Struggling with Customization? Optimize Your Data Store Today!
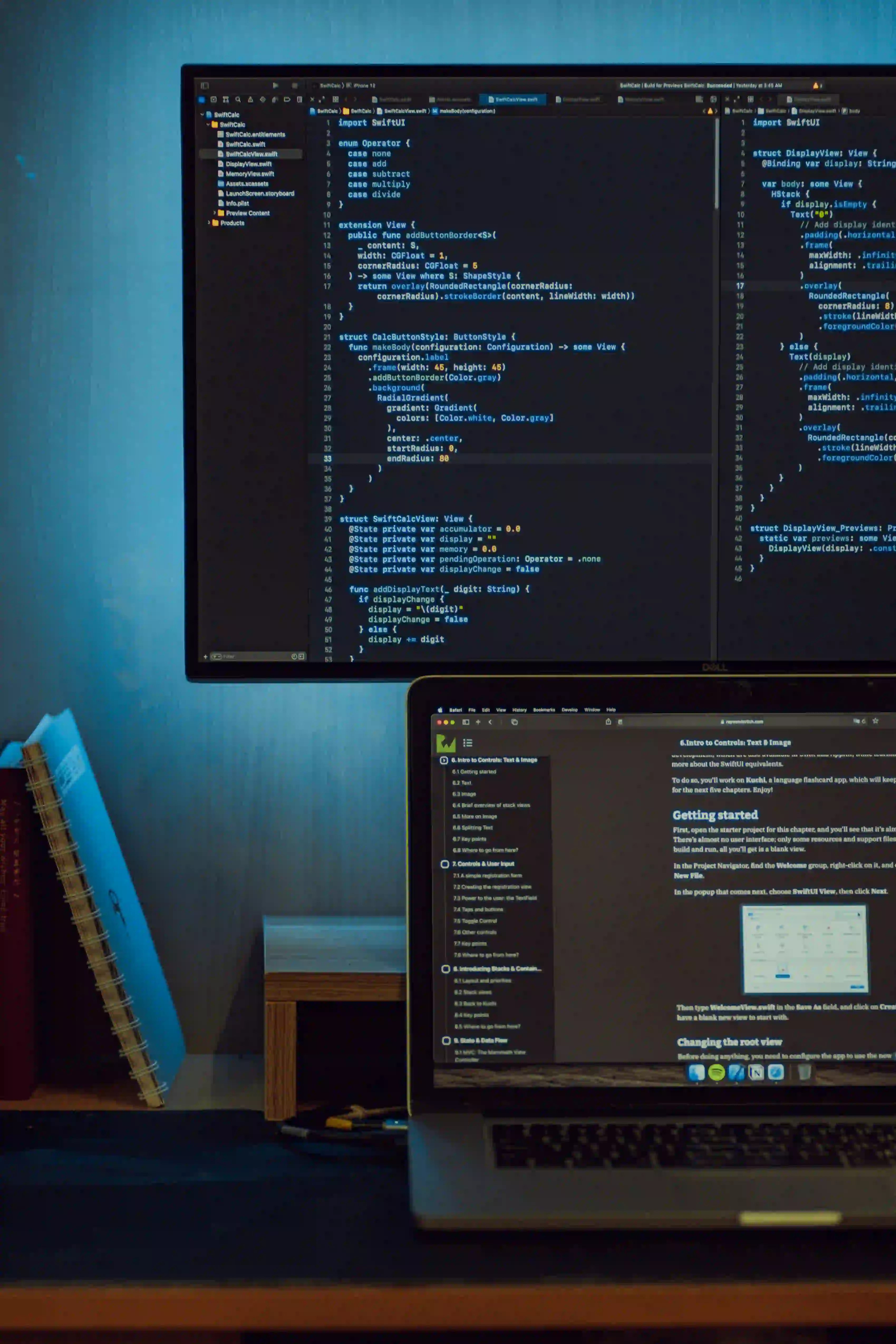
Struggling with Customization? Optimize Your Data Store Today!
In an increasingly data-driven world, the ability to efficiently customize and optimize your data storage methods is key to your application's performance. Java, a robust programming language, offers various libraries and frameworks to help developers manage data effectively. This blog post will walk you through several customization strategies that can enhance your data store, ensuring optimal performance and a better user experience.
Understanding the Basics of Data Storage
Before diving into customization techniques, it’s crucial to understand the different types of data storage available in Java. Generally, data can be stored in:
- In-memory Databases: Fast and efficient, but not suited for persistent storage.
- Relational Databases: Good for structured data but can become complex with large datasets.
- NoSQL Databases: Flexible schemas and scalability, but might lack consistency.
- File Systems: Suitable for storing large unstructured data.
You will soon recognize that the choice of data storage solution is paramount, depending on your specific needs.
Essentials at a Glance to Java Data Store Optimization
Optimization begins with understanding what customization options exist. Java provides several libraries and frameworks, such as Hibernate and JPA (Java Persistence API), to facilitate data management. Here’s a breakdown of various options to consider:
1. Utilize Proper Indexing
Indexing is critical for enhancing query performance, especially with relational databases. By creating indexes on frequently queried columns, you can drastically reduce the data lookup time.
Example Code Snippet
CREATE INDEX idx_user_email ON users (email);
Why It Works: This SQL command creates an index on the email
column of the users
table. Search queries involving the email field will return results faster, thereby improving overall performance.
2. Efficient Data Retrieval
Use JPA
to streamline data retrieval in your Java application. Fetching large amounts of data can consume memory and slow down performance. Implementing pagination can improve efficiency.
Example Code Snippet
TypedQuery<User> query = entityManager.createQuery("SELECT u FROM User u", User.class);
query.setFirstResult(page * size);
query.setMaxResults(size);
List<User> users = query.getResultList();
Why It Works: The above code snippet limits the number of records retrieved at once. Paginating responses significantly reduces memory overhead, making the application more scalable.
Learn more about JPA here.
3. Cache Frequently Accessed Data
To further enhance performance, consider implementing a caching strategy. Frameworks like EHCache or Spring Cache can store frequently accessed data in memory, reducing the need for repeated database queries.
Example Code Snippet
@Cacheable("usersCache")
public User findUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
Why It Works: By caching user data, subsequent calls for the same user ID fetch information from the cache rather than querying the database, significantly speeding up access time.
Discover more about caching strategies in Spring here.
4. Optimize Your SQL Queries
Writing efficient SQL queries is essential for optimization. Ensure that your queries are well-structured to avoid full table scans.
Example Code Snippet
SELECT name, email FROM users WHERE status = 'active';
Why It Works: This query selects only the fields needed for active users, reducing the amount of data processed and sent over the network. Always aim to reduce the volume of retrieved data.
5. Handle Transactions Wisely
Managing database transactions is vital for maintaining data integrity and performance. Use appropriate isolation levels to balance consistency and performance.
Example Code Snippet
EntityTransaction transaction = entityManager.getTransaction();
try {
transaction.begin();
// Perform operations
transaction.commit();
} catch (RuntimeException e) {
transaction.rollback();
throw e; // or handle it
}
Why It Works: This code demonstrates a best practice in handling transactions. By rolling back transactions on failure, you maintain data integrity while still ensuring that your application runs efficiently.
Advanced Techniques
Once you have addressed the basics, you can explore advanced optimization techniques. These strategies vary based on the scale of your application and data complexity.
6. Asynchronous Processing
If your application can handle it, consider asynchronous processing. APIs can respond quickly while handling data save or retrieval in the background.
Example Code Snippet
@Async
public CompletableFuture<User> findUserAsync(Long id) {
return CompletableFuture.completedFuture(userRepository.findById(id).orElse(null));
}
Why It Works: By making the findUserAsync
method asynchronous, users receive a response immediately without waiting for the data retrieval process to complete.
Read more about asynchronous processing in Spring here.
7. Monitor Performance
Last but not least, consistently monitor your application’s performance. Tools such as Java Mission Control, JProfiler, or YourKit can help analyze where your application may be lagging.
Example Monitoring Code
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
@Aspect
public class LoggingAspect {
@Before("execution(* com.example.service..*(..))")
public void logBeforeService() {
System.out.println("Service method called");
}
}
Why It Works: By logging service method calls, you can gain insights into your application’s performance, identifying bottlenecks and enhancing user experience.
Key Takeaways
Optimizing your data store is no small feat, but the effort is certainly worth it. Through effective customization strategies such as utilizing proper indexing, efficient data retrieval, caching, SQL query optimization, managing transactions wisely, and integrating asynchronous processing, you can significantly enhance your data store’s performance.
Remember, performance monitoring is also an essential aspect of optimization. It ensures that you can proactively address any issues before they escalate.
This guide should serve as a launchpad for your data optimization journey in Java. For additional resources, don’t forget to explore Hibernate, Spring Data, and the official Java Documentation.
As you implement these strategies, may your Java applications thrive with performance! Happy coding!