Understanding 'Happens-Before' to Avoid Java Memory Bugs
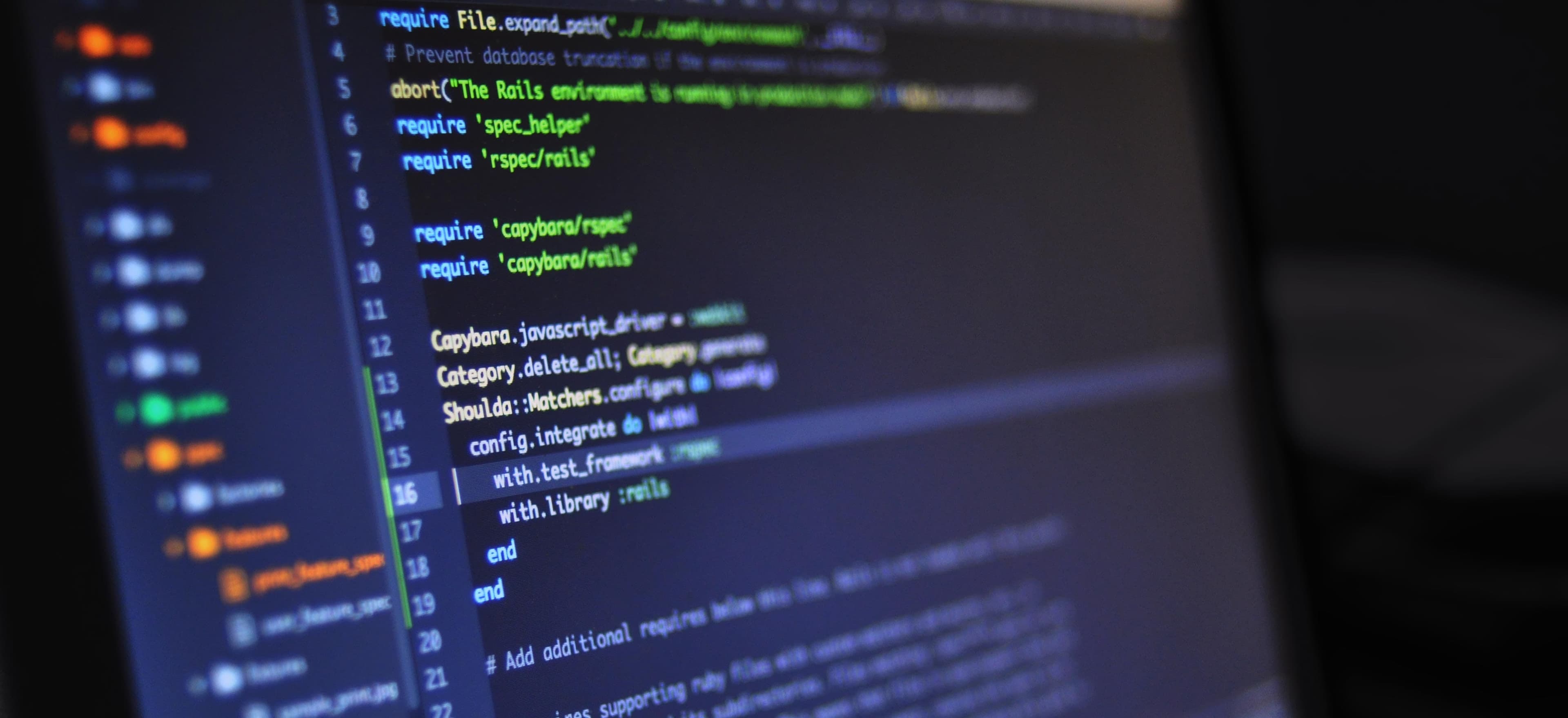
- Published on
Understanding 'Happens-Before' to Avoid Java Memory Bugs
In the world of multithreading, ensuring that your Java application runs smoothly and reliably can be a daunting task. One of the fundamental concepts that developers must comprehend is the "happens-before" relationship. This relationship is crucial for reasoning about thread safety and eliminating memory bugs in concurrent applications. In this post, we'll delve into the meaning of "happens-before," explore how it relates to Java's memory model, and provide code examples to illustrate its significance.
What is Happens-Before?
The happens-before relationship is a formal rule that helps define the order of operations in a concurrent environment. It describes how memory writes made by one thread are visible to another thread. Understanding this relationship helps prevent issues such as stale data and race conditions, which can lead to unpredictable behavior in your programs.
In Java, the happens-before relationship is primarily defined by the Java Memory Model (JMM). According to the JMM, if one action happens-before another, then the first action is visible and ordered before the second action.
Key Principles of Happens-Before
-
Program Order Rule: Each action in a single thread happens-before every action that comes after it in that same thread.
-
Monitor Lock Rule: An unlock on a monitor happens-before each subsequent lock on that same monitor. This is key for synchronized blocks.
-
Volatile Variable Rule: A write to a volatile variable happens-before every subsequent read of that variable. Volatile variables provide visibility guarantees across threads.
-
Thread Start Rule: A call to Thread.start() on a thread happens-before any actions in the started thread.
-
Thread Join Rule: All actions in a thread happen-before any thread that successfully returns from a Thread.join() on that thread.
Understanding these rules can significantly reduce the chance of introducing bugs into your Java applications.
Why Should You Care About Happens-Before?
Failing to respect the happens-before relationship can lead to memory visibility issues, where a thread does not see updates made by another thread. This can manifest as inconsistent data states, resulting in bugs that are notoriously hard to track down.
Example of a Memory Bug
Let's illustrate a classic situation where not understanding happens-before can lead to problems.
public class SharedResource {
private int counter = 0;
public void increment() {
counter++;
}
public int getCounter() {
return counter;
}
}
In this example, multiple threads might call the increment()
method simultaneously. The method seems simple, but if multiple threads access counter
at the same time, you could end up with a race condition.
Issues with the Above Code
Consider what could happen if we have two threads incrementing the counter at the same time without proper synchronization:
- Thread A reads the current value of
counter
. - Thread B reads the same value.
- Both threads increment the value independently.
- Finally, both threads set
counter
back, resulting in only one increment instead of two.
To fix this, we can introduce synchronization.
Using Synchronization
We can employ synchronization to ensure that only one thread modifies the counter
at a time.
public class SharedResource {
private int counter = 0;
public synchronized void increment() {
counter++;
}
public synchronized int getCounter() {
return counter;
}
}
Here, the synchronized
keyword is essential. According to the monitor lock rule, the increment()
method's unlock happens-before any subsequent call to the increment()
or getCounter()
methods. This ensures that the changes made to the counter by one thread are visible to the other threads.
Volatile Variables
In cases where visibility is more crucial than atomicity, we can use volatile
variables.
public class VolatileExample {
private volatile boolean active = true;
public void deactivate() {
active = false; // 1
}
public void monitor() {
while (active) { // 2
// perform some operation
}
}
}
In this example, the volatile keyword guarantees that if one thread changes the active
variable to false (line 1), any thread reading active
(line 2) will see that change. Thus, we prevent potential visibility issues that could arise from caching stale values in registers or local caches.
The Power of Atomic Variables
Java also offers atomic classes in the java.util.concurrent.atomic
package, which provide thread-safe operations without using explicit synchronization.
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicExample {
private AtomicInteger counter = new AtomicInteger(0);
public void increment() {
counter.incrementAndGet(); // Thread-safe increment
}
public int getCounter() {
return counter.get(); // Thread-safe access
}
}
With AtomicInteger
, you get built-in atomic operations. This means that the increment operation is not only thread-safe but also ensures that the increment is visible to other threads immediately.
How to Avoid Memory Bugs
- Use
synchronized
for critical sections to ensure mutual exclusion. - Use
volatile
for visibility when you need cheap reads and writes without the overhead of locking. - Leverage atomic classes for compound actions that require atomicity without locking.
- Always adhere to the happens-before rules to understand and predict memory visibility between threads.
Closing Remarks
Understanding the happens-before relationship is imperative in writing safe and efficient multithreaded applications in Java. Mismanaging shared data can lead to memory bugs that are difficult to debug and result in unpredictable application behavior. By employing synchronization, volatile variables, and atomic operations, you can significantly diminish the risks of data inconsistencies.
Java provides a robust framework for managing concurrency, but it requires a clear understanding of the underlying concepts. Familiarizing yourself with happens-before not only helps you write better Java code but also bolsters your ability to maintain and scale applications in a multithreaded environment.
For further reading on concurrency in Java, check out the Java Concurrency in Practice book or visit the java.util.concurrent documentation.
Remember, concurrency is not just about doing things simultaneously—it's about doing things correctly!
Checkout our other articles