Common Pitfalls of Static Blocks in Java Initialization
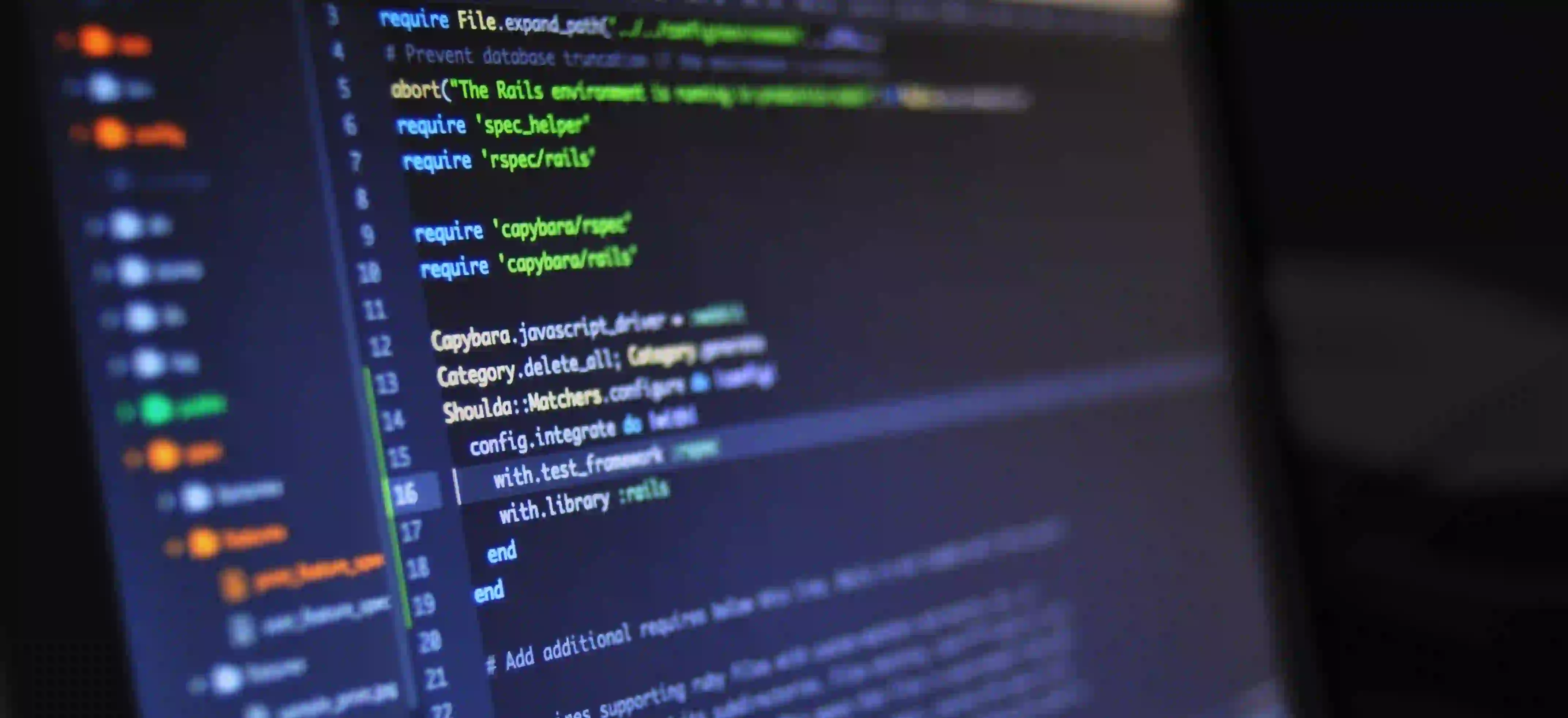
Common Pitfalls of Static Blocks in Java Initialization
Java is a powerful and versatile programming language, widely used in various applications from server-side development to mobile applications. One aspect of Java that often comes up in discussions is static blocks. These blocks allow developers to initialize static variables or perform tasks when the class is loaded. However, though they may seem straightforward, using static blocks can introduce several pitfalls if not handled correctly.
In this blog post, we will explore the common pitfalls associated with static blocks in Java initialization. We will use clear code examples and elaborate on the 'why' behind these pitfalls to enhance your understanding. Let's dive into the nuances of this feature and sharpen your Java skills.
Understanding Static Blocks
Before we jump into the pitfalls, let's define what a static block is. A static block is a block of code that runs at the time of class loading before the main method is called. It is typically used to perform initializations or execute any static initialization that needs to happen once when the class is loaded.
Here's a quick example:
public class MyClass {
static int staticVariable;
static {
staticVariable = 5; // Initializing static variable
System.out.println("Static block executed");
}
public static void main(String[] args) {
System.out.println("Static Variable: " + staticVariable);
}
}
In this code, the static block initializes staticVariable
to 5 when the class MyClass
is loaded, before executing the main
method. You'll see the output:
Static block executed
Static Variable: 5
Common Pitfalls
1. Overusing Static Blocks
One key pitfall is the overuse of static blocks for initialization when simpler means exist. While static blocks can perform complex initializations, using them unnecessarily can clutter your class and reduce readability.
Why to Avoid: Readability is crucial in software development. Overcomplicating logic with static blocks can confuse other developers who read your code.
Instead: Use regular static initializations when possible:
public class MyClass {
static int staticVariable = 5; // Cleaner initialization
public static void main(String[] args) {
System.out.println("Static Variable: " + staticVariable);
}
}
2. Uncaught Exceptions
Static blocks can throw exceptions, but this is often overlooked. If an exception is thrown during the execution of a static block, the class will not load, and an error will be raised.
public class MyClass {
static {
System.out.println("Static block executed");
int x = 1 / 0; // This throws ArithmeticException
}
public static void main(String[] args) {
System.out.println("This will not run due to exception.");
}
}
Why to Avoid: If a class fails to load, it can have cascading effects on your application, potentially crashing or causing unforeseen issues downstream.
Best Practice: Handle exceptions properly:
public class MyClass {
static {
try {
int x = 1 / 0;
} catch (ArithmeticException e) {
System.err.println("Exception in static block: " + e.getMessage());
}
}
public static void main(String[] args) {
System.out.println("Static block handling exception finished.");
}
}
3. Unpredictable Execution Order
Another common issue is related to the execution order of static blocks in cases of inheritance. Static blocks in superclasses are executed first, followed by static blocks in subclasses.
class SuperClass {
static {
System.out.println("SuperClass static block");
}
}
class SubClass extends SuperClass {
static {
System.out.println("SubClass static block");
}
}
public class Test {
public static void main(String[] args) {
new SubClass(); // Triggers class loading order.
}
}
Output:
SuperClass static block
SubClass static block
Why to Avoid: Not being aware of this execution order can lead to unexpected behavior and bugs if the subclass relies on uninitialized static variables from the superclass.
4. State Dependence
Static blocks should not rely on the state of instance variables. Since static context does not have access to instance variables, it can lead to algorithms that assume state where it doesn't exist.
public class MyClass {
static int staticVar;
int instanceVar;
static {
// System.out.println(instanceVar); // Uncommenting would cause an error
}
}
This will compile with an error.
Why to Avoid: Confusion can arise as developers may assume static blocks have access to all class fields. Remember that static blocks have a different lifetime and context than instance fields.
5. Performance Issues
While not a direct pitfall, be aware that excessive use of complex logic within static blocks can lead to performance issues. Static blocks are executed only once at class loading time, however, they can still introduce significant overhead if poorly designed.
Why to Avoid: It can slow down your application start-up time and impact user experience.
Best Practice: If you require heavy computations, consider moving that logic into a method that can be called when needed, optimizing resource consumption.
Lessons Learned
Static blocks in Java can be a useful feature for class initialization, but they can also lead to a range of pitfalls. By understanding these common issues and adopting best practices, you can avoid problematic scenarios that could hinder your Java programming experience.
For further reading on Java initialization blocks and best practices, you can check the official Java Documentation.
By being aware of these pitfalls, you can write more effective and maintainable Java code. Remember, clarity and simplicity can often be your greatest assets in the art of programming. Happy coding!