Mastering Java Annotations: Common Runtime Challenges Explained
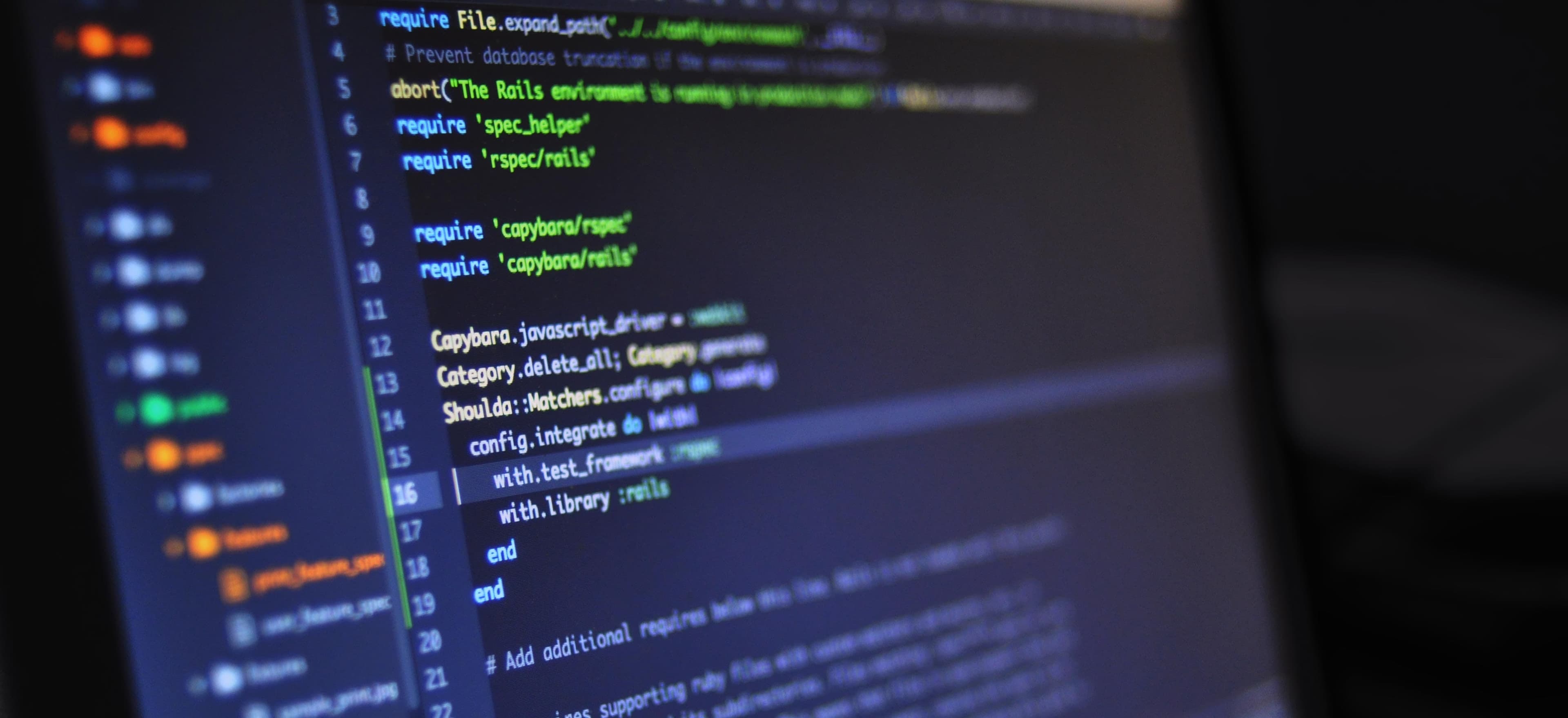
- Published on
Mastering Java Annotations: Common Runtime Challenges Explained
Java annotations are a fundamental part of the Java programming language that enables metadata to be associated with program elements. They provide a powerful way to implement custom functionalities, facilitate code analysis, and implement frameworks. Despite their advantages, developers often encounter runtime challenges when using annotations. In this blog post, we’ll explore the common runtime challenges associated with Java annotations, and how to effectively resolve them.
Understanding Java Annotations
Before diving into the challenges, let's briefly summarize what Java annotations are. Annotations are special metadata that you can add to classes, methods, fields, parameters, and other program elements. They have no direct effect on the operation of the code they annotate but can provide information to tools and frameworks that process this metadata.
Java provides several built-in annotations, such as @Override
, @Deprecated
, and @SuppressWarnings
. You can also define your custom annotations with ease.
Defining a Custom Annotation
Here is a simple example of defining a custom annotation:
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface CustomAnnotation {
String value();
}
In this code:
@Retention(RetentionPolicy.RUNTIME)
indicates that the annotation should be available at runtime.@Target(ElementType.METHOD)
specifies that this annotation can only be applied to methods.
Common Runtime Challenges with Annotations
1. Reflection-Based Access
One of the most common challenges is accessing annotation data at runtime. Since annotations can only be read via reflection, this can lead to performance issues or runtime exceptions if not handled properly.
Example of Accessing Annotations
Here’s how you might access the CustomAnnotation
from a method:
import java.lang.reflect.Method;
public class AnnotationProcessor {
public void processAnnotations(Class<?> clazz) {
for (Method method : clazz.getDeclaredMethods()) {
if (method.isAnnotationPresent(CustomAnnotation.class)) {
CustomAnnotation annotation = method.getAnnotation(CustomAnnotation.class);
System.out.println("Method: " + method.getName() + ", Value: " + annotation.value());
}
}
}
}
In this snippet, we use reflection to cycle through methods of a class, check for our custom annotation, and print its value if present. Be cautious because reflection can introduce performance overhead.
2. Dealing With Inheritance
A frequent pitfall is the misconception of how annotations work in class inheritance. By default, annotations are not inherited by subclasses.
Making Annotations Inheritable
To solve this, you can define your annotation's inheritance behavior by using the @Inherited
meta-annotation:
import java.lang.annotation.Inherited;
@Inherited
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
public @interface InheritedAnnotation {
String value();
}
@InheritedAnnotation("Base")
class BaseClass {}
class SubClass extends BaseClass {}
In this example, the SubClass
will inherit the InheritedAnnotation
from BaseClass
, allowing easier access when working with class hierarchies.
3. Retention Policy Misunderstandings
Another common issue arises from misusing retention policies. Developers often expect runtime access to annotations only marked with RetentionPolicy.RUNTIME
.
Choosing the Right Strategy
SOURCE
: Annotations are discarded by the compiler.CLASS
: Annotations are stored in the class file but not available at runtime.RUNTIME
: Annotations are available during execution through reflection.
For example, if you define an annotation without specifying the retention policy, it defaults to CLASS
, making it inaccessible at runtime.
4. Annotation Processor Limitations
Java provides the annotation processing tool (APT), allowing you to process annotations during build time. However, it can introduce limitations.
Example of Using APT
import javax.annotation.processing.*;
import javax.lang.model.SourceVersion;
import javax.lang.model.element.TypeElement;
import java.util.Set;
@SupportedAnnotationTypes("CustomAnnotation")
@SupportedSourceVersion(SourceVersion.RELEASE_8)
public class CustomAnnotationProcessor extends AbstractProcessor {
@Override
public boolean process(Set<? extends TypeElement> annotations, RoundEnvironment roundEnv) {
// Processing logic here
return true; // Indicating processing is complete
}
}
Using APT has restrictions such as inability to access runtime values. Keep this in mind when your application's functionality hinges upon dynamically evaluated annotations.
5. Handling Multiple Annotations
Combining multiple annotations can sometimes lead to conflicting behaviors or unintended side effects.
Example of Combining Annotations
While it's possible to use multiple annotations on a single method, care must be taken to understand how each annotation operates:
@CustomAnnotation("First")
@AnotherAnnotation("Second")
public void combinedAnnotationsMethod() {
// Method implementation
}
It's important to have a strategy or logic in place that respects the intended behaviors of each annotation.
Bringing It All Together
Java annotations offer powerful features for developers, but they come with their share of runtime challenges. Understanding these challenges and knowing how to mitigate them is crucial for effective Java programming.
By leveraging reflection responsibly, understanding inheritance behaviors, and utilizing proper retention policies, you can master the use of annotations in your applications.
References:
Going Further
For a deeper dive into Java annotations and how they can be utilized effectively, consider reading articles on Java Design Patterns or exploring the possibilities of Spring Annotations.
Let us continue to learn, create, and master Java annotations, and in doing so, enhance our coding proficiency while navigating these challenges.
Checkout our other articles