Navigating Java SecurityManager: Overcoming Permission Challenges
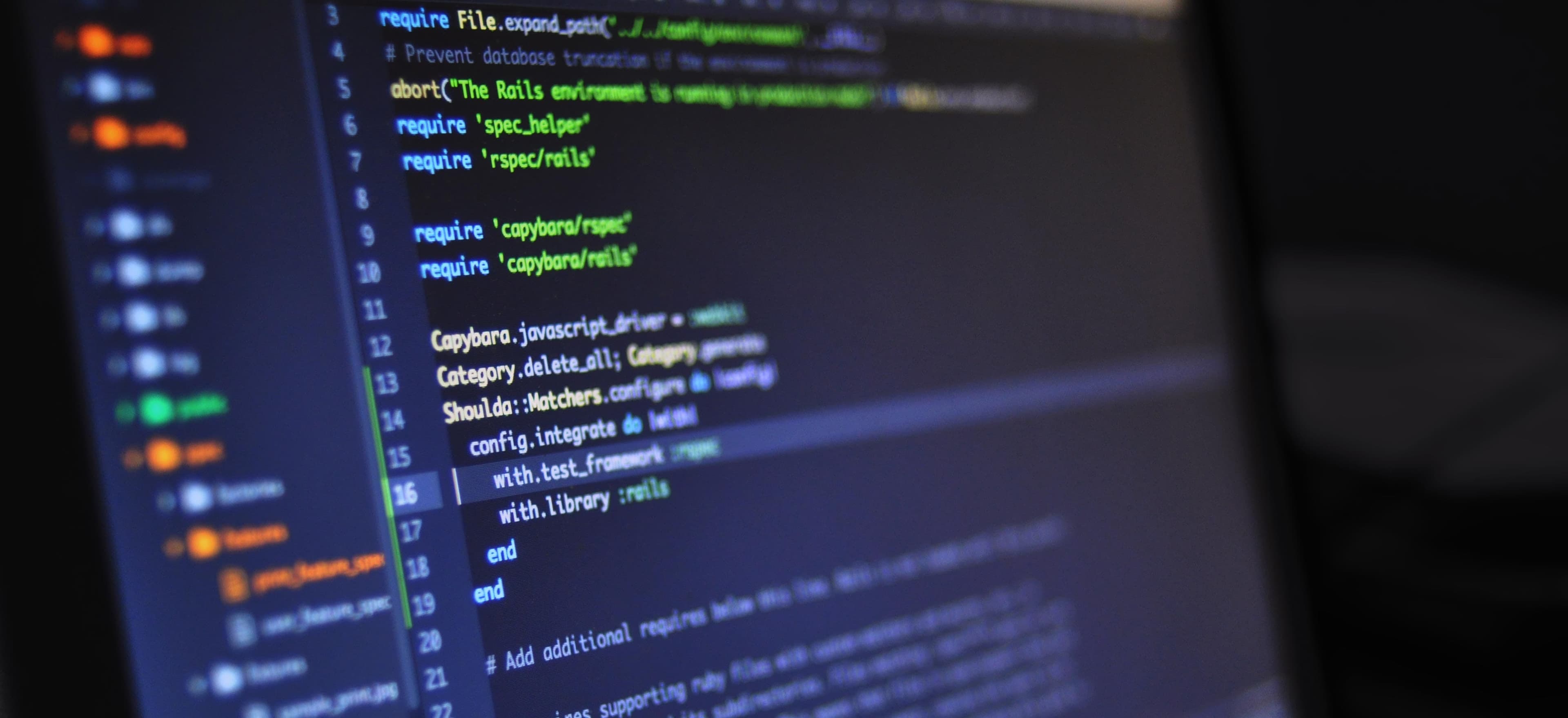
- Published on
Navigating Java SecurityManager: Overcoming Permission Challenges
Java, one of the most robust programming languages widely used for building secure applications, offers a powerful feature called the SecurityManager. This mechanism provides a way to implement a security policy for your Java applications. However, navigating through the intricacies of permission-based security can be challenging. In this blog post, we will delve into the concepts surrounding Java's SecurityManager, common permission challenges, and practical solutions with illustrative code snippets.
1. Understanding SecurityManager
The Java SecurityManager is a critical component that dictates the permissions granted to classes. When you run a Java application, the SecurityManager allows you to enforce a security policy that determines which operations are permitted and which are not. By default, the SecurityManager is not enabled in Java applications. It can be activated by using the -Djava.security.manager
option when starting the Java Virtual Machine (JVM).
Here’s a simple example of how to enable a SecurityManager:
public class SecurityManagerDemo {
public static void main(String[] args) {
System.setSecurityManager(new SecurityManager());
// Application logic goes here
}
}
In this code snippet, we utilize System.setSecurityManager
to instantiate a new SecurityManager. However, it's essential to note that setting a SecurityManager can greatly impact the behavior of your application, so it's crucial to have the permissions correctly configured.
2. Common Permission Challenges
2.1. Permissions Overview
Java permissions are categorized into several types, such as file read/write permissions, network access, and system property access. Each permission is defined in the java.security.Permission
class hierarchy. When the SecurityManager is in place, any request made by your application for these permissions is intercepted and evaluated against the granted permissions set in the security policy file.
public class FileAccessDemo {
public static void main(String[] args) {
System.setSecurityManager(new SecurityManager());
try {
System.getProperty("user.home");
System.out.println("Permission granted to access user.home property.");
} catch (SecurityException e) {
System.err.println("Permission denied: " + e.getMessage());
}
}
}
In this example, we attempt to access the user’s home directory property. If the application does not have permission for this operation, a SecurityException will be thrown.
2.2. Security Policy File
To mitigate permission issues, you can create a security policy file that defines what permissions your application requires. Here’s an example of a simple policy file:
grant {
permission java.util.PropertyPermission "user.home", "read";
permission java.io.FilePermission "/path/to/resource", "read, write";
permission java.net.SocketPermission "localhost:1024-", "connect, resolve";
};
This policy file grants specific permissions to access system properties, file operations, and network connectivity. To set this policy file when starting the JVM, use:
java -Djava.security.manager -Djava.security.policy=/path/to/policy.file YourMainClass
3. Overcoming Permission Challenges
3.1. Diagnosing Permission Issues
Understanding the cause of permission issues can significantly streamline the debugging process. When the SecurityManager denies an operation, it throws a SecurityException
. You can catch this exception to provide more clarity on what went wrong.
public class PermissionDiagnoser {
public static void main(String[] args) {
System.setSecurityManager(new SecurityManager());
try {
System.loadLibrary("exampleLibrary");
} catch (SecurityException e) {
System.err.println("Security violation detected: " + e.getMessage());
}
}
}
By capturing the exception and printing an explanatory message, you can identify where your application is lacking necessary permissions.
3.2. Fine-tuning Permissions
Sometimes, modifying a few lines in the policy file can resolve issues without exposing the application to unnecessary risks. Fine-tuning the permissions ensures that your application maintains security while having the necessary functionality.
For instance, if your application needs to access temporary directories, add the following line to the policy file:
permission java.io.FilePermission "/temp/-", "read, write";
This allows read and write access to all files in the /temp
directory while keeping other file manipulations restricted.
3.3. Using Code Permissions
For more complex applications, it may be beneficial to check an application's permissions programmatically. This can be especially useful in modular applications where different modules may require varying levels of security.
Here's an example code snippet that checks for permission before performing a file operation:
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class PermissionCheck {
public static void main(String[] args) {
System.setSecurityManager(new SecurityManager());
File file = new File("/path/to/file");
try {
System.getSecurityManager().checkRead(file.getPath());
FileReader reader = new FileReader(file);
// Reading file content
reader.close();
} catch (SecurityException e) {
System.err.println("Read access denied: " + e.getMessage());
} catch (IOException e) {
System.err.println("I/O error occurred: " + e.getMessage());
}
}
}
This example demonstrates how to check if the application has the necessary permissions before attempting to read a file, preventing SecurityException
and handling I/O exceptions cleanly.
4. Conclusion
Navigating the Java SecurityManager can be tricky, especially when tackling permission challenges. However, understanding the fundamentals of permissions, creating well-defined security policies, and programmatically checking for permissions can mitigate many potential issues. By implementing the strategies detailed in this post, developers can harness the power of Java's built-in security features without compromising their application’s functionality.
To learn more about Java security, it’s worth exploring the official Java Security Documentation for additional insights. For a deeper dive into best practices and advanced configurations, consider reviewing Oracle’s Best Practices for Security.
With the right approach, you can effectively safeguard your Java applications while retaining full control over their permissions. Embrace the challenge, and let your Java applications thrive in a secure environment.
Checkout our other articles