Troubleshooting HTML to RichTextString in Apache POI
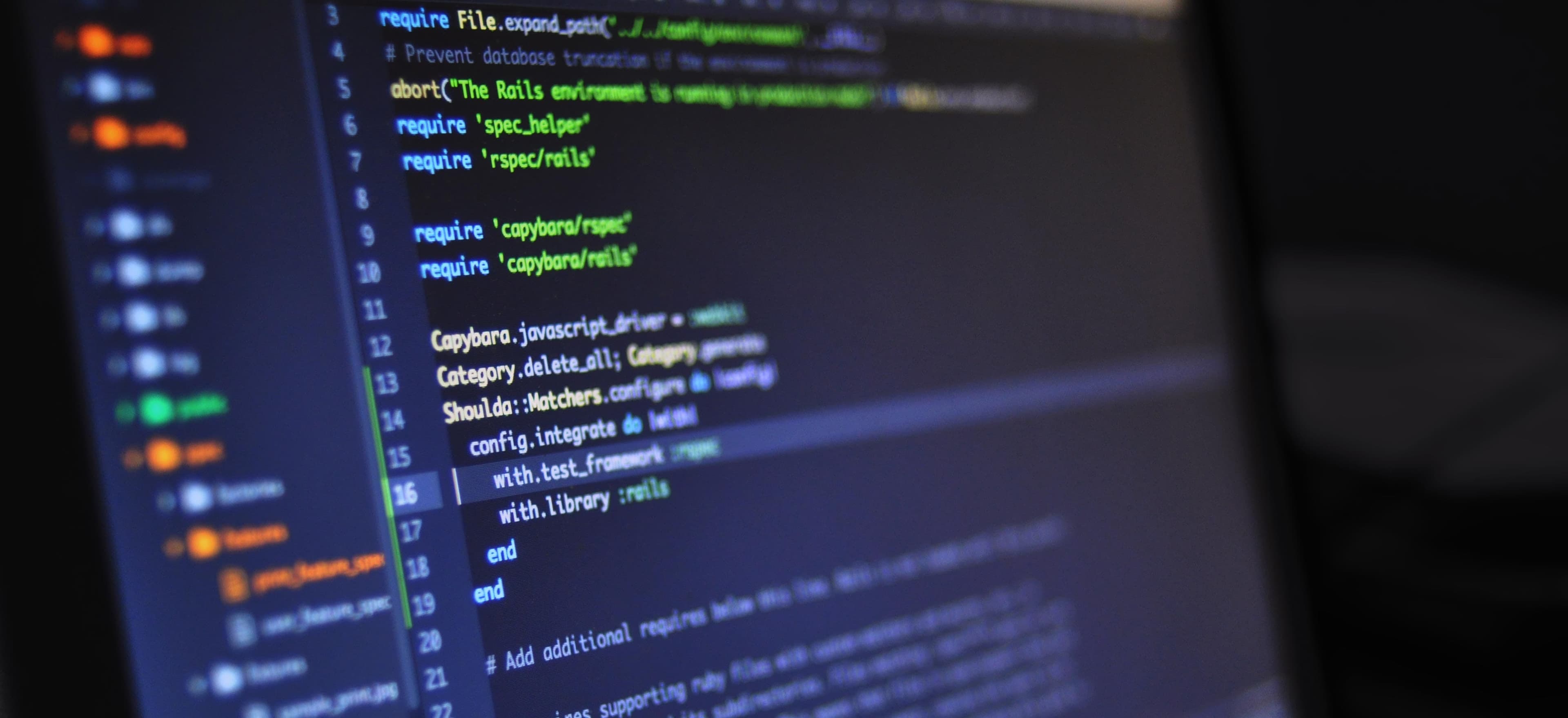
- Published on
Troubleshooting HTML to RichTextString in Apache POI
Apache POI is a powerful Java library for manipulating various file formats based on the Office Open XML standards (e.g., .xlsx) and Microsoft OLE 2 Compound Document format (e.g., .xls). One common requirement developers face is converting HTML content to a format that can be used in Excel cells, such as RichTextString
. This post covers the necessary steps, potential pitfalls, and troubleshooting techniques when working with HTML to RichTextString
conversion in Apache POI.
Understanding RichTextString
Before diving into HTML conversion, it’s important to understand what RichTextString
is. This is an interface in Apache POI that represents a string that can have different styles applied to it.
Why Use RichTextString?
- Formatted Text: Enables the use of different fonts, colors, and styles within a single cell.
- Improved Presentation: Enhances the look of the spreadsheet cells, making it easier to read, especially when dealing with complex data.
Converting HTML to RichTextString
To convert HTML to RichTextString
, you generally need a two-step approach:
- Parse the HTML content.
- Create a
RichTextString
that represents the parsed content.
Setting Up Your Environment
You'll first need to include Apache POI in your Java project. If you are using Maven, add the following dependency:
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.2.3</version> <!-- Check for the latest version -->
</dependency>
Sample Code for HTML to RichTextString Conversion
Here’s a simple code snippet that demonstrates converting HTML into a RichTextString
:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileOutputStream;
import java.io.IOException;
public class HtmlToRichText {
public static void main(String[] args) {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sample");
String htmlContent = "<b>Hello</b> <i>World</i>";
RichTextString richText = createRichTextFromHtml(workbook, htmlContent);
Row row = sheet.createRow(0);
Cell cell = row.createCell(0);
cell.setCellValue(richText);
try (FileOutputStream fileOut = new FileOutputStream("output.xlsx")) {
workbook.write(fileOut);
} catch (IOException e) {
e.printStackTrace();
}
}
public static RichTextString createRichTextFromHtml(Workbook workbook, String html) {
RichTextString richText = workbook.getCreationHelper().createRichTextString("");
// Parse HTML and build the RichTextString
String[] segments = html.split(" ");
for (String segment : segments) {
String trimmedSegment = segment.replaceAll("<[^>]*>", ""); // Remove HTML tags
int startIndex = html.indexOf(segment);
int endIndex = startIndex + trimmedSegment.length();
// Apply formatting based on HTML tags
if (segment.startsWith("<b>")) {
richText.append(trimmedSegment, workbook.createCellStyle().setBold(true));
} else if (segment.startsWith("<i>")) {
richText.append(trimmedSegment, workbook.createCellStyle().setItalic(true));
} else {
richText.append(trimmedSegment);
}
}
return richText;
}
}
Code Explanation
- Workbook Creation: Initializes a workbook using
XSSFWorkbook
. - HTML Content: This sample specifies a small snippet of HTML.
- Creating the Cell: Sets up the spreadsheet structure—creating a row and a cell.
- Parsing HTML: A basic parser is implemented. It splits the HTML by spaces, replaces HTML tags, and applies formatting styles based on tag types.
- Writing to File: Finally, it writes the workbook to an Excel file called output.xlsx.
Alternative Approach: Using Jsoup
For more complex HTML, consider using Jsoup, a powerful HTML parser for Java. This will significantly simplify and enhance your HTML parsing capabilities.
Example with Jsoup
Here’s a more complete example using Jsoup that helps manage various HTML tags efficiently:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import java.io.FileOutputStream;
import java.io.IOException;
public class HtmlToRichTextUsingJsoup {
public static void main(String[] args) {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sample with Jsoup");
String htmlContent = "<b>Hello</b> <i>World</i>";
RichTextString richText = createRichTextFromHtml(workbook, htmlContent);
Row row = sheet.createRow(0);
Cell cell = row.createCell(0);
cell.setCellValue(richText);
try (FileOutputStream fileOut = new FileOutputStream("output_jsoup.xlsx")) {
workbook.write(fileOut);
} catch (IOException e) {
e.printStackTrace();
}
}
public static RichTextString createRichTextFromHtml(Workbook workbook, String html) {
RichTextString richText = workbook.getCreationHelper().createRichTextString("");
Document doc = Jsoup.parse(html);
for (Element element : doc.body().children()) {
String text = element.ownText();
int startIndex = richText.length();
// Apply styling based on the HTML tag
if (element.tagName().equals("b")) {
richText.append(text, workbook.createCellStyle().setBold(true));
} else if (element.tagName().equals("i")) {
richText.append(text, workbook.createCellStyle().setItalic(true));
} else {
richText.append(text);
}
}
return richText;
}
}
Reasons to Use Jsoup
- Robust Parsing: jsoup can handle complex HTML, deeply nested elements, and various HTML attributes.
- Flexibility: You can manipulate the HTML structure before conversion.
Common Issues and Troubleshooting
1. HTML Parsing Challenges
When handling HTML directly, the parser may misinterpret certain elements or attributes. It’s essential to validate your HTML to avoid such issues. Use an HTML validator tool if necessary.
2. Styling Not Applying
Ensure proper styles were created and applied. If styles do not seem to apply, double-check the conditions under which styles are applied in the code.
3. Unsupported HTML Tags
Not all HTML tags will translate into Excel formatting. Keep your HTML simple and standard.
4. Performance Concerns
If working with large HTML content, performance may degrade. Optimize your parsing logic and consider breaking down your HTML into manageable chunks.
My Closing Thoughts on the Matter
Converting HTML to RichTextString
using Apache POI provides an effective way to bring formatted text into Excel. By combining POI with tools like Jsoup, you can create sophisticated documents efficiently. Make sure to handle troubleshooting with precision, and validate your HTML for optimal results.
For further reading on Apache POI functionalities, check out the Apache POI Documentation or explore Jsoup's Official Site to dive deeper into HTML parsing.
With this guide, you're well-equipped to tackle HTML conversion challenges and enhance your Excel files programmatically!