Debunking Docker Myths: Java Developers' Common Pitfalls
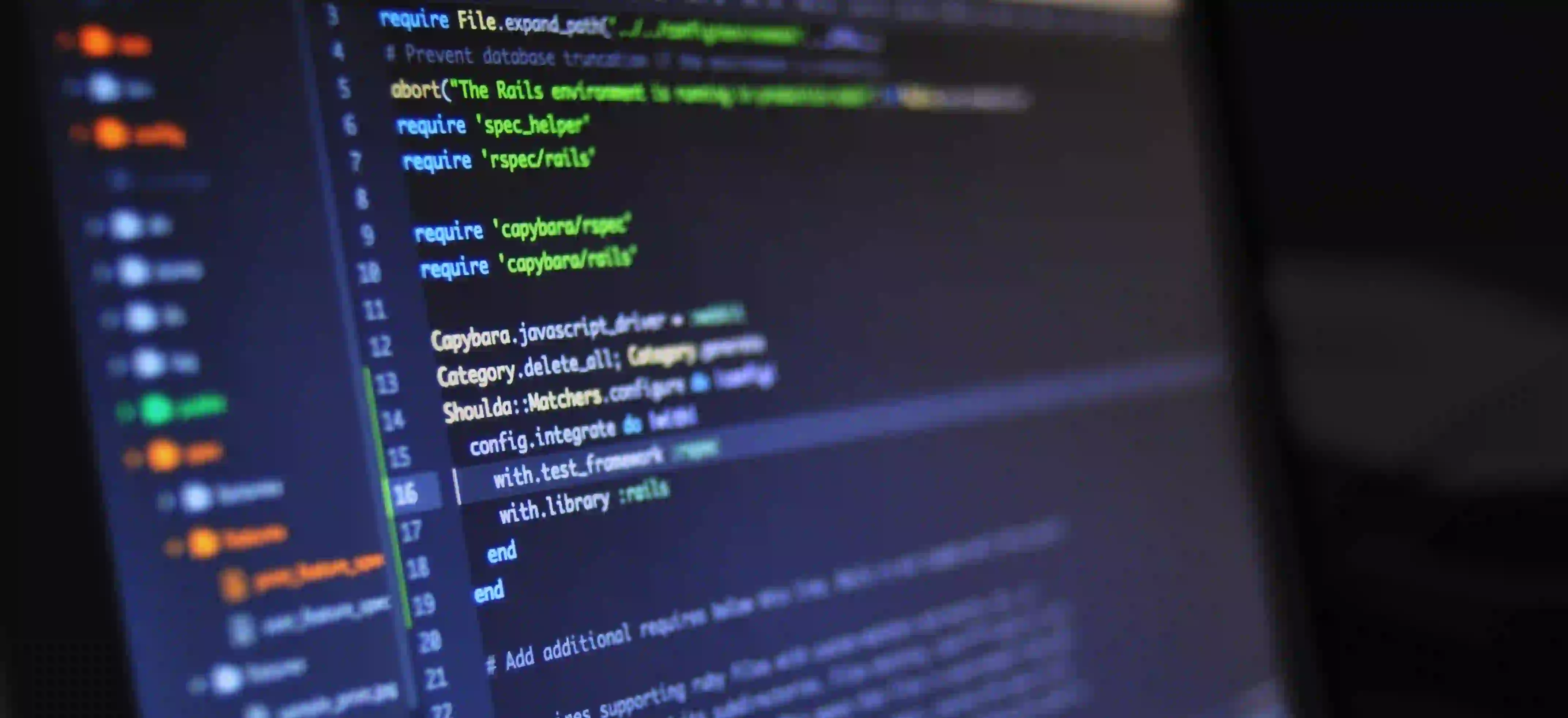
Debunking Docker Myths: Java Developers' Common Pitfalls
Docker has revolutionized the way developers deploy and scale applications. For Java developers, it offers a compelling avenue for packaging applications with their dependencies. However, despite its popularity, several myths surrounding Docker persist, leading to misconceptions and potential pitfalls. In this blog post, we'll explore these myths and clarify the best practices for using Docker effectively in Java development.
What is Docker?
Before delving into the myths, let's quickly revisit what Docker is. Docker is an open-source platform that automates the deployment of applications inside lightweight, portable containers. This means that you can package your Java application, including all its dependencies, into a container and run it consistently across various environments.
!Docker Architecture
Myth 1: Docker is Only for Microservices
Reality: While Docker shines in microservices architectures due to its lightweight and fast nature, it isn't solely limited to microservices.
Understanding Monolithic Applications
Many existing Java applications are monolithic. They bundle all functionalities into a single unit. Docker can still benefit monolithic applications by isolating them in containers, allowing for easier management and deployment.
For instance, let's say you have a simple Java application configured in Maven:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>myapp</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>jar</packaging>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.2</version>
</dependency>
</dependencies>
</project>
Dockerfile Example
You can use Docker to create a container for this Java application without converting it into microservices. Here's a sample Dockerfile:
# Use the official OpenJDK image from Docker Hub
FROM openjdk:11-jre-slim
# Set the working directory inside the container
WORKDIR /app
# Copy the JAR file from the target directory
COPY target/myapp-1.0-SNAPSHOT.jar myapp.jar
# Expose the application port
EXPOSE 8080
# Run the application
CMD ["java", "-jar", "myapp.jar"]
This Dockerfile simplifies the deployment process by providing a consistent environment across different stages of development and production. It saves you from the intricacies of "works on my machine" situations.
Myth 2: Docker Guarantees Performance
Reality: Docker provides a performance boost but does not guarantee it. Containerization adds a layer of abstraction, which can sometimes lead to unexpected latency.
Understanding Performance Optimization
One common pitfall is assuming that simply containerizing your application will improve its performance. It’s crucial to optimize your Java application and its Docker configuration.
For example, allocate sufficient memory and CPU resources when running the container. You can specify resource limits as follows:
docker run -m 512m --cpus=1 myapp
Jaeger for Performance Monitoring
Integrating performance monitoring tools like Jaeger can offer insights into your application’s behavior inside Docker. You can analyze traces and improve performance by resolving bottlenecks. More about Jaeger can be found here.
Myth 3: Docker Images are Static
Reality: Docker images can be built, modified, and optimized over time. Stagnation is not the goal.
Continuous Integration & Deployment (CI/CD)
In a CI/CD setup, it's common to rebuild Docker images for every code change. Here’s a snippet of how to automate the process using GitHub Actions:
name: CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Build Docker image
run: docker build . -t myapp:latest
- name: Push Docker image
run: docker push myapp:latest
This workflow automatically rebuilds your Docker images whenever you push code changes, ensuring your containers are always up-to-date.
Myth 4: Debugging Dockerized Applications is Difficult
Reality: Debugging can be more complex but also offers unique advantages.
Docker Exec for Real-Time Debugging
Using docker exec
enables developers to enter a running container and perform real-time debugging. Here’s how you can do this:
docker exec -it <container_id> sh
Inside the container, you can run Java commands to inspect the application in context, significantly simplifying the debugging process.
Myth 5: All Java Applications Work Well with Docker
Reality: Not all Java applications are inherently Docker-friendly.
Performance Characteristics
Certain Java applications might rely heavily on I/O operations or have specific JVM settings that do not translate well into a containerized environment. Always ensure your application is tested within the container.
Running an Application in Full Control
Consider a scenario where your Java application uses JNI (Java Native Interface). There may be additional configurations required for compatibility within Docker. Always check Docker's documentation and consider the following when running JNI applications:
-
Ensure that your Docker image includes the necessary native libraries.
-
Test the performance in a Dockerized system.
Key Takeaways
Docker is a powerful tool that aids Java developers in building and deploying applications. However, it's essential to debunk the myths surrounding it to avoid common pitfalls. From emphasizing that Docker is not exclusively for microservices, understanding performance implications, being aware of continuous integration practices, to comprehending the nuances of debugging, Java developers must approach Docker with informed caution.
For more information, you can explore Docker's official documentation and Java in Docker for comprehensive guides on Titanic Java applications with Docker.
Remember, the key to mastering Docker lies in continuous learning and experimentation. Mastering these fundamentals will enable you to create efficient, reliable, and scalable Java applications.
Now, go ahead and start containerizing your Java applications, and steer clear of those common myths!