Streams vs Readers/Writers: Choosing the Right Java I/O Method
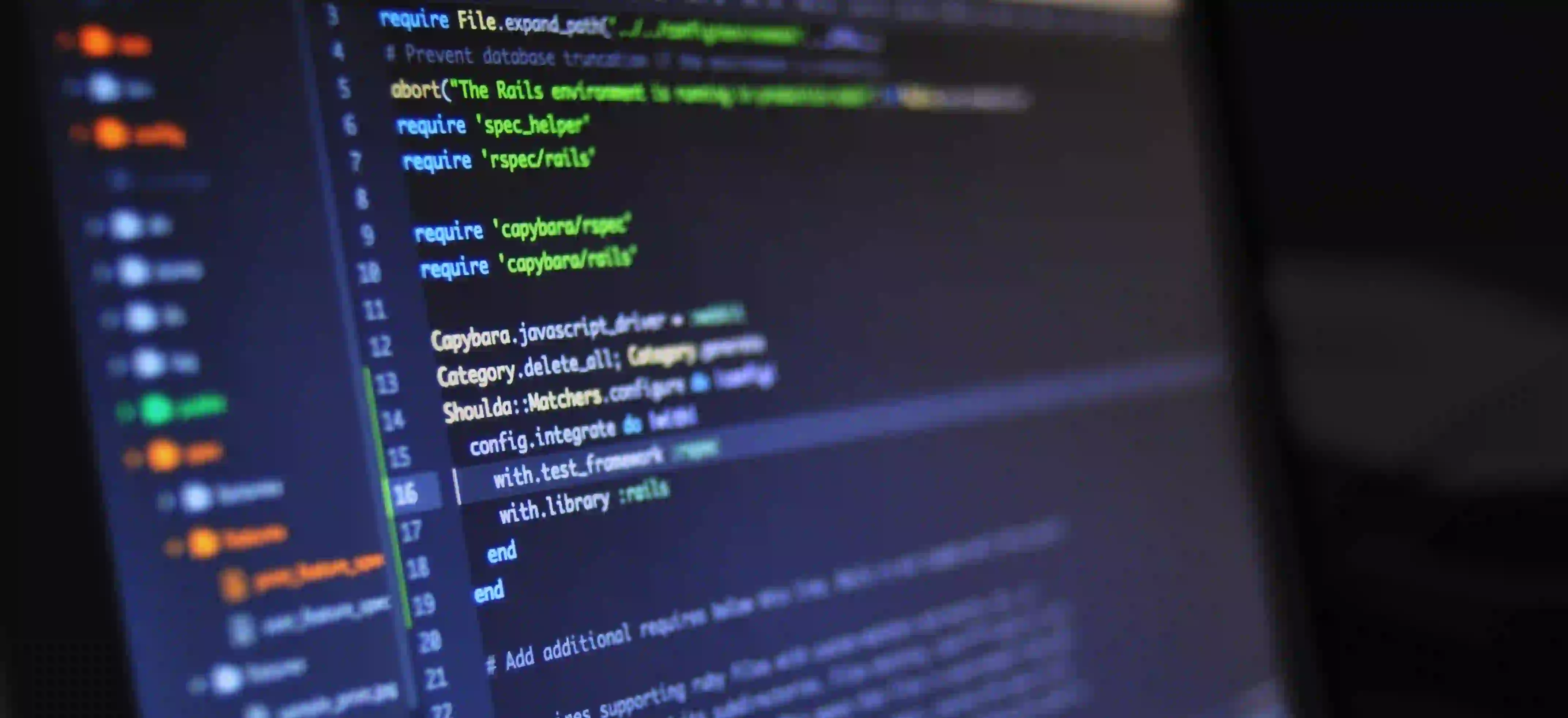
Streams vs Readers/Writers: Choosing the Right Java I/O Method
In the Java programming world, Input/Output (I/O) operations are fundamental. Whether you are reading user input, writing to a file, or processing data streams, understanding how to use Java's I/O mechanisms effectively can significantly improve your code's performance and readability. In this guide, we will dissect two primary Java I/O approaches: Streams and Readers/Writers. By the end of this article, you'll have a clearer understanding of when to use each method.
Understanding Java I/O
Java I/O is primarily divided into two categories:
- Byte Streams: These handle raw binary data and are a good choice when you need to read or write binary files (e.g., images and audio).
- Character Streams: These handle characters and are ideal for text data, using Unicode.
Stream Basics
Java's InputStream and OutputStream are the foundational classes for byte stream handling, while Reader and Writer are the counterparts for character streams.
- InputStream: Abstract class for reading byte data.
- OutputStream: Abstract class for writing byte data.
- Reader: Abstract class for reading character data.
- Writer: Abstract class for writing character data.
The table below summarizes the key classes: | Type | Class | Description | |--------------|---------------------|--------------------------------------------------| | Byte Streams | InputStream | Reads raw byte data | | | OutputStream | Writes raw byte data | | Character Streams | Reader | Reads characters (text) | | | Writer | Writes characters (text) |
Streams: The Byte-Level Approach
Advantages of Using Streams
Streams offer low-level control and are resource-efficient for binary data. They are suitable for operations like:
- Reading image files.
- Streaming audio data.
- Transmitting binary files over a network.
Here’s a simple example of reading an image file using FileInputStream
:
import java.io.FileInputStream;
import java.io.IOException;
public class ImageReader {
public static void main(String[] args) {
try (FileInputStream fis = new FileInputStream("path/to/image.jpg")) {
byte[] data = fis.readAllBytes();
// Process your byte data here...
System.out.println("Image read successfully: " + data.length + " bytes");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary on the Code
- FileInputStream: This class allows you to read raw bytes from a file, making it useful for binary files.
- readAllBytes(): This method returns all remaining bytes in the file as a byte array. Great for small to medium files when you need all the data at once.
- try-with-resources: This ensures that the
FileInputStream
is closed automatically, preventing resource leaks.
Limitations
Despite their usefulness, byte streams can be inefficient for text processing. When working with character data, you may face encoding issues. Thus, using character streams is often a better choice when working with text.
Readers/Writers: The Character-Level Approach
Advantages of Using Readers/Writers
Readers and Writers are designed for handling character data, offering support for various character encodings. They simplify reading and writing text files and are especially useful for:
- Reading and writing text files.
- Manipulating strings more efficiently.
- Supporting different character sets and encodings.
Below is an example of writing to a text file using PrintWriter
:
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
public class TextFileWriter {
public static void main(String[] args) {
try (PrintWriter writer = new PrintWriter(new FileWriter("path/to/output.txt", true))) {
writer.println("Hello, World!");
writer.println("Writing text files is easy with PrintWriter.");
System.out.println("File written successfully.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary on the Code
- PrintWriter: This is an easy-to-use class for writing formatted text. It also provides convenient methods, such as
println()
, for writing lines of text. - FileWriter: This class can be used with
PrintWriter
to specify the file. - Appending Mode: By passing
true
toFileWriter
, you enable appending to the file instead of overwriting.
Limitations
While Readers and Writers provide excellent text-handling capabilities, they may introduce overhead when processing binary data. If you're dealing with images, audio, or arbitrary binary files, stick to byte streams.
Performance Considerations
Choosing between Streams and Readers/Writers isn't just about functionality; performance is key. Here are some performance factors:
-
Buffering: When dealing with large files, consider using buffered streams such as
BufferedInputStream
orBufferedReader
to reduce I/O operations and improve efficiency. -
Character Encoding: Ensure you're aware of the character encoding. Using incorrect encoding can lead to data loss or corruption.
Use Cases
Now that we’ve reviewed the fundamentals, let's look at when to use each method:
Use Streams When:
- You need to handle binary data (images, audio).
- You’re focused on performance and lower-level I/O operations.
- You want full control over byte handling.
Use Readers/Writers When:
- Working with text files and character data is essential.
- You require ease of use and localization capabilities.
- Data encoding matters in your application.
Wrapping Up
In conclusion, both Streams and Readers/Writers have their unique advantages and use cases in Java I/O operations. Understanding the intricacies of each can help you make informed decisions that enhance your application's efficiency and functionality.
By leveraging byte streams for binary data and character streams for text data, you can optimize your Java applications for better performance and maintainability.
For further reading on Java I/O, consider checking out the official documentation:
By thoughtfully choosing between these two methodologies, you can ensure your code is not only functionally robust but also easier to maintain and modify in the long run.
Happy coding!