Mastering Security: Safeguarding Secrets in Java Microservices
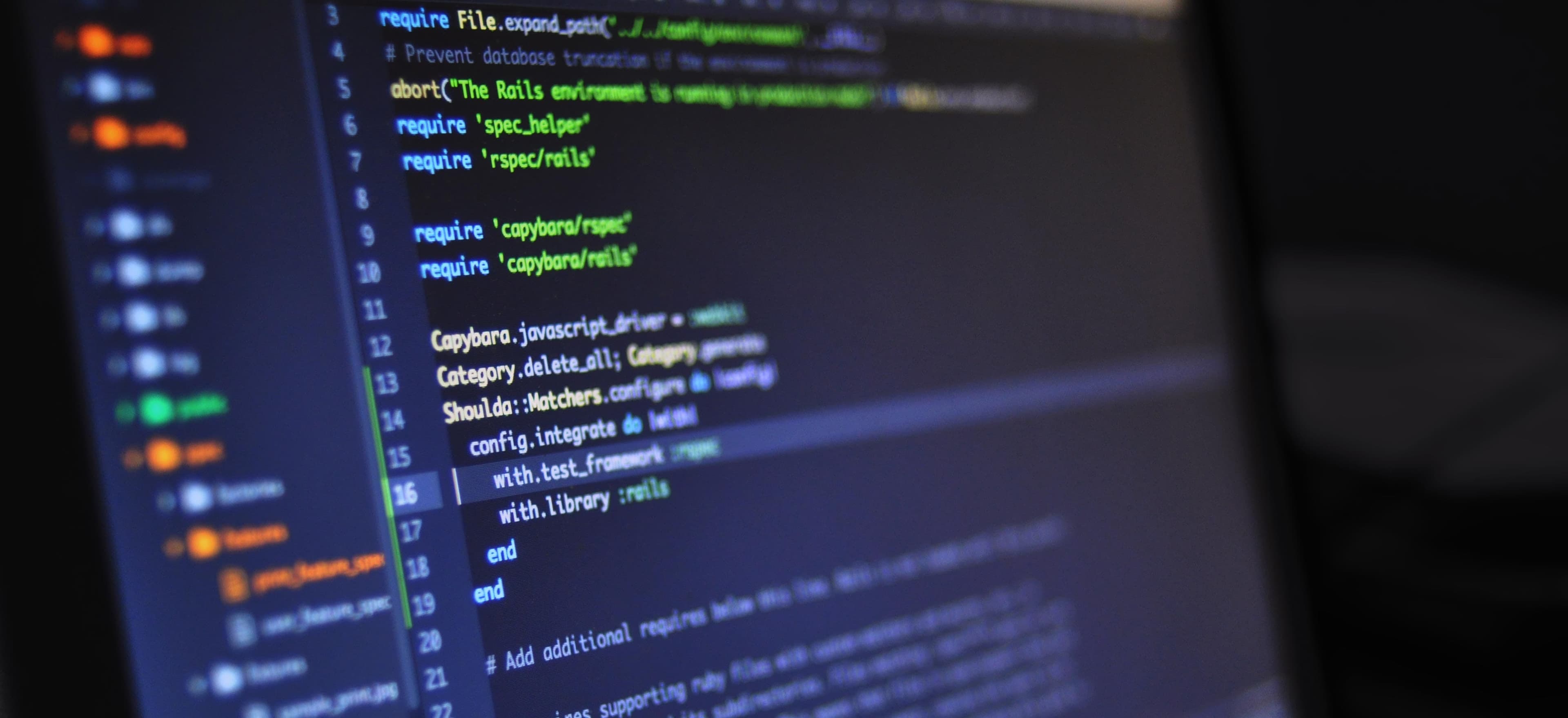
- Published on
Mastering Security: Safeguarding Secrets in Java Microservices
In today's digital landscape, security is not merely an option; rather, it is a necessity, particularly for Java microservices designed to operate in distributed environments. With the increasing complexity of microservices architectures, the risk of exposing sensitive information has also escalated. This blog post will guide you through best practices for safeguarding secrets, such as API keys, passwords, and any sensitive configuration data in your Java microservices.
Understanding Secrets Management
Secrets management involves storing and accessing sensitive information in a secure manner. In microservices, each service often requires its own secrets, which can lead to challenges in terms of both security and scalability.
Why is Secrets Management Important?
- Data Protection: Prevents unauthorized access to sensitive data.
- Compliance: Helps meet regulatory requirements (e.g., GDPR, HIPAA).
- Operational Integrity: Ensures the services function as intended without vulnerabilities.
Best Practices for Secrets Management
1. Avoid Hardcoding Secrets
Hardcoding sensitive information directly in your code is a recipe for disaster. It exposes your secrets to anyone with access to your codebase.
Consider this example:
public class DatabaseConfig {
private static final String DB_USER = "admin";
private static final String DB_PASSWORD = "password123"; // Hardcoded secret
public static void connect() {
// Connect to the database
}
}
Why Avoid Hardcoding?
- Version Control Leakage: If you use version control (e.g., Git), any pushes to your repository expose these secrets.
- Inefficiency: Changing the secret involves code modification, breaking the deployment flow.
Instead, utilize environment variables or configuration files to read secrets at runtime:
public class DatabaseConfig {
private static final String DB_USER = System.getenv("DB_USER");
private static final String DB_PASSWORD = System.getenv("DB_PASSWORD");
public static void connect() {
// Connect to the database using credentials
}
}
2. Use Configuration Management Tools
Tools like HashiCorp Consul, Spring Cloud Config, and Envoy provide effective ways to manage secrets. These tools allow you to externalize and manage secrets securely.
For example, with Spring Cloud Config, you can manage properties in a Git repository securely. Here’s how you can set it up:
- Add Dependency: Include it in your
pom.xml
.
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
- Application Properties: Define your config server properties.
spring:
cloud:
config:
uri: http://localhost:8888 # Config server URL
- Accessing Secrets:
@Value("${db.user}")
private String dbUser;
@Value("${db.password}")
private String dbPassword;
3. Leverage Secret Management Services
Using dedicated services for secret management, like AWS Secrets Manager or Azure Key Vault, helps keep your secrets secure. These services offer features such as automatic key rotation, logging, and encryption.
AWS Secrets Manager Example
- Storing a Secret:
You can store your secret in AWS Secrets Manager via their console or AWS CLI.
- Retrieving Secrets:
Use the AWS SDK in your Java microservice:
import com.amazonaws.services.secretsmanager.AWSSecretsManager;
import com.amazonaws.services.secretsmanager.AWSSecretsManagerClientBuilder;
import com.amazonaws.services.secretsmanager.model.GetSecretValueRequest;
public class SecretManager {
public String getSecret(String secretName) {
AWSSecretsManager client = AWSSecretsManagerClientBuilder.standard().build();
GetSecretValueRequest getSecretValueRequest = new GetSecretValueRequest()
.withSecretId(secretName);
return client.getSecretValue(getSecretValueRequest).getSecretString();
}
}
4. Secure Communication
Ensure that secrets are transmitted securely. Use HTTPS for API communication and employ TLS for data in transit.
HttpURLConnection connection = (HttpURLConnection) new URL("https://api.yourservice.com/").openConnection();
connection.setRequestMethod("GET");
5. Encrypt Secrets
Encryption is the last line of defense. Even if an attacker accesses your secrets, encryption will mitigate the risk. Use Java’s built-in libraries or third-party libraries like Bouncy Castle.
Example using AES encryption:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class EncryptionUtil {
public static String encrypt(String data) throws Exception {
SecretKey key = KeyGenerator.getInstance("AES").generateKey();
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encryptedData = cipher.doFinal(data.getBytes());
return new String(encryptedData);
}
}
Why Use Encryption?
- Data Interception: Even intercepted data remains secure.
- Compliance Requirements: Many regulations mandate encryption of sensitive information.
6. Audit and Monitor
Establish a robust logging and monitoring system to detect unauthorized access to your secrets. Adjust your secrets management strategy based on audit findings.
Tools like Splunk or ELK Stack can help you visualize and analyze logs.
Closing the Chapter
In summary, securing secrets in Java microservices requires adopting best practices like avoiding hardcoding, using secrets management services, encrypting data, and maintaining a robust audit and monitoring system.
While the journey of mastering security can be daunting, following these guidelines will lead you toward a more secure microservices architecture.
Staying informed about continuous advancements in security practices is crucial because the landscape is constantly evolving. Consider checking out resources such as OWASP for enriching your knowledge on application security.
Remember, in the realm of microservices, the safety of your secrets is directly proportional to the trust your application earns from its users. Meet their expectations and safeguard their data diligently. Happy coding!
Checkout our other articles