Overcoming Migration Challenges in Jakarta EE Clean Slate
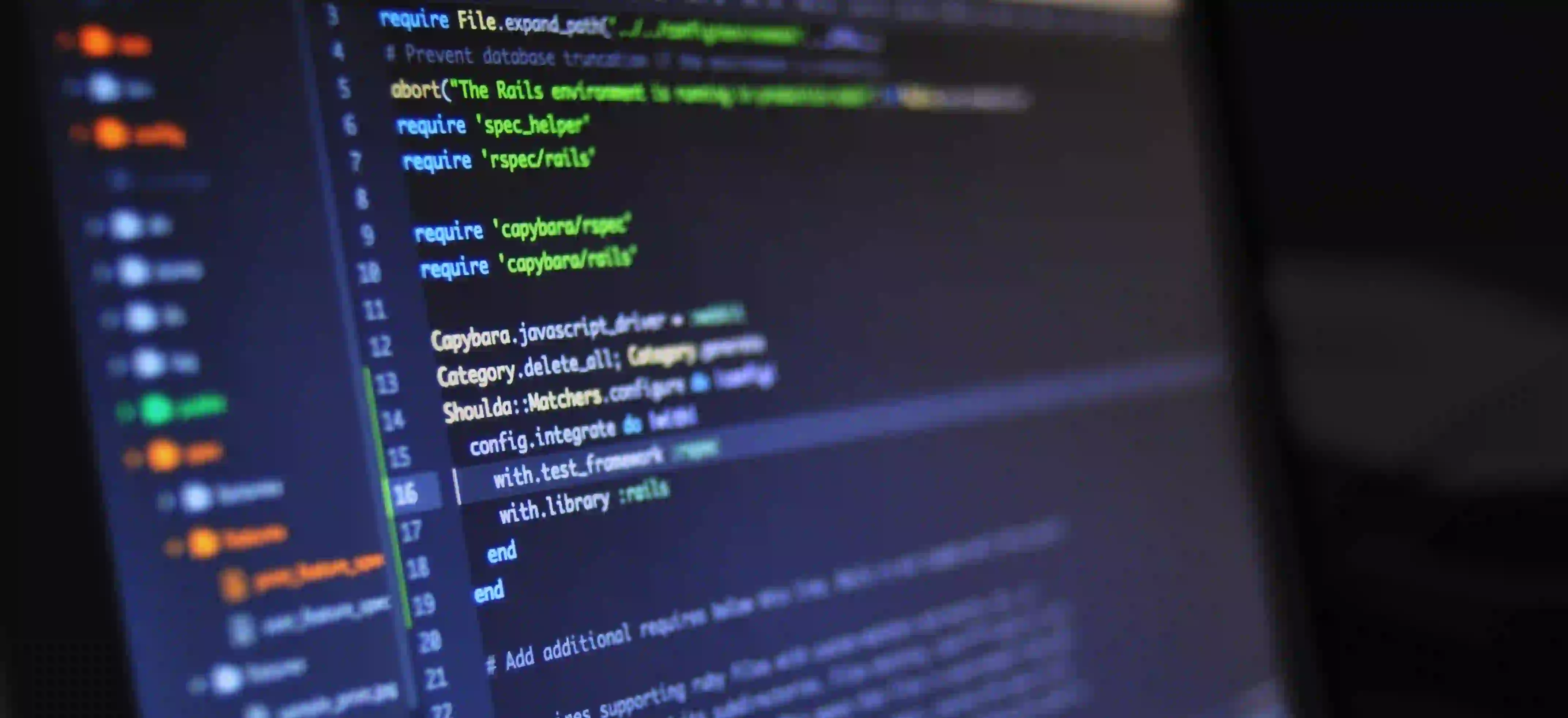
Overcoming Migration Challenges in Jakarta EE Clean Slate
In the constantly evolving world of software development, transitioning from one platform to another can often feel like navigating a minefield. Jakarta EE, formerly known as Java EE, is a promising framework for developing enterprise applications, but migrating to a clean slate can pose significant challenges. In this blog post, we will explore how to overcome these challenges when migrating your applications to Jakarta EE, leveraging best practices, effective strategies, and real-world code examples.
Table of Contents
- Understanding Jakarta EE
- Common Migration Challenges
- Strategies to Overcome Migration Challenges
- Example Migration Steps
- Conclusion
Understanding Jakarta EE
Jakarta EE is the new manifestation of Java EE under the Eclipse Foundation. It aims to provide a robust and scalable framework for enterprise applications while remaining open source. With its modern specifications, Jakarta EE offers enhancements that significantly improve developer productivity and application performance.
Key Features of Jakarta EE
- Microservices support: With the implementation of MicroProfile, Jakarta EE simplifies the development of microservices.
- Dependency injection: This makes your code more modular and easier to manage.
- Reactive programming support: Building responsive applications has never been easier.
For those interested, you can dive deeper into the Jakarta EE Documentation.
Common Migration Challenges
-
Legacy Code Compatibility: One of the most daunting challenges is ensuring that legacy systems operate seamlessly with the new Jakarta EE architecture.
-
API changes: Transitioning to Jakarta EE involves dealing with updated APIs, renaming of packages, and removal of deprecated features.
-
Dependency Management: Managing dependencies in a clean slate environment can become complex during migration.
-
Skill Gap: Development teams accustomed to Java EE may require training to become proficient in Jakarta EE.
-
Testing and Quality Assurance: Ensuring that the new system operates as expected can be tricky, particularly if the legacy system had insufficient tests.
Strategies to Overcome Migration Challenges
1. Assessment and Planning
Before jumping into migration, conducting a thorough assessment of your existing application is vital.
- Code Review: Analyze the legacy codebase to identify areas that may require significant rewrites.
- Feature Inventory: List features in the legacy system that are essential, deprecated, or can be improved.
Having a clear plan helps smooth the transition to Jakarta EE.
2. Training and Resources
Ensure your development team understands the nuances of Jakarta EE:
- Workshops and Courses: Invest in training sessions dedicated to Jakarta EE. The Eclipse Foundation offers resources and workshops that can be beneficial.
- Documentation: Encourage developers to read up on the Jakarta EE specifications to become familiar with updates.
3. Incremental Migration
Breaking the migration into smaller, manageable tasks is an effective strategy:
- Modular Approach: Start by migrating less critical components and gradually work towards critical applications. This reduces the risk of downtime.
- Parallel Operation: Keep both the legacy and new systems operational until the latter is fully proven.
4. Utilize Jakarta EE Best Practices
Embrace best practices specific to Jakarta EE that can streamline the process.
- Use Dependency Injection: Make use of Contexts and Dependency Injection (CDI). Here’s a code snippet showcasing this:
@ApplicationScoped
public class UserService {
// Injecting dependency
@Inject
private UserRepository userRepository;
public User createUser(User user) {
// business logic
return userRepository.save(user);
}
}
In this code, CDI promotes a clean separation of concerns, ensuring that UserService
does not create its own instance of UserRepository
, leading to better testability and maintainability.
5. Testing as a First-Class Citizen
Making testing a priority cannot be overstated:
-
Automated Tests: Build a suite of automated tests that cover both unit and integration tests for the application. You can utilize tools like JUnit or Arquillian.
Here is an example of a simple JUnit test:
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class UserServiceTest {
private UserService userService = new UserService();
@Test
void testCreateUser() {
User user = new User("John", "Doe");
User createdUser = userService.createUser(user);
assertEquals("John", createdUser.getFirstName()); // Basic test for example
}
}
By incorporating testing into your migration plan, you will reduce the uncertainty around system reliability during and after the migration process.
Example Migration Steps
Let’s outline a practical example of migrating a simple application to Jakarta EE.
- Inventory Features: Begin by listing all features in your current application.
- Set Up Jakarta EE Environment: Install a Jakarta EE-compliant server (e.g., Payara, WildFly).
- Migrate Libraries: Update your build configuration (Maven/Gradle) to include Jakarta dependencies.
- Refactor Code: Begin refactoring code, starting with utility classes and services.
- Implement Unit Tests: Develop unit tests around the new code.
- Deploy and Validate: Deploy the application in a staging environment and validate against the original application’s behavior.
- Full Deployment: Once validated, proceed to full deployment.
Lessons Learned
Migrating to Jakarta EE can indeed be fraught with challenges, but with careful planning, a commitment to training, and a structured strategy, it can lead to higher productivity and more robust applications. Embracing best practices, incremental migration, and extensive testing solidify your efforts and minimize risks.
Ultimately, the move to Jakarta EE represents not just a technical upgrade but an opportunity to modernize how you approach development. Explore the possibilities, equip your team with knowledge, and unlock the full potential of enterprise applications.
For more insights and community discussions on Jakarta EE, check out the Jakarta EE Community.
By following these guidelines, you can navigate the complexities of a migration to Jakarta EE while ensuring that your applications remain effective, efficient, and ready for the challenges of tomorrow. Happy coding!