Solving StackOverflowError in Java Recursion Effectively
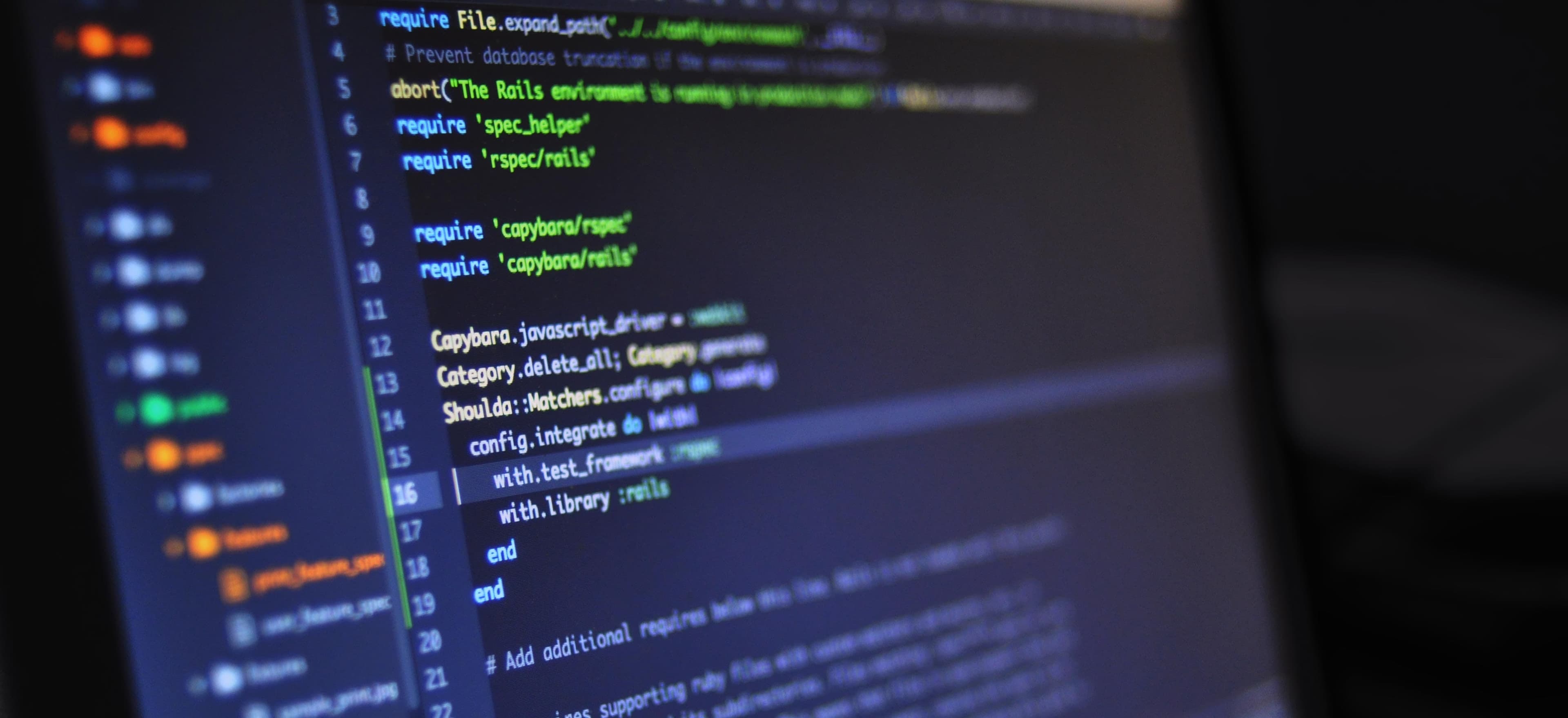
- Published on
Solving StackOverflowError in Java Recursion Effectively
Recursion is a powerful programming paradigm that allows a method to call itself to solve a problem. However, while employing recursion in Java, you may encounter a commonly daunting issue known as StackOverflowError
. This error occurs when the call stack memory allocated for a thread is exhausted, typically due to excessive recursion. In this post, we will explore the causes, consequences, and effective strategies to resolve and prevent StackOverflowError
in Java recursion.
Understanding StackOverflowError
Before diving deep into the problem, it is critical to understand what a StackOverflowError
is. It occurs when a thread has run out of call stack space, and generally, this situation arises in recursive methods where the termination condition is not met or when too many recursive calls are made.
Key Characteristics of StackOverflowError:
- Exception Type: It is an
Error
in Java, meaning it is an abnormal condition that is not usually meant to be caught. - Heap vs. Stack: Unlike heap memory, stack memory is typically much smaller, limiting how deeply recursion can go.
Basic Example of Recursion
Consider a simple recursive function that calculates the factorial of a number:
public class Factorial {
public static int factorial(int n) {
// Base case
if (n == 0) {
return 1;
}
// Recursive call
return n * factorial(n - 1);
}
public static void main(String[] args) {
System.out.println(factorial(5)); // Output: 120
}
}
In this example, the recursion halts when n
reaches 0
, preventing a StackOverflowError
. The base case is vital here, as it provides a stopping condition.
When StackOverflowError Occurs
Let’s see an example where StackOverflowError
may occur:
public class EndlessRecursion {
public static void recursiveCall() {
// No base case
recursiveCall();
}
public static void main(String[] args) {
recursiveCall(); // This will eventually cause StackOverflowError
}
}
In this code, because there is no base condition to stop the recursion, the method will continue to call itself indefinitely, leading to a StackOverflowError
.
How to Effectively Solve and Prevent StackOverflowError
-
Implement Proper Base Cases
Always ensure that your recursive methods have a clear and reachable base case to stop recursion:
public static int safeFactorial(int n) { if (n < 0) { throw new IllegalArgumentException("Negative input not allowed"); } if (n == 0) { return 1; } return n * safeFactorial(n - 1); }
In this code, we validate the input beforehand, throwing an exception for negative numbers and ensuring the base case is reached.
-
Use Iterative Solutions When Appropriate
Some recursive problems can be solved more efficiently with iterative methods. For example, calculating the Fibonacci sequence can lead to recursion issues, but an iterative solution is more memory efficient:
public static int fibonacci(int n) { if (n <= 1) { return n; } int a = 0, b = 1; for (int i = 2; i <= n; i++) { int temp = a + b; a = b; b = temp; } return b; }
This iterative approach uses a constant amount of space, avoiding
StackOverflowError
. -
Optimize Recursive Algorithms
For some problems like Fibonacci calculation, using memoization can drastically reduce call stack usage:
import java.util.HashMap; public class MemoizedFibonacci { private static HashMap<Integer, Integer> cache = new HashMap<>(); public static int fibonacci(int n) { if (n <= 1) { return n; } if (cache.containsKey(n)) { return cache.get(n); } int result = fibonacci(n - 1) + fibonacci(n - 2); cache.put(n, result); return result; } }
Here, we store calculated values in a HashMap to avoid redundant calculations, reducing the number of recursive calls.
-
Utilize Tail Recursion
Some Java implementations may optimize tail-recursive methods. A tail-recursive function’s recursive call is at the end of the function, allowing compilers to optimize stack memory usage. Java does not optimize tail-recursive calls natively but writing them can still help in languages that do.
Here’s an illustration of how you might write such a function:
public class TailRecursionExample { public static int tailRecursiveHelper(int n, int accumulator) { if (n == 0) { return accumulator; } return tailRecursiveHelper(n - 1, n * accumulator); } public static int factorial(int n) { return tailRecursiveHelper(n, 1); } }
This approach keeps track of the intermediate results, reducing the depth of the recursion.
-
Increase Stack Size (Last Resort)
If the recursion depth is inherently deep due to the problem's nature, you may consider increasing the Java Virtual Machine (JVM) stack size, although this is usually a last resort. For example, you can use the
-Xss
parameter when running your Java application:java -Xss2m YourClassName
Here,
-Xss2m
sets the stack size to 2 megabytes. This is useful in environments where deep recursion is unavoidable, but it's better to find a solution that doesn't necessitate changing defaults.
Closing the Chapter
In conclusion, StackOverflowError
can thwart developers working with recursive algorithms in Java. By keeping the four strategies discussed in mind—implementing proper base cases, considering iterative solutions, optimizing with memoization, and practicing tail recursion—you can effectively reach your goal without overflowing the stack.
Remember that recursion is a powerful tool in your programming arsenal when used correctly. Balancing between recursion and other programming techniques can pave the way for optimized and safer code.
For further extensive reading on recursion in Java, you can refer to Oracle's official documentation.
Additional Resources
- Understanding Recursion
- Java Memory Management
By following these principles, you can ensure that your recursive implementations remain efficient and manageable, avoiding the dreaded StackOverflowError
. Happy coding!
Checkout our other articles