Managing Java 16 Records with JPA and jOOQ: Common Issues
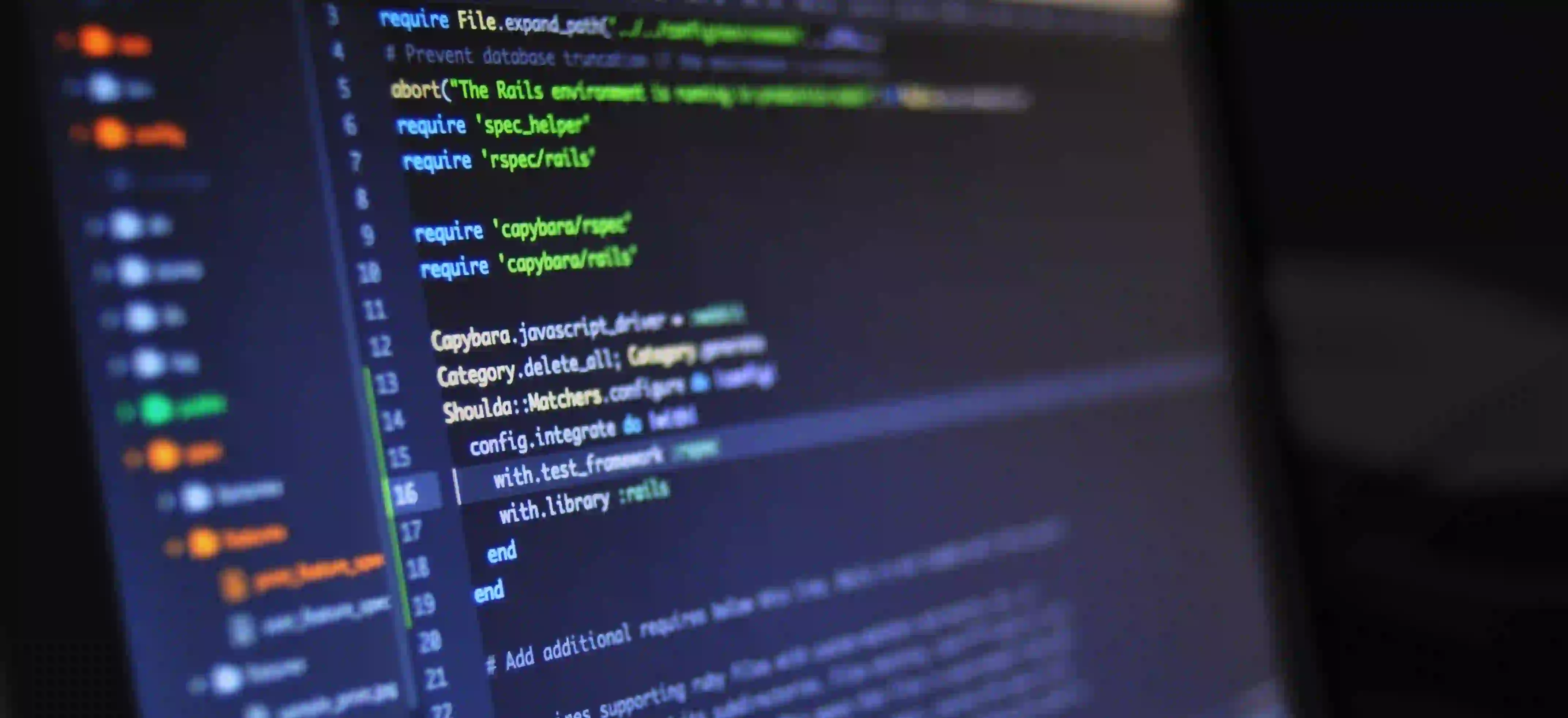
Managing Java 16 Records with JPA and jOOQ: Common Issues
As Java continues to evolve, developers are consistently greeted with new features that enhance language capabilities. One of the major introductions in Java 16 is Records. Records offer a compact, immutable way to create data transfer objects (DTOs). Coupled with JPA (Java Persistence API) and jOOQ (Java Object Oriented Querying), managing these records effectively can be a challenging endeavor, especially when navigating common issues. In this blog post, we will explore the intersection of Record management with JPA and jOOQ, discuss common problems, and provide practical solutions.
Understanding Records
Java Records are a feature introduced to simplify the task of creating classes that primarily carry data. They are immutable, final classes that extend java.lang.Record
. Here's a simple example:
public record User(String username, String email) {}
This definition automatically provides you with several useful methods like equals()
, hashCode()
, and toString()
.
Why Use Records?
- Conciseness: The syntax is much shorter, reducing boilerplate code.
- Immutability: Records are inherently immutable, enhancing data integrity.
- Readability: Records clearly indicate that the class is intended for data storage.
JPA and Records
JPA simplifies database operations but was primarily designed before Records were introduced. Hence, integrating Records into your JPA workflows can lead to several common issues.
Common JPA Issues with Records
1. Unsupported Type:
JPA does not natively support records for mapping to database entities. You might encounter java.lang.IllegalArgumentException: Not a known class
if you try to use records directly.
Solution: Use a traditional entity class or leverage a DTO pattern. Here's how you could adapt the User Record for JPA:
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class UserEntity {
@Id
private Long id;
private String username;
private String email;
// Constructors, Getters, and Setters
}
By using an entity class instead of a record, you can ensure that JPA can work with your data.
2. No Default Constructor:
Records do not have a default constructor, which is a requirement for JPA entities.
Solution: Use a builder approach or another DTO that can be populated via JPA and then convert it to a Record if needed.
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class UserEntity {
@Id
private Long id;
private String username;
private String email;
// Provide a no-arg constructor
public UserEntity() {}
// Custom constructor for creating a UserEntity
public UserEntity(String username, String email) {
this.username = username;
this.email = email;
}
// Convert to Record
public User toRecord() {
return new User(username, email);
}
}
This code snippet demonstrates how to create a UserEntity
with a no-arg constructor and a conversion method back to the User record.
jOOQ and Records
jOOQ is a popular library for building SQL queries in a type-safe manner. When dealing with records and jOOQ, similar issues can arise.
Common jOOQ Issues with Records
1. Schema Generation:
Like JPA, jOOQ does not recognize records out-of-the-box for generation of schemas.
Solution: Use generator classes or map to a standard Java class. Here’s an example:
public class UserRecord extends TableRecord<UserRecord> {
// Define your fields
private String username;
private String email;
// Constructor
public UserRecord(String username, String email) {
this.username = username;
this.email = email;
}
}
2. Type Safety:
jOOQ works with a code generation feature that might not line up properly with the record structure.
Solution: Generate jOOQ classes for your database schema and then use them in combination with Records.
Example of Usage
Here’s how you could use both jOOQ and Records together effectively.
import static org.jooq.impl.DSL.*;
public void insertUser(UserRecord user, DSLContext dsl) {
dsl.insertInto(table("users"))
.columns(field("username"), field("email"))
.values(user.username(), user.email())
.execute();
}
In this snippet, dsl
is a context from jOOQ, which allows you to perform database operations using fluent API calls.
Final Thoughts
Managing Java 16 records with JPA and jOOQ provides developers with enhanced capabilities but also introduces certain complexities. Moving from traditional entity management to leveraging records in conjunction with database libraries requires some adaptation in approach.
Best Practices
- Use DTOs or Traditional Entities: For JPA, prefer using traditional entities or DTOs that encapsulate the records, ensuring that the framework's requirements are met.
- Utilize jOOQ’s Code Generation: This approach maintains strong type safety, ensuring fewer runtime errors and clearer code.
- Consider Immutability Needs: Decide when immutability is a necessary feature, as it can help guide your choices in design.
For those interested in further exploring these methodologies, you can check out the JPA Documentation and jOOQ User Guide for more insights and advanced topics.
With this newfound understanding, you’re better equipped to navigate the challenges associated with records in JPA and jOOQ, enhancing your Java applications for a more efficient data handling experience.