Unlocking SOA: Overcoming Common Implementation Challenges
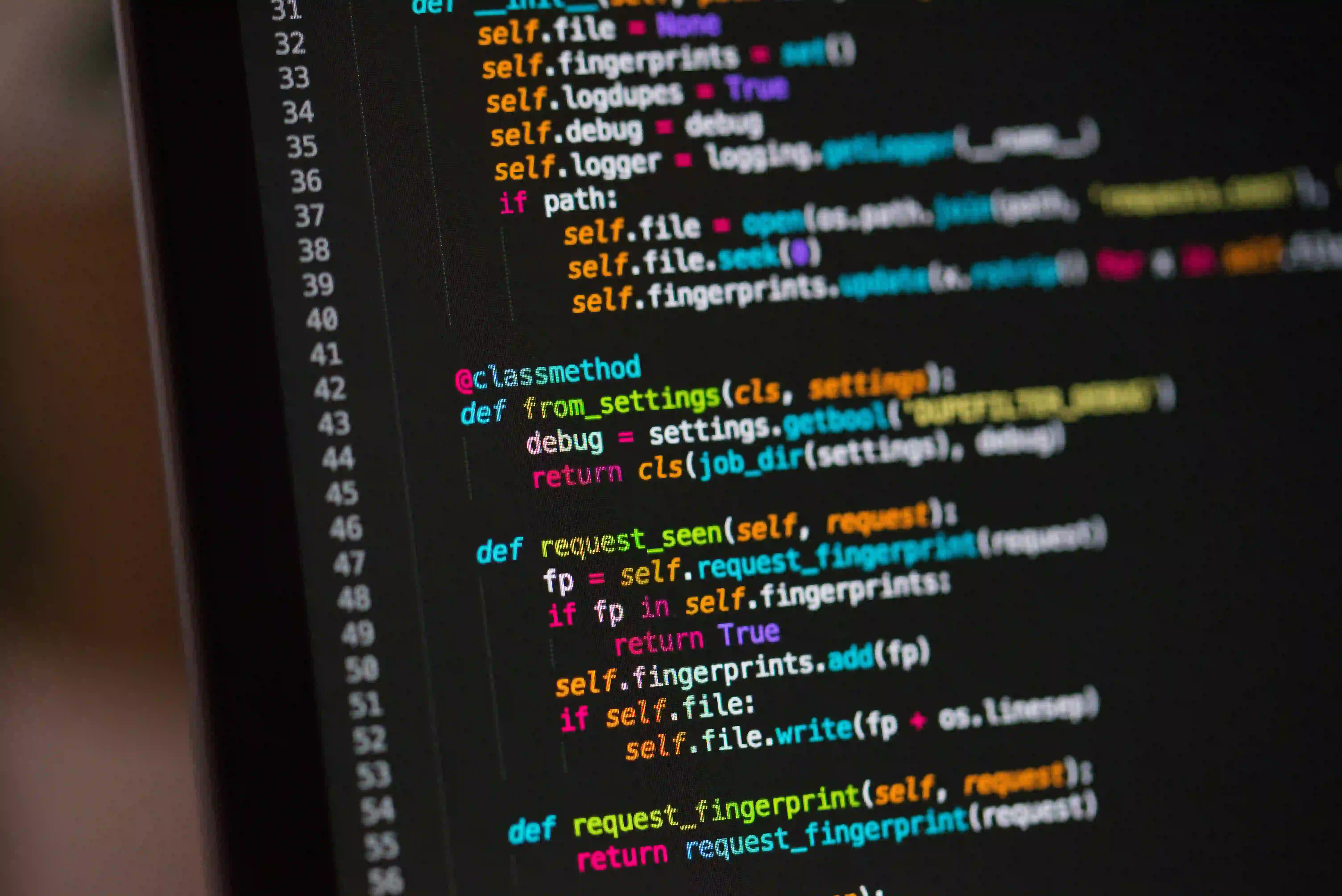
Unlocking SOA: Overcoming Common Implementation Challenges
Service-Oriented Architecture (SOA) has been a game-changer in the way businesses structure their IT infrastructure. By breaking down monolithic applications into interoperable, reusable services, organizations can achieve greater agility and flexibility. However, along with its advantages, SOA also comes with its own set of challenges during implementation. In this blog post, we'll explore those challenges and provide practical solutions to overcome them.
What is SOA?
Before diving into the challenges, let’s clarify what Service-Oriented Architecture is. SOA is a design paradigm that allows different services— software components that communicate with each other over a network—to work together. These services can be reused across different applications, enhancing efficiency and reducing redundancy.
Common Challenges in SOA Implementation
- Service Granularity
- Interoperability Issues
- Governance and Management
- Performance Concerns
- Organizational Resistance to Change
Organizations often encounter these challenges while trying to implement SOA. Below, we’ll discuss each issue in detail along with strategies to mitigate them.
1. Service Granularity
Challenge: Determining how granular your services should be is often a point of contention. Create too few services, and you may end up with a monolithic architecture. On the other hand, if you create too many services, management and orchestration become burdensome.
Solution: Employ a ‘just right’ principle when designing service granularity. Focus on creating services that encapsulate a single business function. This can be achieved through:
- Domain-Driven Design: Break down systems based on business domains. Services should reflect distinct business capabilities.
- Iterative Development: Start with a few services and refine their granularity based on feedback.
Example Code Snippet:
public class OrderService {
public Order createOrder(Customer customer, List<Item> items) {
// Create order logic
return new Order(customer, items);
}
}
Why? In this code, OrderService
focuses on a single responsibility: creating orders. This keeps our service cohesive and more manageable.
2. Interoperability Issues
Challenge: As organizations adopt SOA, they often struggle with services that cannot easily communicate with each other, especially if built using different technologies.
Solution: Use standardized protocols and data formats like RESTful APIs with JSON or SOAP-based web services. Additionally:
- Service Registry: Implement a service registry to facilitate communication between services. This helps keep track of available services and their endpoints.
- API Gateways: Use API gateways to handle requests and ensure data format consistency.
Example Code Snippet:
@RestController
@RequestMapping("/api/orders")
public class OrderController {
@Autowired
private OrderService orderService;
@PostMapping("/")
public ResponseEntity<Order> createOrder(@RequestBody OrderRequest orderRequest) {
Order order = orderService.createOrder(orderRequest.getCustomer(), orderRequest.getItems());
return ResponseEntity.ok(order);
}
}
Why? This snippet showcases a RESTful controller, providing clear API endpoints, allowing easy interoperability among various front-end applications.
3. Governance and Management
Challenge: Without a proper governance framework, organizations often face issues in ensuring compliance, maintaining security, and managing circumventions in the architecture.
Solution: Implement robust governance policies, including:
- Service Versioning: Control changes to services while ensuring backward compatibility.
- Monitoring Tools: Use monitoring solutions to track service usage, performance, and security vulnerabilities.
Example Code Snippet:
@KafkaListener(topics = "order-updates", groupId = "order_service_group")
public void listenOrderUpdates(OrderUpdateMessage message) {
// Process message
}
Why? Utilizing event-driven architecture ensures that the order service can adapt to changes in real-time, giving it the flexibility to evolve without breaking existing functionalities.
4. Performance Concerns
Challenge: SOA may introduce latency due to the increased number of network calls between services.
Solution: To combat performance issues, consider adopting:
- Caching Mechanisms: Implement caching strategies at different layers to minimize redundant data fetching.
- Asynchronous Communication: Use message brokers to facilitate asynchronous communication, reducing wait times.
Example Code Snippet:
public class OrderCache {
private Map<Long, Order> cache = new HashMap<>();
public void putOrder(Long id, Order order) {
cache.put(id, order);
}
public Order getOrder(Long id) {
return cache.getOrDefault(id, null);
}
}
Why? This caching mechanism allows for quick retrieval of order data, reducing the need for repetitive database queries and enhancing performance.
5. Organizational Resistance to Change
Challenge: Resistance to SOA can stem from team members who are accustomed to traditional methods of software development.
Solution: Use training and gradual transition methods to ease your team into SOA. Consider:
- Refactoring Existing Applications: Start with small, less critical applications to demonstrate the benefits of SOA.
- Involve Stakeholders Early: Involvement from all levels of the organization can help in addressing concerns and getting buy-in.
The Closing Argument
Implementing Service-Oriented Architecture can transform how your organization delivers software. However, to reap its full benefits, it is essential to navigate the inherent challenges with effective strategies.
By focusing on proper service granularity, establishing interoperability through standards, enforcing governance, addressing performance concerns, and managing change effectively, you can unlock the full potential of SOA.
For additional insights on SOA best practices, check out IBM's SOA Guide and Oracle's Guide on SOA. Start your journey toward an efficient and agile architecture today!