Understanding Java Atomic Operations: Key Limitations Explored
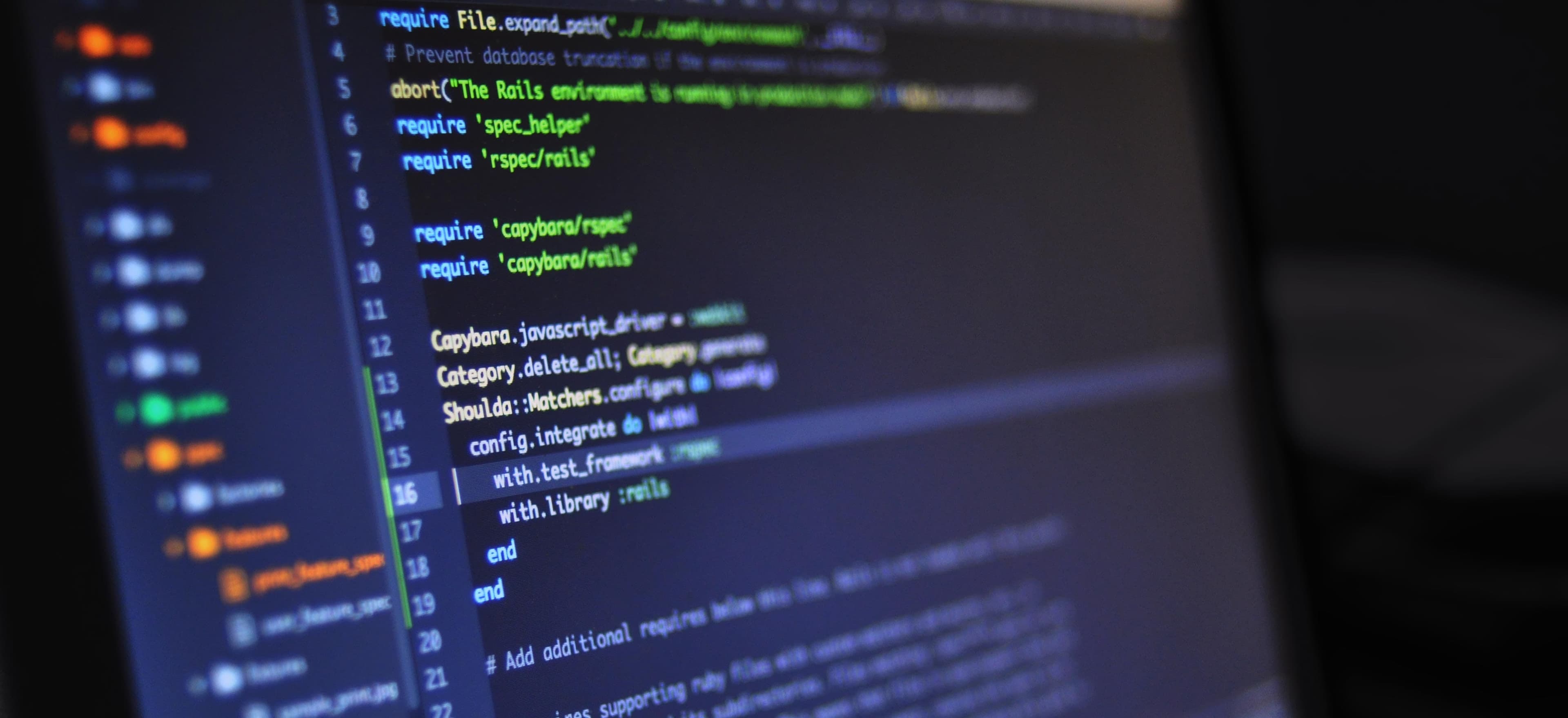
- Published on
Understanding Java Atomic Operations: Key Limitations Explored
In the realm of concurrent programming, ensuring thread safety is paramount. Java offers a variety of mechanisms to handle concurrency, with atomic operations being one of the most efficient. However, understanding the limitations of these atomic operations is crucial for developing robust multi-threaded applications. In this blog post, we will delve into the nature of atomic operations in Java, explore their advantages, and highlight key limitations that developers should consider.
What are Atomic Operations?
Atomic operations are those that complete in a single step from the perspective of other threads. In simpler terms, an atomic operation is performed entirely or not at all, ensuring data integrity in multithreaded environments. Java provides several atomic classes in the java.util.concurrent.atomic
package, allowing developers to perform non-blocking operations.
Why Use Atomic Operations?
-
Improved Performance: Atomic operations do not require locking, which can lead to reduced contention among threads. This improves overall application performance.
-
Simplicity: Using atomic operations can simplify the code since they eliminate the need for synchronized blocks or methods.
-
Non-blocking: Since atomic operations are non-blocking, they do not cause threads to wait, which can reduce deadlock scenarios.
Key Atomic Classes in Java
Java provides multiple classes for atomic operations:
- AtomicInteger: A class for integers that supports atomic operations.
- AtomicBoolean: A class for boolean flags.
- AtomicReference: A generic reference type supporting atomic operations.
Example: AtomicInteger
Let's look at a simple example of using AtomicInteger
for a counter.
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicCounter {
private AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet(); // Atomically increments by 1
}
public int getCount() {
return count.get(); // Returns the current count value
}
public static void main(String[] args) {
AtomicCounter counter = new AtomicCounter();
counter.increment();
System.out.println("Count: " + counter.getCount());
}
}
Commentary
incrementAndGet()
: This method increments the current value by 1 and returns the updated value atomically.get()
: Retrieves the current value of the counter without modifying it. This is an essential operation when you need to read the atomic variable safely.
Limitations of Atomic Operations
While atomic operations in Java can significantly improve performance and simplify code, they have notable limitations that developers must be aware of.
1. Limited Atomicity
Atomic operations are atomic only at the level of individual variable updates. If your logic requires atomicity across multiple variables or multi-step operations, atomic classes will not suffice. For example:
import java.util.concurrent.atomic.AtomicInteger;
public class ComplexOperation {
private AtomicInteger countA = new AtomicInteger(0);
private AtomicInteger countB = new AtomicInteger(0);
public void updateCounts() {
// This operation is NOT atomic across countA and countB
countA.incrementAndGet();
countB.incrementAndGet();
}
}
In this case, if updateCounts
is called by multiple threads, it could lead to inconsistent states between countA
and countB
. Developers must use synchronization when performing multi-variable updates.
2. Non-Transactional Nature
Unlike some other languages and frameworks that offer transactional memory, Java's atomic operations do not support complex transactions. If a series of actions must either complete entirely or not at all, Java's atomic classes cannot inherently facilitate this. For example, if you need to perform a series of updates that should all succeed or fail together, a simple atomic operation won't cut it.
3. Volatile Variables vs. Atomic Operations
Many Java developers may confuse volatile
variables and atomic operations. While both can be used to handle certain concurrency scenarios, they serve different purposes. Volatile variables ensure that changes are visible across threads but do not guarantee atomicity.
Consider the following:
public class VolatileExample {
private volatile boolean flag = false;
public void setFlag() {
flag = true; // This write is atomic but not the whole operation
}
public boolean isFlagSet() {
return flag; // Reads the current value atomically
}
}
While the read and write to flag
appear atomic, there is no guarantee that observing changes using isFlagSet
will see the result of multiple operations correctly synchronized.
4. Performance Overhead
Even though atomic operations are faster than traditional synchronized blocks, they still come with overhead. Operations performed using CAS (Compare-And-Swap) can lead to high contention scenarios that might degrade performance. This is often referred to as the "ABA problem," where a variable may change between the time it is read and updated, leading to erroneous state.
Closing the Chapter
Java's atomic operations are a powerful tool for concurrent programming, but they are not a silver bullet. Understanding their limitations is crucial for creating efficient and safe multi-threaded applications. When designing your systems, keep in mind the following:
- Use atomic operations for single-variable updates that require no additional synchronization.
- Be wary of operations requiring atomic transactions across multiple variables.
- Consider the use of more robust concurrency controls such as locks or
java.util.concurrent
collections for complex scenarios.
By leveraging atomic operations judiciously and acknowledging their limitations, developers can achieve a well-balanced approach to concurrency in Java.
Additional Resources
For further reading on Java concurrency and atomic operations, check out these resources:
- Java Concurrency in Practice - An insightful book on concurrency in Java.
- Java Documentation on Atomic Variables - Official API documentation for Java's atomic classes.
Feel free to share your experiences and insights about using atomic operations in Java in the comments below!
Checkout our other articles