Top Java Exception Pitfalls and How to Avoid Them
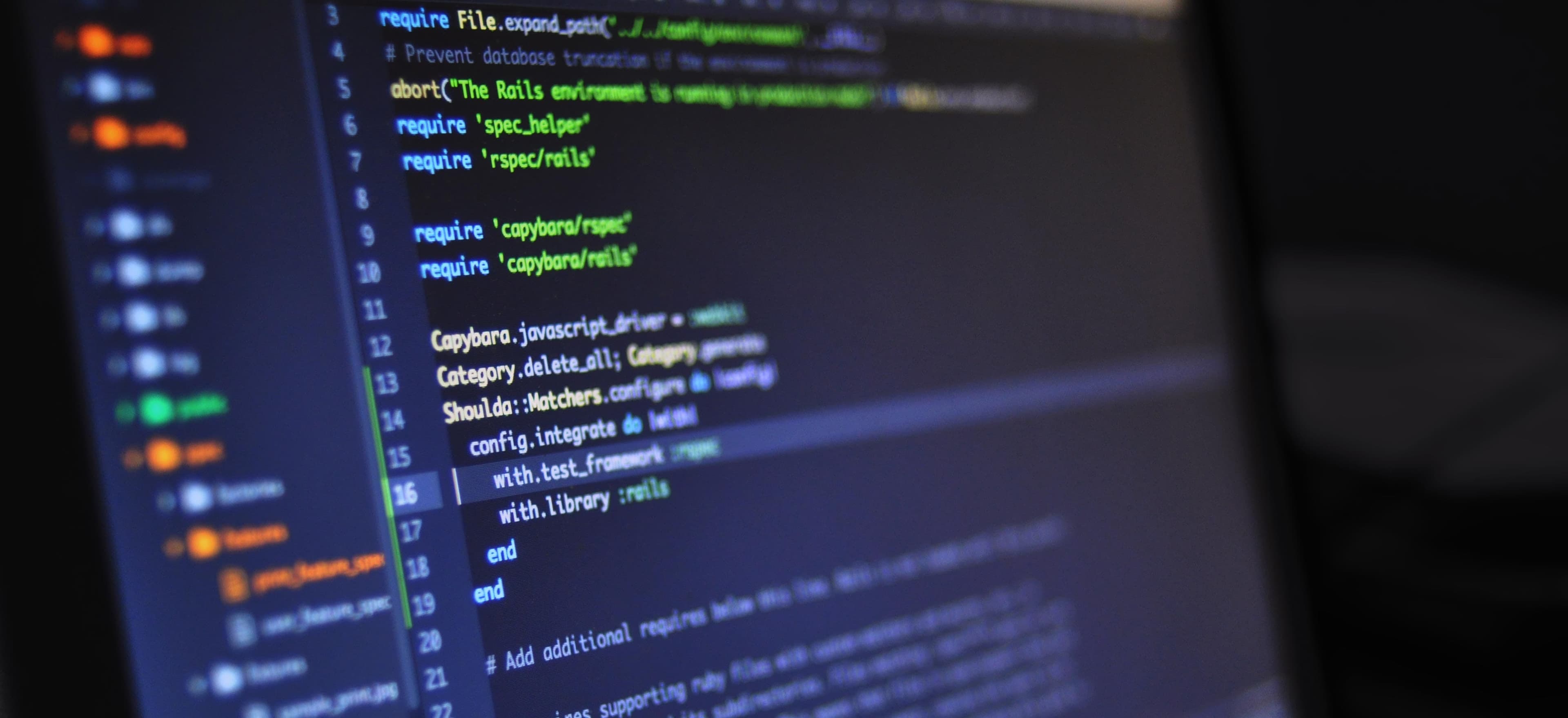
- Published on
Top Java Exception Pitfalls and How to Avoid Them
Java is a powerful programming language that is known for its robustness, primarily due to its strong exception handling capabilities. However, many Java programmers encounter common pitfalls when dealing with exceptions. In this guide, we'll explore these pitfalls, explain why they arise, and provide practical tips to avoid them. Whether you are a beginner or an experienced developer, this post will enhance your understanding of exception handling in Java.
Understanding Exceptions in Java
Before diving into the pitfalls, it’s crucial to understand what exceptions are. Simply put, exceptions are events that disrupt the normal flow of execution in a program. In Java, exceptions are classified into two main categories:
-
Checked Exceptions: These are exceptions that are checked at compile-time. The programmer must handle these exceptions, or the code will not compile. Examples include
IOException
andSQLException
. -
Unchecked Exceptions: These are exceptions that occur at runtime and are not checked at compile-time. They typically indicate programming errors, such as
NullPointerExceptions
andArrayIndexOutOfBoundsExceptions
.
Now that we have a foundational understanding, let’s delve into common pitfalls.
Pitfall 1: Overusing Exception Handling
The Issue
A prevalent mistake in Java programming is the overuse of exception handling. Some developers resort to catching all exceptions with a generic catch block without addressing specific exceptions. This obscures the specific issues that may arise.
Why It Matters
Overusing generic exception handling can lead to debugging nightmares. You may catch exceptions but not understand why they happened, making it challenging to fix the root cause.
How to Avoid This
Instead of catching Exception
, catch specific exceptions based on the situation. For instance:
try {
// Some code that may throw exceptions
} catch (IOException e) {
System.out.println("I/O error occurred: " + e.getMessage());
} catch (SQLException e) {
System.out.println("SQL error occurred: " + e.getMessage());
}
By narrowing down to the specific exceptions, you'll have more control and better logging, which is crucial in troubleshooting.
Pitfall 2: Ignoring Finally Blocks
The Issue
Many Java developers neglect the finally
block, which executes code regardless of whether an exception is thrown or caught. This can lead to resource leaks, especially with file I/O or database connections.
Why It Matters
If resources aren't released properly, it can lead to memory leaks and performance degradation.
How to Avoid This
Always use a finally
block or Java's try-with-resources statement for handling resources:
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.out.println("Error reading file: " + e.getMessage());
}
// No need for a finally block; resources are auto-closed.
The try-with-resources statement simplifies resource management and mitigates the risk of leaks.
Pitfall 3: Throwing Exceptions at the Wrong Level
The Issue
It is common for developers to throw exceptions from layers that don’t effectively communicate the problem. For example, throwing a low-level exception from a high-level service class can confuse the caller about the source of the error.
Why It Matters
Mixing low-level and high-level exception handling makes code harder to understand and maintain. It can also mislead other developers regarding error types.
How to Avoid This
Wrap low-level exceptions in a custom exception that provides meaningful context.
public class UserServiceException extends Exception {
public UserServiceException(String message, Throwable cause) {
super(message, cause);
}
}
public void fetchUser(int id) throws UserServiceException {
try {
// Logic that may throw SQLException
} catch (SQLException e) {
throw new UserServiceException("Failed to fetch user with id: " + id, e);
}
}
This way, you maintain a clear communication line regarding where the error stems from.
Pitfall 4: Catching Too Many Exceptions
The Issue
Another common pitfall is catching broader exception classes, such as Throwable
or Exception
. This can mask critical issues such as OutOfMemoryError
or StackOverflowError
.
Why It Matters
Catching all exceptions can lead to an unstable application. It hides significant problems that should be handled differently.
How to Avoid This
Catch only specific exceptions when necessary, and let the critical ones propagate:
try {
// Code that may throw exceptions
} catch (IOException e) {
System.out.println("Error reading file: " + e.getMessage());
}
// Do not catch Throwable or Exception here.
By doing this, you maintain the integrity of your application.
Pitfall 5: Not Using Custom Exceptions
The Issue
Many developers overlook creating custom exceptions tailored to the application's needs, relying solely on existing exception types.
Why It Matters
Custom exceptions help make the code self-documenting. They provide clarity and enhance the ability to handle specific scenarios relevant to the application.
How to Avoid This
Create custom exception classes when dealing with unique situations:
public class InvalidUserInputException extends Exception {
public InvalidUserInputException(String message) {
super(message);
}
}
public void validateUserInput(String input) throws InvalidUserInputException {
if (input == null || input.isEmpty()) {
throw new InvalidUserInputException("User input cannot be null or empty");
}
}
Custom exceptions clarify error handling, enhancing maintainability.
Best Practices for Exception Handling
To complement the avoidance of pitfalls, implementing best practices will further refine your approach to exception handling in Java:
- Use Specific Exceptions: Always prefer specific exception classes over general ones.
- Document Exceptions: Use Javadoc to describe the exceptions a method might throw.
- Log Exceptions: Implement logging in your catch blocks to record exceptions for future investigation. Libraries like SLF4J or Log4j are excellent choices.
- Don’t Swallow Exceptions Silently: Ensure that exceptions are not simply caught and ignored. Always handle them in a meaningful way.
- Test Exception Handling: Write unit tests that specifically test the behavior of your code under expected exceptions.
In Conclusion, Here is What Matters
Java's exception handling mechanism is powerful, but it can also lead to pitfalls if not carefully managed. By avoiding common mistakes such as overusing exception handling, neglecting finally blocks, throwing exceptions at the wrong levels, catching too many exceptions, and not creating custom exceptions, you can write cleaner, more maintainable code.
By following the best practices laid out above, you'll not only enhance your applications' resilience but also contribute to the overall health of your codebase. Remember, effective exception handling is not just about catching errors; it’s about understanding them and responding appropriately.
For further insights into Java exception handling and best practices, consider checking out Java's official documentation.
Happy coding!