Mastering Memory Management: Soft vs Weak References in Java
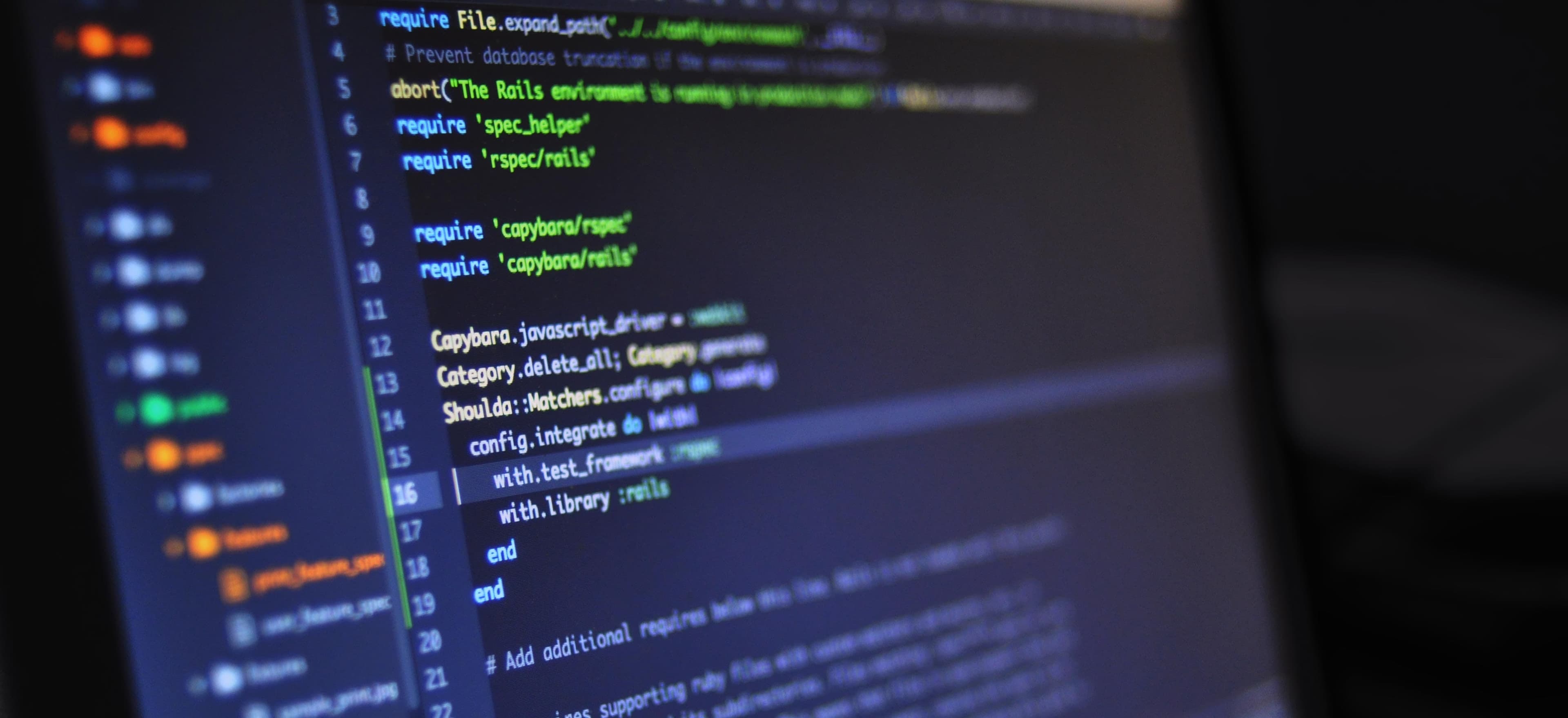
- Published on
Mastering Memory Management: Soft vs Weak References in Java
Managing memory is a crucial aspect of Java programming. With Java's automatic garbage collection, developers often take a backseat regarding memory management. However, understanding how different types of references work can give you an edge in building efficient applications. In this blog post, we will delve into soft and weak references, examining their use cases, benefits, and practical implementations.
Understanding References in Java
Before diving into soft and weak references, it is essential to understand the basic memory references in Java:
-
Strong References: The most common type. If there is a strong reference to an object, the garbage collector won't reclaim that object's memory.
Object obj = new Object(); // Strong reference to a new object.
-
Soft References: These allow the garbage collector to reclaim memory more aggressively while keeping objects available for as long as possible.
-
Weak References: These ensure that the referenced object can be collected more easily and is cleared at the next garbage collection cycle if there are no strong references to it.
-
Phantom References: This type provides a way to determine when an object has been finalized and is no longer reachable.
What Are Soft References?
Soft references are used in cases where you want to allow the garbage collector to free up memory if the system is running low on memory, but still maintain a cache-like behavior. They are particularly useful in the implementation of caches, where you want to cache data but don't want it to prevent the application from shutting down in low memory scenarios.
Soft Reference Implementation
Let’s look at an example of how to use soft references in Java to create a basic cache system.
import java.lang.ref.SoftReference;
import java.util.HashMap;
import java.util.Map;
public class SoftReferenceCache {
private final Map<String, SoftReference<Object>> cache = new HashMap<>();
public void put(String key, Object value) {
cache.put(key, new SoftReference<>(value));
}
public Object get(String key) {
SoftReference<Object> softRef = cache.get(key);
return softRef != null ? softRef.get() : null; // Returns null if collected
}
}
Commentary on the Code
-
SoftReference: The
SoftReference
class in this example wraps an object to allow it to be garbage collected when memory is needed but keeps it available as long as there is memory. -
Cache Logic: When putting an object in the cache, you create a new soft reference. When retrieving, you check if the object was collected. If not, it gracefully returns the value.
Using soft references in a caching mechanism helps optimize memory usage, allowing your application to recover gracefully from out-of-memory situations rather than crashing unexpectedly.
What Are Weak References?
Weak references are even more aggressive than soft references. When the garbage collector runs, it will reclaim any memory for an object that is only weakly reachable. This means that if there are no strong references to the object, it will be collected regardless of whether there are weak references pointing to it.
Weak Reference Implementation
Here's how you can implement a weak reference in a similar cache-like system.
import java.lang.ref.WeakReference;
import java.util.HashMap;
import java.util.Map;
public class WeakReferenceCache {
private final Map<String, WeakReference<Object>> cache = new HashMap<>();
public void put(String key, Object value) {
cache.put(key, new WeakReference<>(value));
}
public Object get(String key) {
WeakReference<Object> weakRef = cache.get(key);
return weakRef != null ? weakRef.get() : null; // Returns null if collected
}
}
Commentary on the Code
-
WeakReference: Similar to the soft reference cache, we use
WeakReference
to wrap objects. If the memory is needed, the garbage collector can collect the object whenever it wants. -
Immediate Collection: An object stored in a weak reference can be cleaned up quickly, clearing away the space it occupied, making this approach particularly suitable for managing large objects where you want minimal memory overhead.
Comparing Soft and Weak References
| Feature | Soft References | Weak References | |---------------------|------------------------|------------------------| | Collection Timing | Collected before OutOfMemoryError | Collected at next GC cycle | | Use Case | Caches and memory-sensitive data | Short-lived associations | | Memory Pressure | Collected under memory pressure | Collected immediately when not strongly reachable |
When to Use Which?
-
Soft References: Use them for caching data that you want to keep around for quick access but can be reloaded if necessary. For instance, image caches, session data, etc.
-
Weak References: They are more appropriate for implementing listeners or associations that should not prevent an object from being garbage collected. For example, holding a reference to a large object that you might not need if it isn't being actively used.
Real-World Considerations
When developing applications in Java, understanding how references interact with the garbage collector can significantly enhance performance and memory management. Caching strategies that use soft and weak references allow your application to adapt to varying memory conditions seamlessly. Oracle's Java Documentation is an excellent resource for understanding how Java handles references.
The Last Word
Mastering memory management with soft and weak references can make a substantial difference in performance and reliability in Java applications. By using these references effectively, developers can create efficient caches that improve resource utilization while preventing out-of-memory errors.
For further reading on memory management in Java, consider exploring topics like the Java Memory Model or profiling memory usage with tools like VisualVM. Understanding these concepts will pave the way for mastering advanced Java performance optimization techniques.
By applying these techniques, you will not only improve the efficiency of your applications but will also gain a deeper understanding of Java’s memory management semantics. Happy coding!
Checkout our other articles