Boost App Performance with Smart Distributed Caching
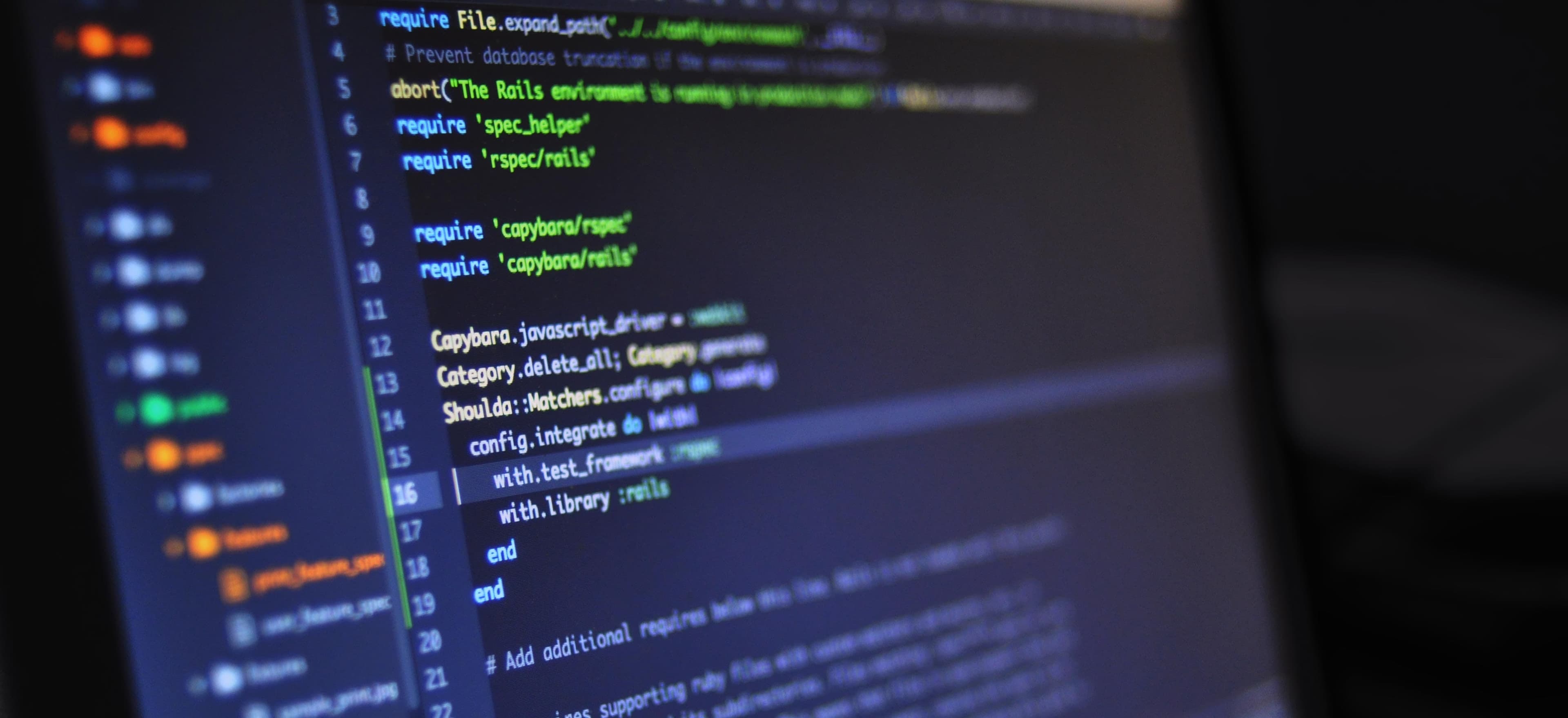
- Published on
Boost App Performance with Smart Distributed Caching
In the rapidly evolving landscape of web applications, performance is everything. Users expect fast, responsive applications that can handle their demands seamlessly. One effective strategy for enhancing performance is distributed caching. In this blog post, we'll explore what distributed caching is, why it matters, and how to implement it in Java applications, supplemented with practical examples and best practices.
What is Distributed Caching?
Distributed caching is an architecture that allows data to be stored and accessed across multiple servers. Unlike traditional caching, which might reside on a single machine, distributed caching distributes data across a server cluster, ensuring high availability, fault tolerance, and scalability. This method significantly improves the read performance of frequently accessed data, reducing the load on your primary database and speeding up your application.
Why Use Distributed Caching?
-
Improved Performance: By storing data closer to where it is needed, you can drastically reduce latency and improve retrieval times.
-
Scalability: A distributed cache can effortlessly scale out by adding more nodes, allowing you to handle increased loads without significant modifications to your existing infrastructure.
-
Reduced Database Load: By caching frequently accessed data, fewer read requests hit your database, allowing it to focus on write operations and lessening the likelihood of bottlenecks.
-
Increased Availability: If one node in your cache fails, others continue to serve requests, ensuring your application remains available.
How to Implement Distributed Caching in Java
Let’s dive into the practical side of things. We will implement a distributed cache using Hazelcast, one of the most popular in-memory data grids. Hazelcast is easy to set up and integrates well with Java applications.
Getting Started with Hazelcast
Start by adding the Hazelcast dependency to your Maven project:
<dependency>
<groupId>com.hazelcast</groupId>
<artifactId>hazelcast</artifactId>
<version>5.2</version>
</dependency>
You can find the latest version of Hazelcast on Maven Central.
Basic Configuration
Next, let's set up a simple distributed cache configuration.
import com.hazelcast.config.Config;
import com.hazelcast.core.Hazelcast;
import com.hazelcast.core.HazelcastInstance;
public class CacheServer {
public static void main(String[] args) {
Config config = new Config();
HazelcastInstance hazelcastInstance = Hazelcast.newHazelcastInstance(config);
// Starting the Hazelcast instance
System.out.println("Hazelcast Instance started: " + hazelcastInstance.getCluster());
}
}
Code Explanation
- Config: This class allows you to customize the configuration settings of your Hazelcast instance.
- HazelcastInstance: Represents a member of the Hazelcast cluster. Multiple nodes can be created, allowing data to be distributed.
Caching Data
Now, let’s cache some data.
import com.hazelcast.core.Hazelcast;
import com.hazelcast.core.HazelcastInstance;
import com.hazelcast.map.IMap;
public class CacheData {
public static void main(String[] args) {
HazelcastInstance hazelcastInstance = Hazelcast.newHazelcastInstance();
IMap<Integer, String> map = hazelcastInstance.getMap("dataCache");
// Storing data in the cache
map.put(1, "Hello, Distributed Caching!");
map.put(2, "Caching with Hazelcast is easy.");
// Retrieving data from the cache
System.out.println("Value for key 1: " + map.get(1));
System.out.println("Value for key 2: " + map.get(2));
}
}
Code Explanation
- IMap: An interface that represents a distributed map, which automatically handles data partitioning.
- put(): Adds key-value pairs to the cache.
- get(): Retrieves values based on the specified key.
Benefits of Distributed Caching with Hazelcast
Utilizing Hazelcast for distributed caching brings several advantages:
-
Ease of Use: Hazelcast’s API is straightforward, allowing developers to quickly implement caching solutions.
-
Comprehensive Documentation: Comprehensive user guides and a supportive community can help streamline development.
-
Scalability: It allows for on-the-fly scaling of resources, which is crucial as your application grows.
-
Clustering: Hazelcast automatically handles distribution of data across clusters, making it easier for developers.
Advanced Caching Techniques
Expiring Cache Entries
One common caching scenario involves setting expiration policies on cached entries. Hazelcast supports time-to-live (TTL) with ease.
map.put(3, "Temporary Data", 10, TimeUnit.SECONDS); // Data expires after 10 seconds
This command puts data into the cache that automatically expires after ten seconds. This is particularly useful for scenarios where freshness is crucial.
Cache Replication
For higher availability and fault tolerance, Hazelcast supports data replication across nodes. You can configure backup options in Config
.
Config config = new Config();
config.getMapConfig("dataCache").setBackupCount(1);
This configuration ensures that there is one backup for each partition in the cache, enhancing reliability.
Best Practices for Distributed Caching
-
Use Appropriate Keys: Ensure keys are unique and thoughtfully constructed, as this will drastically reduce cache misses.
-
Regularly Monitor Cache Usage: Keep track of cache hits and misses using monitoring tools. This can help identify bottlenecks and areas of improvement.
-
Cache Only What You Need: Avoid caching unnecessary data. Cache selective data that will improve performance substantially.
-
Implement a Cache Eviction Policy: Define clear rules on when and how data should be removed from the cache, such as LRU (Least Recently Used) or TTL.
-
Test and Scale: Regularly test your application's responses under load and scale your caching nodes as needed for optimal performance.
In Conclusion, Here is What Matters
Distributed caching with tools like Hazelcast can significantly boost the performance of your Java applications. By implementing smart caching strategies, you not only improve response times but also enhance scalability and system resilience.
For further reading and an in-depth dive into advanced caching strategies, check out Hazelcast Documentation.
Furthermore, if you're interested in the broader implications of distributed systems on performance, you may want to read about Microservices Architecture.
In the fast-paced world of application development, leveraging distributed caching is a powerful technique to stay ahead. Implementing these strategies will place you in a stronger position to deliver high-performing applications that meet your users’ expectations. Happy coding!
Checkout our other articles