Common Errors in Java Prime Number Programs and Fixes
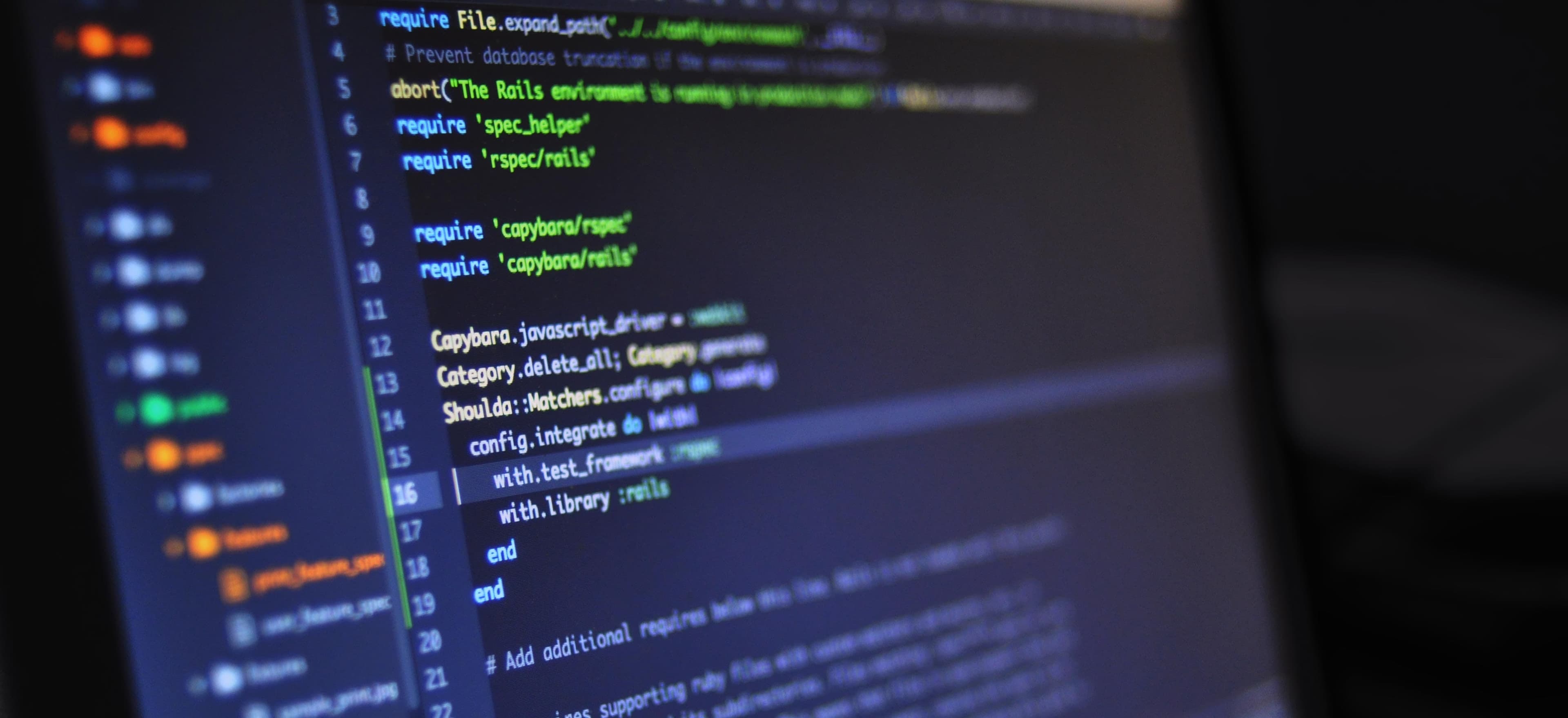
- Published on
Common Errors in Java Prime Number Programs and Fixes
Java is a highly versatile and widely-used programming language, often a go-to for aspiring developers. Among its numerous applications, one popular task is writing programs to identify prime numbers. While the task may seem straightforward, many learners encounter common pitfalls. In this blog post, we will explore the common errors in Java prime number programs and their corresponding fixes.
Understanding Prime Numbers
Before diving into errors, let's define prime numbers. A prime number is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. For example, 2, 3, 5, 7, and 11 are prime numbers, while 4, 6, 8, and 9 are not, as they have divisors other than 1 and themselves.
Basic Prime Number Algorithm
First, let’s discuss a simple algorithm to check whether a number is prime, which will help us understand potential errors. Below is the basic code structure:
public class PrimeChecker {
public static boolean isPrime(int number) {
if (number <= 1) {
return false; // Numbers less than or equal to 1 are not prime
}
for (int i = 2; i <= Math.sqrt(number); i++) {
if (number % i == 0) {
return false; // Found a divisor, so not prime
}
}
return true; // No divisors found, number is prime
}
public static void main(String[] args) {
int num = 17; // Change this number to test
if (isPrime(num)) {
System.out.println(num + " is a prime number.");
} else {
System.out.println(num + " is not a prime number.");
}
}
}
In this example, the isPrime
method checks if the given number is prime and returns a boolean value accordingly. Now, let's tackle common mistakes developers might encounter when writing similar programs.
Common Errors and Fixes
Error 1: Incorrect Handling of Edge Cases
Problem: Many beginners overlook edge cases, particularly the values 0 and 1. These numbers are not prime, yet they are often included in checks.
Fix: Always include a condition to handle numbers less than or equal to 1.
if (number <= 1) {
return false;
}
Error 2: Inefficient Looping
Problem: Some naïve implementations check all numbers up to the integer itself to determine if it is prime, leading to unnecessary computations.
Fix: You can limit the loop to the square root of the number. If n
has a factor larger than its square root, the corresponding factor must be smaller than the square root.
for (int i = 2; i <= Math.sqrt(number); i++) {
if (number % i == 0) {
return false;
}
}
Error 3: Unintended Variable Scope
Problem: Sometimes, developers introduce local variables that unintentionally shadow a field. This can lead to unexpected behaviors.
Fix: Name your variables clearly and ensure you do not unintentionally name a local variable the same as a field.
Error 4: Off-By-One Errors
Problem: Off-by-one errors are prevalent and can occur within looping conditions, leading developers to incorrectly conclude if a number is prime.
Fix: Pay close attention to loop boundaries. Review them carefully to ensure they align with the intended logic.
for (int i = 2; i <= Math.sqrt(number); i++) { // Correct boundary
// Logic...
}
Error 5: Handling Negative Numbers
Problem: Some implementations ignore negative numbers, which could lead to incorrect outputs or exceptions.
Fix: Properly handle negative input by returning false immediately.
if (number < 0) {
return false; // Negative numbers cannot be prime
}
Optimized Prime Checking
For a production-level application, you may want a more performance-optimized prime checking function. Here’s an updated version of our previous implementation:
public class OptimizedPrimeChecker {
public static boolean isOptimizedPrime(int number) {
if (number <= 1) {
return false;
}
if (number <= 3) {
return true; // 2 and 3 are prime
}
if (number % 2 == 0 || number % 3 == 0) {
return false; // Eliminate multiples of 2 and 3
}
for (int i = 5; i * i <= number; i += 6) { // Check factors of 6
if (number % i == 0 || number % (i + 2) == 0) {
return false;
}
}
return true;
}
public static void main(String[] args) {
int num = 29; // Change this number to test
if (isOptimizedPrime(num)) {
System.out.println(num + " is a prime number.");
} else {
System.out.println(num + " is not a prime number.");
}
}
}
Explanation of the Optimized Code
- Initial Checks: We first eliminate numbers less than or equal to 1 and quickly identify the primes 2 and 3.
- Exclusion of Multiples: We eliminate even numbers and multiples of 3 right away.
- Incremental Checking: By testing potential factors in increments of 6 (i.e., 5, 11, 17...), we only check numbers of the form 6k ± 1. This is based on the fact that all primes are of this form after 2 and 3.
For detailed algorithms behind prime number generation and sieves, check out Wikipedia's article on Prime Numbers.
The Last Word
Identifying prime numbers in Java can be a straightforward task, but be mindful of common pitfalls and errors. We discussed several errors such as handling edge cases, improper loop boundaries, and inefficiencies in logic. When written correctly, a Java prime number program is not only functional but also efficient.
Feel free to experiment with the code snippets provided, and explore further advancements in prime number algorithms. Happy coding!
For further reading, consider checking out Oracle's Java Documentation for a deeper understanding of Java programming concepts.
Additional Resources
- Java Primitive Data Types: Java Documentation
- Additional Java Programming Practices: GeeksforGeeks Java Practices
This exploration of common errors in Java prime number programs serves as a foundation for debugging and refining your coding practices. With patience and attention to detail, you'll soon overcome these hurdles!
Checkout our other articles