7 Key Questions to Perfect Your Code Quality
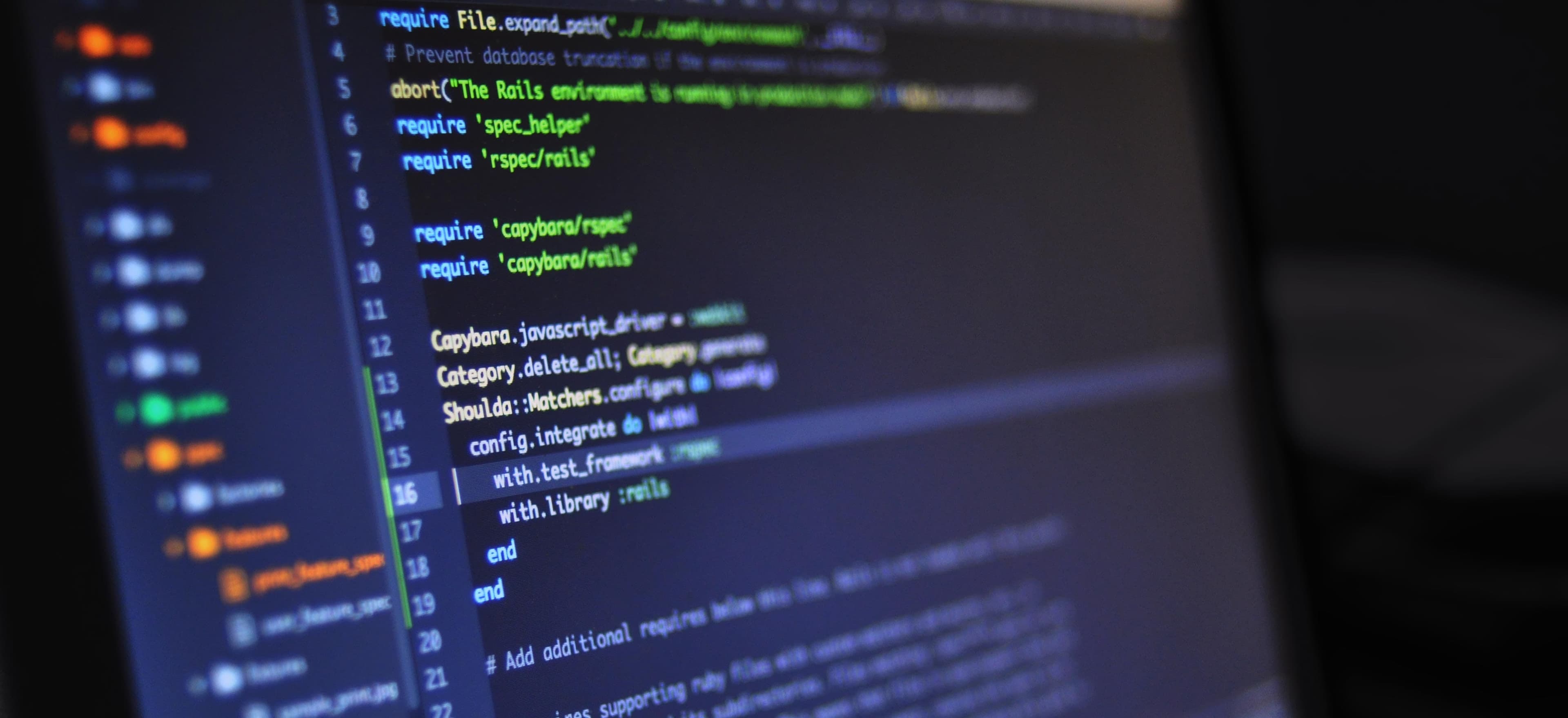
- Published on
7 Key Questions to Perfect Your Code Quality
In the world of software development, code quality holds immense significance. High-quality code is not only crucial for maintaining and scaling applications, but it also enhances collaboration among developers. Poor code quality leads to increased costs and time spent on debugging and refactoring, which can hinder project timelines and team morale. In this blog post, we’ll explore seven key questions that will help you evaluate and perfect your code quality.
The Importance of Code Quality
Before diving into the questions, it’s essential to understand why code quality matters. Code quality directly impacts the maintainability, readability, and performance of the software. Quality code reduces the risk of defects, hence lowering the cost of maintenance and improving overall system performance.
The process of writing quality code can be guided by several factors, including best practices, design patterns, and continuous integration processes. To help you with your journey toward impeccable code quality, let’s talk about the seven key questions to ask yourself.
1. Is My Code Readable?
Why It Matters
Readability is vital because readable code can significantly reduce the time it takes for a new developer to understand the codebase. When code is easy to read, it minimizes confusion and makes it easier to spot bugs.
What to Consider
- Naming Conventions: Are your variables and method names descriptive?
- Comments: Are you using comments effectively to explain why you implemented certain logic?
Example
// Good naming
public class Product {
private String productName;
public String getProductName() {
return productName;
}
// Poor naming
public String getN() {
return productName;
}
}
In this example, the getProductName()
method is much clearer than getN()
. Always strive for clarity in naming.
2. Is My Code Efficient?
Why It Matters
Efficiency can significantly impact an application's performance. Code that runs slowly can lead to poor user experiences and wasted resources.
What to Consider
- Algorithm Complexity: Are you aware of your algorithms' time and space complexity?
- Data Structures: Are you using the right data structures for your needs?
Example
// Using ArrayList for random access is efficient
List<Integer> numbers = new ArrayList<>();
for (int i = 0; i < n; i++) {
numbers.add(i);
}
// Inefficient for sorted data - should use a LinkedList
int firstElement = numbers.get(0);
Using an ArrayList
when random access is needed is a better choice than a LinkedList
.
3. Does My Code Follow Best Practices?
Why It Matters
Adhering to industry best practices ensures that your code is maintainable and reduces technical debt. Following guidelines, such as SOLID principles, can lead to better-structured code.
What to Consider
- SOLID Principles: Are you adhering to SOLID principles in your object-oriented design?
- DRY Principle: Are you avoiding code duplication?
Example
// Following the Single Responsibility Principle
public class Order {
private List<Item> items;
public void addItem(Item item) {
items.add(item);
}
public void calculateTotal() {
// Calculate total
}
}
// Poor example: violating SRP
public class Order {
// Mixing responsibilities
public void addItem(Item item) {}
public void calculateTotal() {}
public void printOrder() {}
}
By ensuring that each class has a single responsibility, you improve the maintainability of your code.
4. Is My Code Testable?
Why It Matters
Code that is difficult to test is often a sign of poor design. Writing unit tests is crucial for ensuring that your code works as expected and can easily be refactored in the future.
What to Consider
- Separation of Concerns: Are your classes and methods isolated enough to be tested independently?
- Mocking Dependencies: Are you using mocking frameworks properly?
Example
// Dependency Injection for Testability
public class UserService {
private UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUser(String id) {
return userRepository.findById(id);
}
}
// Unit Test
public class UserServiceTest {
private UserService userService;
private UserRepository userRepository;
@Before
public void setUp() {
userRepository = mock(UserRepository.class);
userService = new UserService(userRepository);
}
}
By using dependency injection, you can easily mock the UserRepository
for unit testing.
5. Have I Considered Scalability?
Why It Matters
Scalability allows your application to handle growth effectively. If your code isn't scalable, you will run into performance bottlenecks as user demand increases.
What to Consider
- Load Testing: Have you tested your application under conditions that mimic real-world usage?
- Horizontal vs. Vertical Scaling: Are you aware of the differences and how they apply to your architecture?
Example
// Basic example of scalability option
public void processOrders(List<Order> orders) {
for (Order order : orders) {
// Handle each order
}
}
// Scalable approach using parallel streams
orders.parallelStream().forEach(order -> handleOrder(order));
Using parallel streams can enhance performance in scenarios with large datasets.
6. Is My Code Secure?
Why It Matters
Security breaches can lead to data loss, reputational damage, and financial costs. Secure code practices help identify vulnerabilities early in development.
What to Consider
- Input Validation: Are you validating all inputs?
- Data Encryption: Are sensitive data properly encrypted?
Example
// Input validation example
public String processInput(String input) {
if (input == null || input.trim().isEmpty()) {
throw new IllegalArgumentException("Invalid input");
}
return input.trim();
}
Proper validation helps in preventing common security issues such as SQL Injection and Cross-Site Scripting (XSS).
7. Is My Code Well-Documented?
Why It Matters
Good documentation makes code easier to understand and maintain. It assists current and future developers in navigating the codebase effectively.
What to Consider
- Inline Comments: Are they used judiciously?
- External Documentation: Do you have comprehensive documentation for your API?
Example
/**
* Calculates the total price of the order
* @return total price
*/
public double calculateTotal() {
// Calculate total price based on items in the order
return items.stream().mapToDouble(Item::getPrice).sum();
}
Clear documentation is essential for helping others (or your future self) quickly understand the code's purpose.
The Bottom Line
By asking yourself these seven key questions about your code quality, you'll be well-equipped to enhance its readability, efficiency, maintainability, and security. Regularly reflecting on these areas not only improves your current projects but also instills best practices that promise better software development in the long run.
If you're looking for more ways to improve your Java skills, consider checking out resources like Java Code Geeks or the Official Java Documentation for further insights. Remember, the road to perfection is paved with continuous learning and improvement. Happy coding!