Why Immutable Objects are Crucial in Java's Shared State
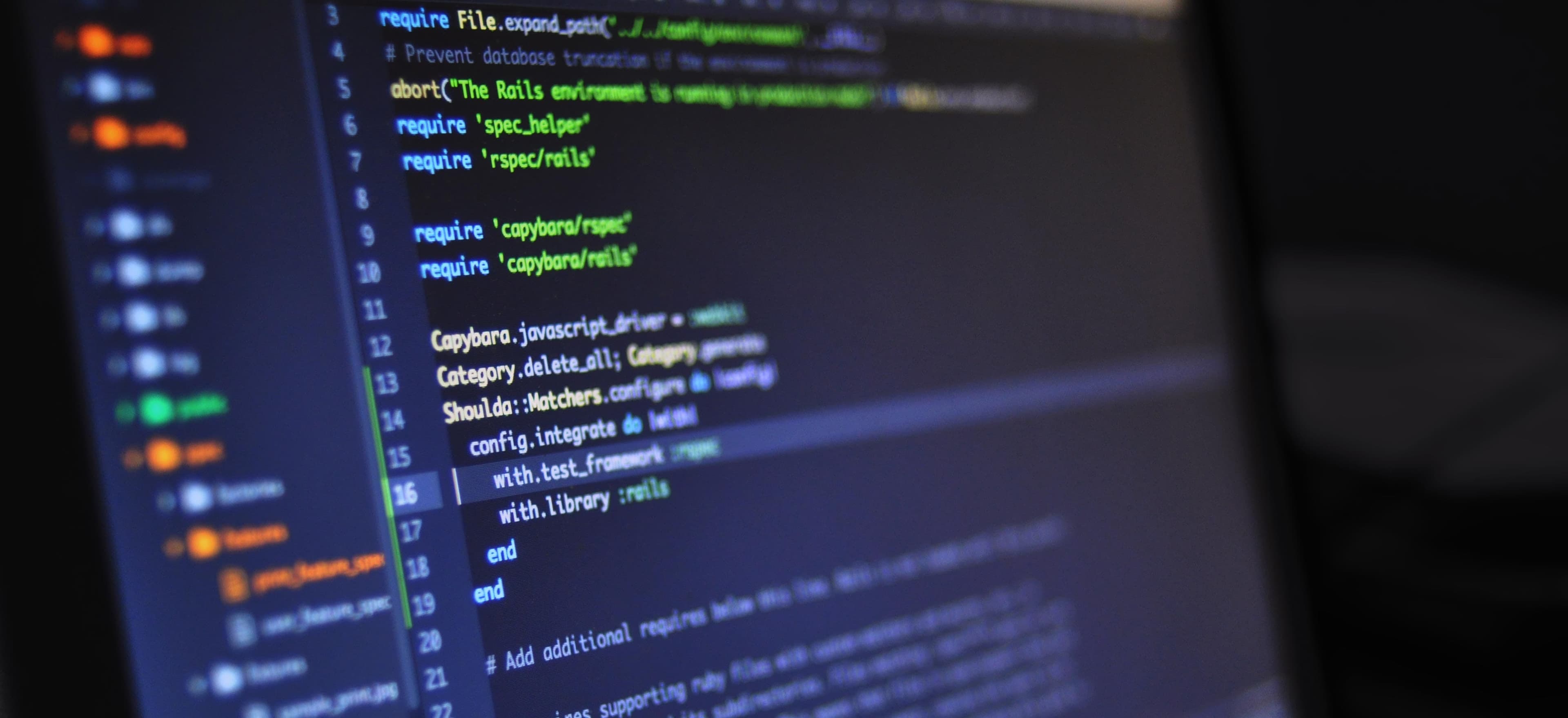
- Published on
Why Immutable Objects are Crucial in Java's Shared State
In the world of Java programming, the design and architecture of systems can significantly impact the reliability and maintainability of code. One such design principle that has gained traction over the years is the use of immutable objects. In this blog post, we will explore what immutable objects are, why they are crucial in Java's shared state scenarios, and how to effectively implement them.
Understanding Immutable Objects
Immutable objects are instances of a class whose state cannot be modified after they’re created. This means that once an immutable object is constructed, it remains unchanged throughout its lifetime. Common examples of immutable classes in Java include String
, Integer
, and LocalDate
.
Benefits of Immutability
-
Thread Safety: Immutable objects inherently provide thread safety. This is because there’s no possibility of an object's state changing while multiple threads access it.
-
Easier to Understand: Since the state of an immutable object does not change, reasoning about code becomes simpler. You can be confident that the data remains constant as long as the object exists.
-
Cache Friendly: Immutable objects can be cached and reused without the risk of unexpected changes. This leads to improved performance due to reduced memory overhead.
-
Preventing Side Effects: Functions that consume immutable objects cannot cause side effects, reducing debugging complexity.
Why are Immutable Objects Important in Shared State?
In Java, especially in concurrent programming, managing shared state is critical. When multiple threads operate on a shared resource, the potential for conflicts and race conditions rises dramatically. Here, immutable objects shine as a solution for several reasons:
1. Elimination of Race Conditions
When multiple threads access a mutable object concurrently, race conditions may occur, leading to unpredictable outcomes. With immutable objects, since the objects cannot be modified, they eliminate the possibility of race conditions entirely. Here is a simple code example illustrating this point:
public final class ImmutablePoint {
private final int x;
private final int y;
public ImmutablePoint(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
// No setters, maintaining the immutability of this class
}
In this example, the ImmutablePoint
class represents a point with x
and y
coordinates. The absence of setter methods ensures that the state of an object of this class cannot change after instantiation.
2. Simplified Concurrent Programming
When using immutable objects, the requirement for synchronization diminishes. In scenarios where multiple threads read from a shared resource, using immutable objects simplifies your code. Here's a basic illustration:
class SharedResource {
private final ImmutablePoint point;
public SharedResource(ImmutablePoint point) {
this.point = point;
}
public ImmutablePoint getPoint() {
return point;
}
}
In this SharedResource
class, multiple threads can safely access getPoint()
without worrying about synchronization since point
is immutable. You can learn more about concurrency in Java from Java Concurrency in Practice by Brian Goetz, which delves deeper into these concepts.
3. Consistency of State
Immutable objects maintain a consistent state. Once created, their state remains the same, ensuring any consumer of these objects is dealing with a stable reference. This is particularly advantageous in environments where the consistency of state is crucial, such as within a distributed system or application relying on caching mechanisms.
4. Functional Programming Paradigm
The rise of functional programming in Java (with the introduction of lambda expressions and the Stream API in Java 8) aligns well with the concept of immutability. Functions that accept immutable data can behave as pure functions, producing the same output for the same input without side effects:
public class PointService {
public ImmutablePoint movePoint(ImmutablePoint point, int deltaX, int deltaY) {
return new ImmutablePoint(point.getX() + deltaX, point.getY() + deltaY);
}
}
Here, the movePoint
method accepts an immutable point and returns a new point modified based on the parameters, preserving the original object's state.
Implementing Immutable Objects in Java
Creating an immutable object requires a specific approach. Let's delve into best practices for designing immutable classes:
-
Declare the class as final to prevent subclassing.
-
Make all fields private and final. This ensures that the values assigned to fields cannot change after construction.
-
Do not provide setter methods. By eliminating setters, you prevent any changes to the object's state.
-
Initialize all fields via a constructor. Ensure that all data is supplied at construction time.
-
Return copies of mutable objects. If your class has a mutable object (like an array or a
Date
), return a copy instead of returning the original reference.
Example of Proper Implementation
Here’s how you might implement an immutable class with these principles:
import java.util.Date;
public final class ImmutableOrder {
private final String orderId;
private final Date orderDate;
public ImmutableOrder(String orderId, Date orderDate) {
this.orderId = orderId;
// We create a new Date instance to ensure immutability.
this.orderDate = new Date(orderDate.getTime());
}
public String getOrderId() {
return orderId;
}
public Date getOrderDate() {
return new Date(orderDate.getTime());
}
}
The Bottom Line
In conclusion, immutable objects play a pivotal role in Java programming, particularly when shared state is involved. They mitigate the complexities associated with concurrency, enhance performance, and promote cleaner code.
By adopting an immutable design pattern in your systems, you can safeguard against a variety of common issues encountered in mutable state management. The next time you are faced with designing classes in Java, consider leveraging the power of immutability.
This approach not only results in more readable and maintainable code but also significantly improves application reliability and robustness.
For further reading on immutability in Java, you may check out resources like Effective Java by Joshua Bloch, which provides valuable insights into best practices in Java programming.
By understanding and implementing immutability effectively, you can become a more proficient Java developer, enhancing both your coding capabilities and the quality of the software you create.