Troubleshooting SSL Handshake Failures in Java Applications
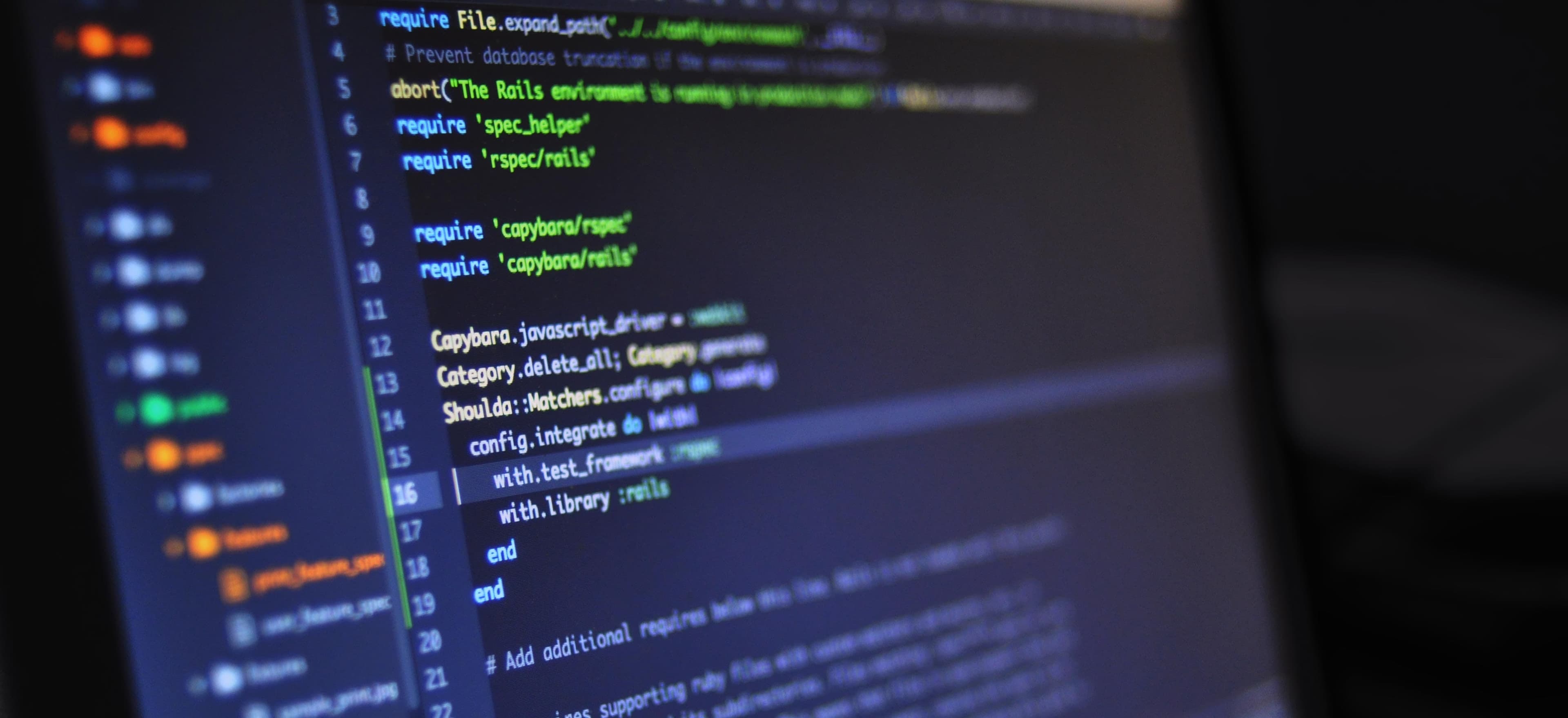
- Published on
Troubleshooting SSL Handshake Failures in Java Applications
When developing secure Java applications, utilizing SSL/TLS is essential to protect sensitive data during transmission. However, SSL handshake failures often occur, disrupting the secure communication channel. This blog post delves into the causes and troubleshooting methods for SSL handshake failures in Java applications.
Understanding the SSL Handshake
The SSL handshake is the process that initiates a secure communication session between a client and a server. It involves several steps to agree upon encryption protocols, authenticate the server (and optionally the client), and establish keys for the session. Any malfunction in this process can result in handshake failures.
Key functionalities during the handshake include:
- Protocol Version Negotiation: Versions of SSL/TLS must be compatible between client and server.
- Cipher Suite Agreement: The client and server must find a common cipher suite.
- Certificate Exchange and Validation: The server presents its certificate to the client for authentication.
To better visualize this process, refer to this comprehensive guide.
Common Causes of SSL Handshake Failures
1. Unsupported Protocol Version
Java applications may be configured to use a specific TLS version. If the server only supports a newer version, the handshake will fail.
Quick Fix:
Check the server's supported protocols and ensure your application is compatible.
2. Misconfigured Certificates
Certificate misconfigurations, such as expired certificates or incorrect certificate chains, can prevent a successful handshake.
Quick Fix:
Verify the server's SSL certificate:
- Check if the certificate is expired.
- Ensure the proper certificate chain is presented by the server.
3. Cipher Suite Mismatch
If the client and server cannot agree on a common cipher suite, an SSL handshake failure will occur.
Quick Fix:
Use tools like SSL Labs to scan your server’s supported cipher suites and ensure your application supports at least one of them.
4. Intermediary Network Issues
Firewalls, proxies, and network settings can interfere with SSL traffic, leading to handshake failures.
Quick Fix:
Inspect network configurations and ensure that no firewall or proxy strip SSL/TLS packets.
Troubleshooting Steps
Step 1: Enable SSL Debugging
Java provides built-in SSL debugging capabilities that can be activated using a system property. You can enable SSL debugging by including the following argument when starting your Java application:
-Djavax.net.debug=ssl
This flag produces verbose output, providing insights into the handshake process. Here’s how to interpret the logs:
ClientHello
: The client informs the server of supported protocols and cipher suites.ServerHello
: Shows the server’s selections which agreed with the client's preferences.
For example:
*** ClientHello, TLSv1.2
*** ServerHello, TLSv1.2
Take note of any handshake failures indicated in the logs.
Step 2: Use SSL Tools
Tools such as OpenSSL can help diagnose handshake issues by allowing you to manually test the connection to the server. Here’s how to test a server with OpenSSL:
openssl s_client -connect example.com:443 -tls1_2
This command tests the connection to the specified server using TLS 1.2. Analyze the output for any certificate validation errors or unsupported protocol versions.
Step 3: Update Java Runtime
Using an outdated Java Runtime Environment (JRE) can lead to incompatibility with newer SSL/TLS protocols. Ensure your Java version is up to date. Use the command:
java -version
You can download the latest version of Java from Oracle’s website.
Step 4: Validate Server Certificate
If the server's SSL certificate is self-signed or not trusted by the client application, the handshake will fail. You can import the server’s certificate into your Java Keystore:
keytool -import -alias myserver -keystore cacerts -file server-cert.pem
Make sure you provide the appropriate path to your Java cacerts file, which is typically found within the Java installation directory.
Example Code Snippet
Below is an example of how to create an HTTPS connection in Java while incorporating error handling to catch SSL exceptions:
import javax.net.ssl.HttpsURLConnection;
import java.net.URL;
public class SSLDemo {
public static void main(String[] args) {
try {
URL url = new URL("https://example.com");
HttpsURLConnection connection = (HttpsURLConnection) url.openConnection();
connection.setRequestMethod("GET");
// Get response code
int responseCode = connection.getResponseCode();
System.out.println("Response Code: " + responseCode);
} catch (Exception e) {
// Handle SSL Exceptions specifically
if (e.getMessage().contains("SSLHandshakeException")) {
System.err.println("SSL handshake failed: " + e.getMessage());
} else {
System.err.println("An error occurred: " + e.getMessage());
}
}
}
}
Why Error Handling Matters
In the above code, we specifically catch SSLHandshakeException
, allowing us to inform users directly about SSL issues, which aids in quicker diagnosis and resolution.
Additional Resources
For further reading on SSL/TLS and Java applications, check out the following resources:
- Understanding SSL and TLS
- Java Secure Socket Extension (JSSE) Reference Guide
- Testing SSL/TLS Configurations
The Last Word
SSL handshake failures can stem from various issues including protocol mismatches, certificate problems, cipher suite mismatches, and network settings. By following systematic troubleshooting steps—like enabling debug logging and checking certificate validity—developers can diagnose and resolve these problems efficiently. Always remember to keep your Java environment updated to avoid potential incompatibilities, as security technologies are continually evolving.
Secure your communications effectively!