Avoiding Common Pitfalls When Swapping Spring Bean Configurations
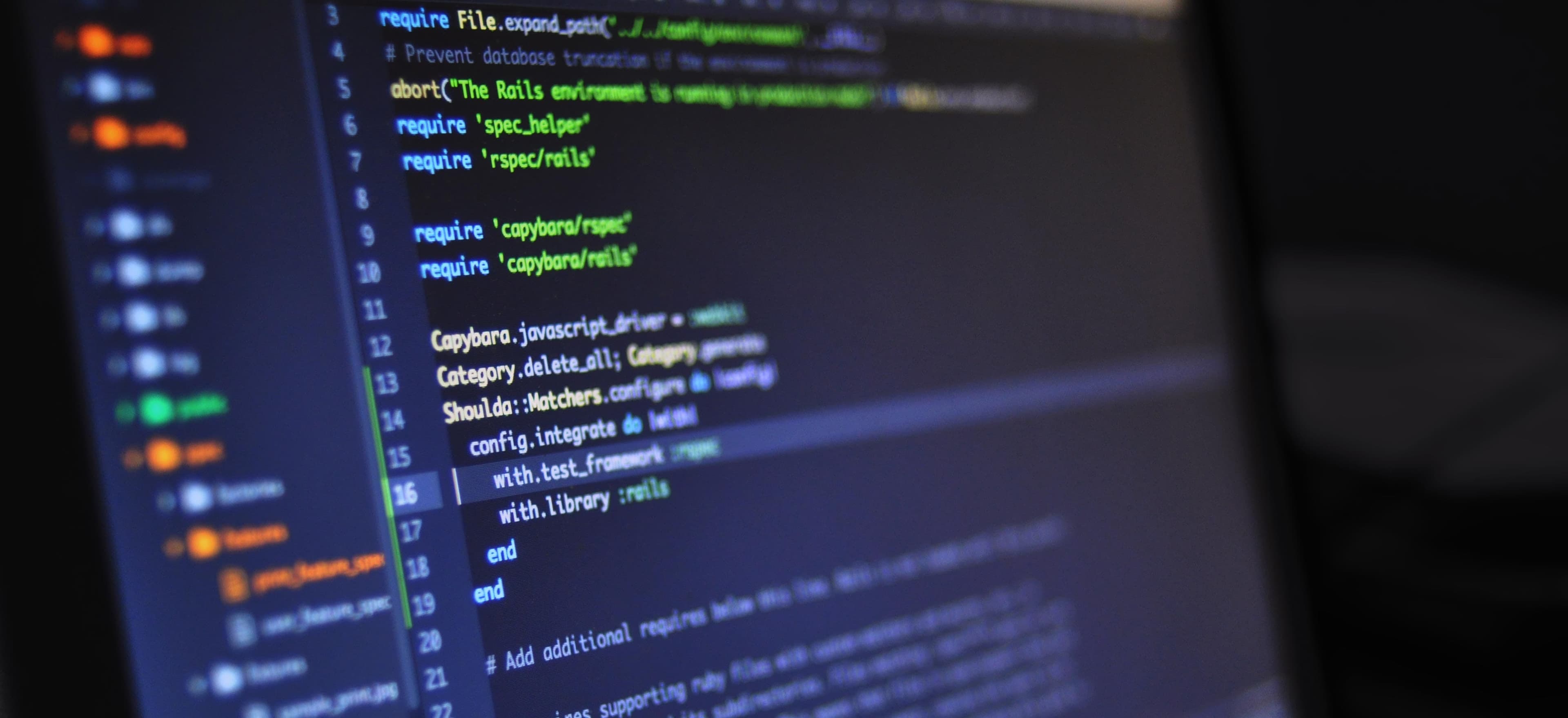
- Published on
Avoiding Common Pitfalls When Swapping Spring Bean Configurations
When working with the Spring Framework, developers often encounter various pitfalls while managing Spring Beans. As applications grow, the complexity of managing these beans also increases. One scenario that can lead to unexpected behavior is the swapping of bean configurations. This blog post will explore best practices for swapping Spring Bean configurations while avoiding common pitfalls.
Understanding Spring Beans
Spring Beans are objects that are instantiated, assembled, and managed by the Spring IoC (Inversion of Control) container. The management of beans occurs through configuration metadata, allowing developers to define their dependencies and lifecycle rather easily.
Common Issues with Spring Beans
When developers change bean configurations, they can inadvertently introduce errors in their applications. Below are some common pitfalls when swapping Spring Bean configurations:
-
Accidental Elimination of Dependencies: When replacing one bean with another, it is crucial to ensure that all required dependencies are preserved. A missing dependency can lead to
NullPointerException
at runtime. -
Scope Issues: Not understanding the scopes of beans (singleton vs. prototype) can lead to unexpected behavior. For example, if a singleton bean is swapped with a prototype bean without considering its lifecycle, it may yield conflicting states.
-
Circular Dependencies: When beans depend on each other, swapping configurations can result in circular references, essentially breaking the application context.
-
Configuration Confusion: Utilizing XML, Java-based configuration, and annotations can sometimes lead to confusion. Maintaining clarity in the configuration style is essential for easy swapping.
Best Practices for Swapping Spring Bean Configurations
Use Profiles for Configurations
Spring Profiles allow you to create different beans for different environments (e.g., development, testing, production). Instead of outright swapping beans, consider utilizing profiles.
@Configuration
@Profile("dev")
public class DevConfiguration {
@Bean
public MyService myService() {
return new MyServiceImpl();
}
}
@Configuration
@Profile("prod")
public class ProdConfiguration {
@Bean
public MyService myService() {
return new MyServiceImplWithCaching();
}
}
In this example, using profiles allows you to easily switch between beans based on the environment without directly altering the configuration.
Leverage Bean Aliasing
Another excellent way to manage different beans without complete swaps is through aliasing. This process involves defining multiple names for a single bean.
@Bean(name = {"myService", "myServicePrimary"})
public MyService myService() {
return new MyServiceImpl();
}
When adopting this approach, you can switch implementations simply by changing the alias reference in your code or configuration while keeping the beans intact.
Minimize Dependencies
When swapping beans, minimizing dependencies among beans can reduce complexity. Use interfaces to decouple components as much as possible.
public interface MyService {
void performAction();
}
@Service
public class MyServiceImpl implements MyService {
@Override
public void performAction() {
// Implementation details
}
}
By using interfaces, you provide yourself with the flexibility to swap implementations without modifying the dependent beans.
Refactor for Clarity
In large applications, refactoring code to improve understandability can ease the process of swapping bean configurations. For instance, split large configuration classes into smaller, more focused ones.
@Configuration
public class ServiceConfiguration {
@Bean
public MyService myService() {
return new MyServiceImpl();
}
}
@Configuration
public class RepositoryConfiguration {
@Bean
public MyRepository myRepository() {
return new MyRepositoryImpl();
}
}
Clear separation of concerns improves readability and makes it easier to manage changes.
Monitor Bean Lifecycles
It is crucial to be aware of the lifecycles of beans. Using Spring's @PostConstruct
and @PreDestroy
annotations will help in managing initialization and destruction respectively.
@Component
public class MyService {
@PostConstruct
public void init() {
// Initialization logic (e.g., connecting to a database)
}
@PreDestroy
public void cleanup() {
// Cleanup logic (e.g., closing resources)
}
}
Understanding and properly managing lifecycles can help ensure that no resources are leaked during bean swaps.
Testing, Testing, Testing
Finally, thorough testing is your best safeguard against issues arising from swapping configurations. Use integration tests to verify that all dependencies resolve correctly and that the application behaves as expected.
Here is an example of a simple JUnit test with the Spring Test context:
@RunWith(SpringRunner.class)
@ContextConfiguration(classes = {ServiceConfiguration.class, RepositoryConfiguration.class})
public class MyServiceTest {
@Autowired
private MyService myService;
@Test
public void testPerformAction() {
myService.performAction();
assertTrue(/* condition to validate the action performed */);
}
}
With tests in place, you can refactor and swap bean configurations more confidently.
Lessons Learned
Swapping Spring Bean configurations is a common scenario but can easily lead to complications if not handled carefully. By employing Spring Profiles, leveraging bean aliasing, minimizing dependencies, refactoring for clarity, monitoring bean lifecycles, and implementing robust testing practices, developers can mitigate common pitfalls effectively.
Adopting these best practices will enhance maintainability and scalability within your application. As you proceed with your Spring projects, keep these insights in mind to ensure a smooth development experience.
For additional reading on Spring Dependency Injection and Bean Management, check out the official Spring documentation.
Happy Coding!
Checkout our other articles