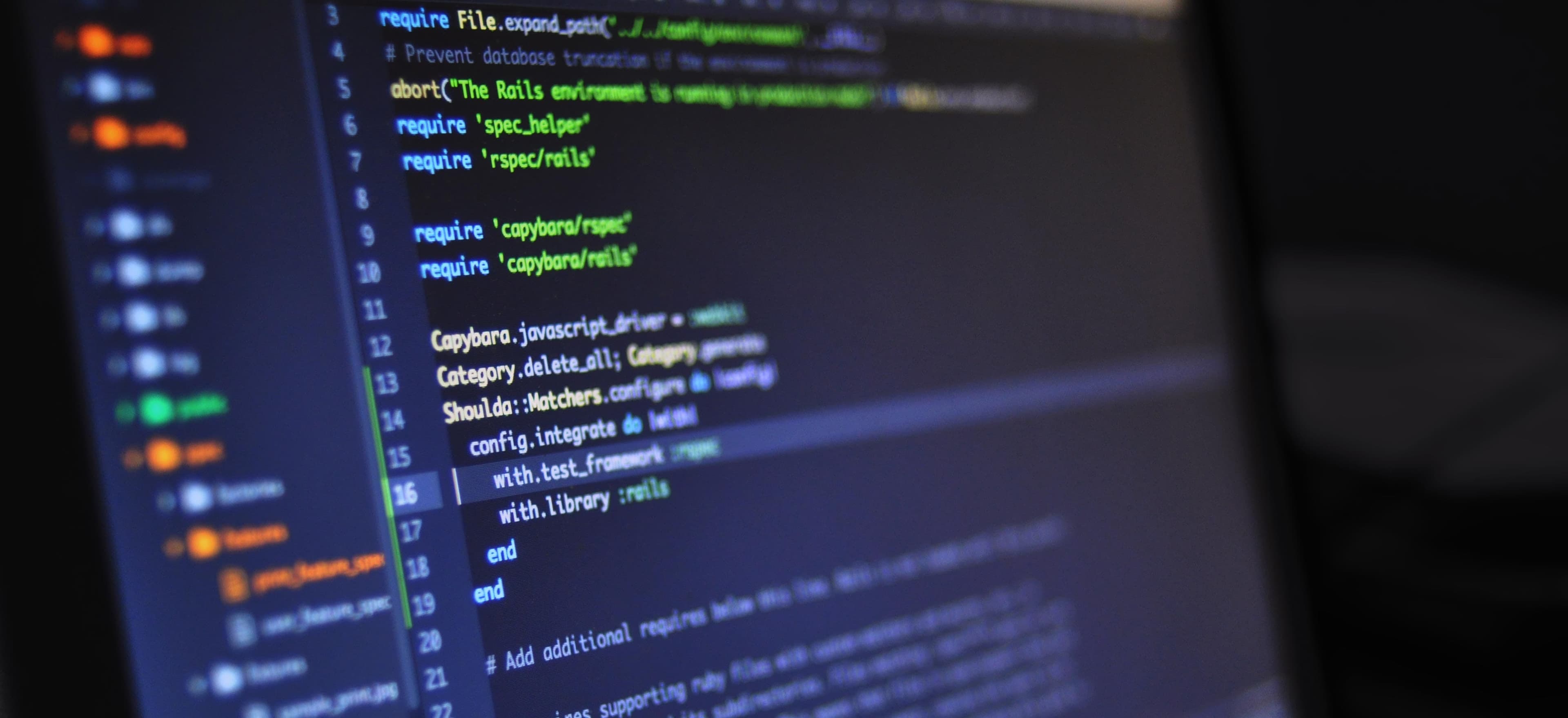
- Published on
Resolving the Java NoClassDefFoundError: A Complete Guide
Java developers often run into a maze of exceptions and errors as they build applications. One of the most perplexing errors is the infamous NoClassDefFoundError. This issue can derail an application’s runtime, making it critical for developers to understand what it is and how to resolve it. In this guide, we'll delve into what causes this error, its implications, and how to fix it effectively.
Understanding NoClassDefFoundError
What is NoClassDefFoundError?
In Java, NoClassDefFoundError
is an error thrown by the Java Virtual Machine (JVM) when it tries to load a class and fails to find the necessary bytecode for that class. This can happen due to a variety of issues, including:
- The class was present during compile time but is missing at runtime.
- The class exists, but a required dependency is missing.
- Classpath issues resulting in the class not being found.
It is important to distinguish between NoClassDefFoundError
and ClassNotFoundException
. While both indicate that the Java application cannot find a class, ClassNotFoundException
is thrown during runtime when an application tries to load a class dynamically. In contrast, NoClassDefFoundError
originates from failing to locate the class file after it was successfully compiled.
Common Scenarios Leading to NoClassDefFoundError
Here are some common situations where developers might encounter this error:
-
Class Not Included in Classpath: The class is not part of the compilation or runtime classpath.
-
Version Mismatch: A newer or older version of a class file is being referenced in the application that isn't compatible.
-
JAR File Issues: The JAR file containing the class is missing or corrupted.
-
Compile-time and Runtime Discrepancies: The application might have compiled successfully, but missing classes are not available during runtime.
-
Static Initializers: If a static initializer fails, the class may not be loaded.
Now that we have a better understanding of what leads to this error, let's explore how to diagnose and resolve it.
Diagnosing NoClassDefFoundError
Step 1: Analyze Your Classpath
One of the first things to check is the classpath configuration in your Java project. The classpath tells the JVM where to look for classes and packages.
- For a typical Java application, ensure that all required JAR files are included.
- If using an IDE like IntelliJ or Eclipse, check the project settings to ensure all dependencies are accounted for under the build path or dependencies section.
Step 2: Check for Dependency Issues
If your project relies on external libraries, verify that all dependencies are correctly included and that the version numbers in your build configuration file match the expected versions.
For Maven projects, you might run:
mvn dependency:tree
This command shows all dependencies and helps identify any conflicts or missing artifacts.
Step 3: Look for Compilation Warnings and Errors
Sometimes, missing classes might not throw clear errors in the IDE. Make sure to check your project's build process for warnings or errors that might indicate compilation issues affecting the output class files.
javac MyClass.java
Step 4: Review Static Initializers
If your class contains static initializers, any exception thrown during their execution will lead the JVM to not load the class. Check and log any potential exceptions that may arise during initialization.
public class Example {
static {
if (someCondition) {
throw new RuntimeException("Static initialization failed.");
}
}
}
Resolving NoClassDefFoundError
Now that we've diagnosed the problem, let's discuss potential solutions.
Solution 1: Ensuring Correct Classpath
Make sure that your classpath is accurately set, including all paths to the necessary JAR files.
For a command-line application, you can specify the classpath using:
java -cp "yourClasspath:otherClasspath" YourMainClass
For instance:
java -cp "lib/*:bin" com.example.Main
Solution 2: Rebuild Your Project
In some cases, project build artifacts can become corrupted or outdated. Rebuilding your project can clear up such issues.
For Maven users:
mvn clean install
Solution 3: Fix Dependency Issues in Build Tool
If using Gradle or Maven, ensure that all dependencies are properly resolved. Update your dependencies or resolve any conflicts in your pom.xml
or build.gradle
.
For instance, in Maven you can do:
<dependencies>
<dependency>
<groupId>org.example</groupId>
<artifactId>your-library</artifactId>
<version>1.0.0</version>
</dependency>
</dependencies>
Make sure the versions align with what your code expects.
Solution 4: Distribute the Required Libraries
In situations where you're deploying your application, ensure that all the dependent JARs are available in the deployment environment. Use tools like Maven Shade Plugin to create an "uber" JAR that contains all dependencies.
Maven Shade Plugin Example:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-shade-plugin</artifactId>
<version>3.2.4</version>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>shade</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Solution 5: Use a Logging Framework
Integrating a logging framework can help you capture the exact point of failure and identify if the class loading is being interrupted by other issues.
For example, using SLF4J and Logback, you can log the class loading events and any issues that arise.
Bringing It All Together
NoClassDefFoundError can be a daunting stumbling block for Java developers. However, understanding its roots, common scenarios, and how to diagnose and fix these issues is crucial for smooth application development. By ensuring that your classpath is correctly configured, managing dependencies appropriately, and leveraging logging, you can effectively navigate and resolve this error.
Further Reading
For those looking to deepen their understanding of Java error handling, consider checking out the following resources:
- Java ClassLoader Documentation
- Managing Dependencies with Maven
- Logging in Java
By adhering to the guidelines outlined in this guide, you will be well-equipped to tackle NoClassDefFoundError
and ensure a more robust Java application. Happy coding!
Checkout our other articles