Unlocking the Hidden Challenges of Java Dynamic Proxies
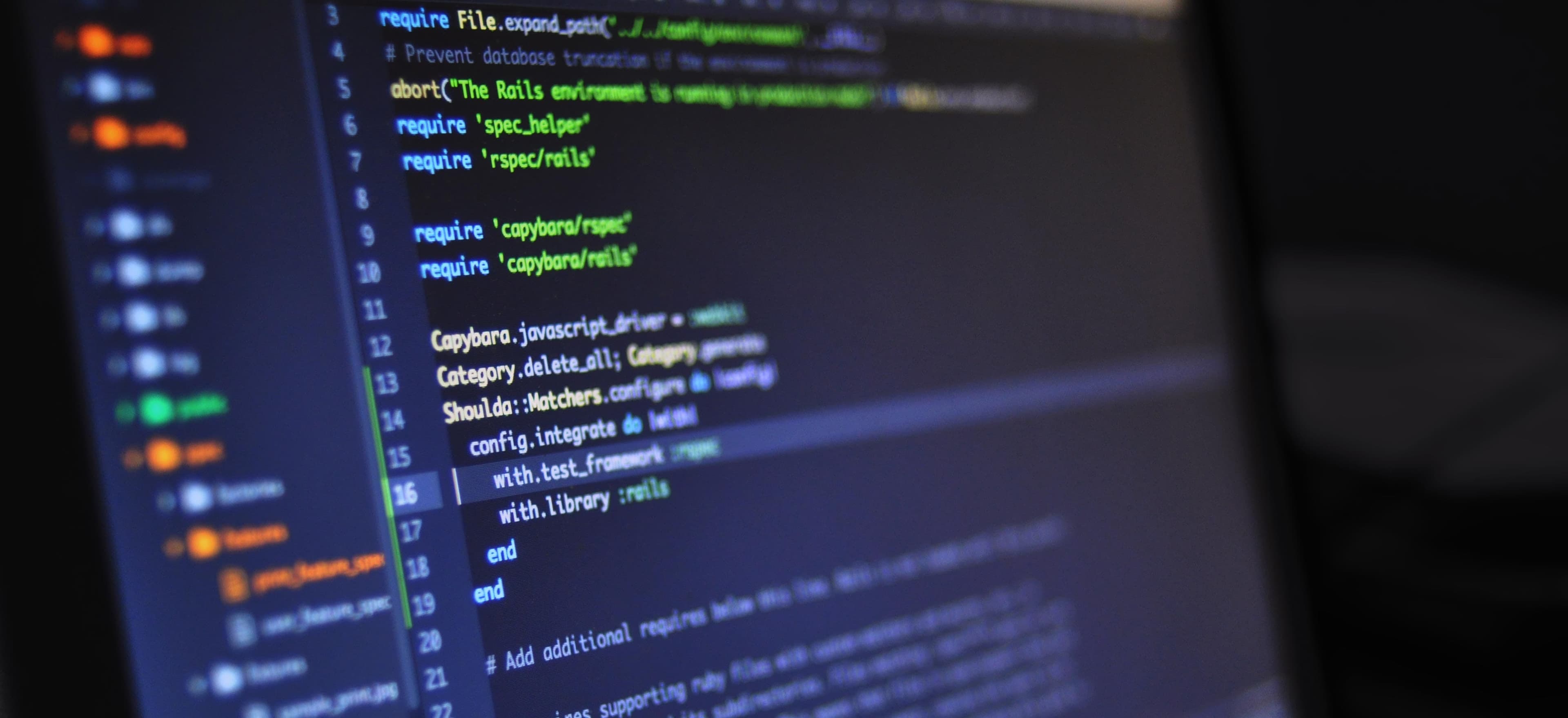
- Published on
Unlocking the Hidden Challenges of Java Dynamic Proxies
Java, a versatile and widely-used programming language, is known for its rich set of features. One such feature is the concept of dynamic proxies. Dynamic proxies offer a powerful way to create proxy instances at runtime, enabling developers to implement cross-cutting concerns, such as logging and transaction management, without altering the logic of the original object. However, with great power comes great responsibility—and challenges.
In this blog post, we will delve into the complexities and hidden challenges of Java dynamic proxies. We’ll explore how they work, when to use them, and the pitfalls you may encounter along the way. This guide aims to clarify the nuances of this powerful feature and provide you with practical insights to navigate its challenges effectively.
What Are Dynamic Proxies?
Dynamic proxies in Java allow you to create proxy instances for interfaces at runtime. The Java Reflection API supports this feature through the java.lang.reflect.Proxy
class, which can be utilized to create proxy instances of any interface type. Proxies are particularly useful in scenarios including:
- Method interception (e.g., logging, metrics)
- AOP (Aspect-Oriented Programming)
- Implementing design patterns like the Proxy Pattern
Creating a Simple Dynamic Proxy
Here's a basic example demonstrating how to create a dynamic proxy:
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
// Step 1: Define an interface
interface HelloService {
void sayHello(String name);
}
// Step 2: Implement the InvocationHandler interface
class HelloServiceHandler implements InvocationHandler {
private final HelloService helloService;
public HelloServiceHandler(HelloService helloService) {
this.helloService = helloService;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
System.out.println("Before Method Invocation");
Object result = method.invoke(helloService, args); // Actual method invocation
System.out.println("After Method Invocation");
return result;
}
}
// Step 3: Create the proxy instance
public class DynamicProxyExample {
public static void main(String[] args) {
HelloService helloService = (name) -> System.out.println("Hello, " + name);
HelloService proxyInstance = (HelloService) Proxy.newProxyInstance(
HelloService.class.getClassLoader(),
new Class<?>[]{HelloService.class},
new HelloServiceHandler(helloService));
proxyInstance.sayHello("Alice");
}
}
Explanation of the Code
- Step 1: We define an interface
HelloService
, which declares a methodsayHello
. - Step 2: We create
HelloServiceHandler
, an implementation of theInvocationHandler
interface. This serves as our interceptor that can add behavior before and after the method invocation. - Step 3: We invoke
Proxy.newProxyInstance()
to create a new instance of the proxy. Here, we pass the class loader, the interface we want to create a proxy for, and the handler that will manage the method invocations.
Why Use Dynamic Proxies?
Dynamic proxies help you separate concerns in your application. They enable cleaner code by allowing you to abstract cross-cutting functionalities, such as logging and error handling, into a single point without tangling them within business logic. This modular approach enhances maintainability and flexibility.
Hidden Challenges of Using Dynamic Proxies
While dynamic proxies are powerful, they are not without their challenges. Below are some of the key hurdles you may face.
1. Type Safety Issues
Dynamic proxies are inherently less type-safe than static proxies. Since they work with Object
, you may end up with ClassCastException
at runtime if the expected type doesn’t match.
Solution: Always validate the types passed to your proxy methods and ensure consistent usage of generics wherever possible.
2. Performance Overhead
Dynamic proxies incur performance overhead due to reflection. Every method invocation requires a reflection call, which is slower than regular method calls. This can severely impact performance in high-frequency method calls.
Solution: Use dynamic proxies judiciously and only for operations that require them. Consider alternatives, such as static proxies or more performant frameworks like Byte Buddy.
3. Limited to Interfaces
Dynamic proxies can only be used to create proxies for interfaces. If you want to proxy a concrete class, this limitation can become a significant barrier.
Solution: When working with concrete classes, consider using libraries that allow bytecode manipulation, such as CGLIB or AspectJ.
4. Debugging Difficulties
Debugging dynamic proxies can be challenging. Since behavior is injected at runtime, tracking flow and understanding what's happening under the hood often requires a deeper understanding of the Java Reflection API.
Solution: Use logging and debugging tools effectively. Learning to read stack traces and method invocations can significantly ease debugging.
5. Lack of Support for Non-Interface Types
Dynamic proxies only work with interfaces; if your design heavily relies on concrete classes, then you cannot use dynamic proxies without restructuring your code.
Solution: Emphasize the use of interfaces in your application design from the initial stages. Adopting an interface-driven approach can mitigate this challenge.
Best Practices for Using Dynamic Proxies
To leverage the power of dynamic proxies while avoiding their pitfalls, consider the following best practices:
-
Define Clear Interfaces: Start by defining clear interfaces for your services. This will make it easier to create proxy instances and manage method invocations.
-
Limit the Scope: Use dynamic proxies only where necessary. If the overhead outweighs the benefits, consider alternative solutions.
-
Monolithic vs. Microservices: In a microservices architecture, dynamic proxies are often unnecessary and can introduce additional complexity. Evaluate architecture before implementation.
-
Unit Testing: Write unit tests for components that utilize dynamic proxies to ensure functionality remains intact and to simplify debugging.
-
Use AOP Frameworks: If using dynamic proxies for cross-cutting concerns, consider more robust AOP frameworks like Spring AOP, which simplify proxy management.
Final Thoughts
Despite the challenges associated with Java dynamic proxies, they remain an essential tool in the developer's arsenal. By understanding their complexities and adhering to best practices, you can unlock their full potential while avoiding common pitfalls.
Ultimately, it’s crucial to assess your project's requirements before diving into dynamic proxies. Always keep performance, maintainability, and code clarity at the forefront of your decision-making process.
For further reading and a deeper understanding of proxies and their implementation, consider checking out:
By exploring these resources, you can continue your journey in mastering Java and dynamic proxies effectively, leading to cleaner, more maintainable code.
Checkout our other articles