Mastering CRUD Validation in Java EE 7 and AngularJS
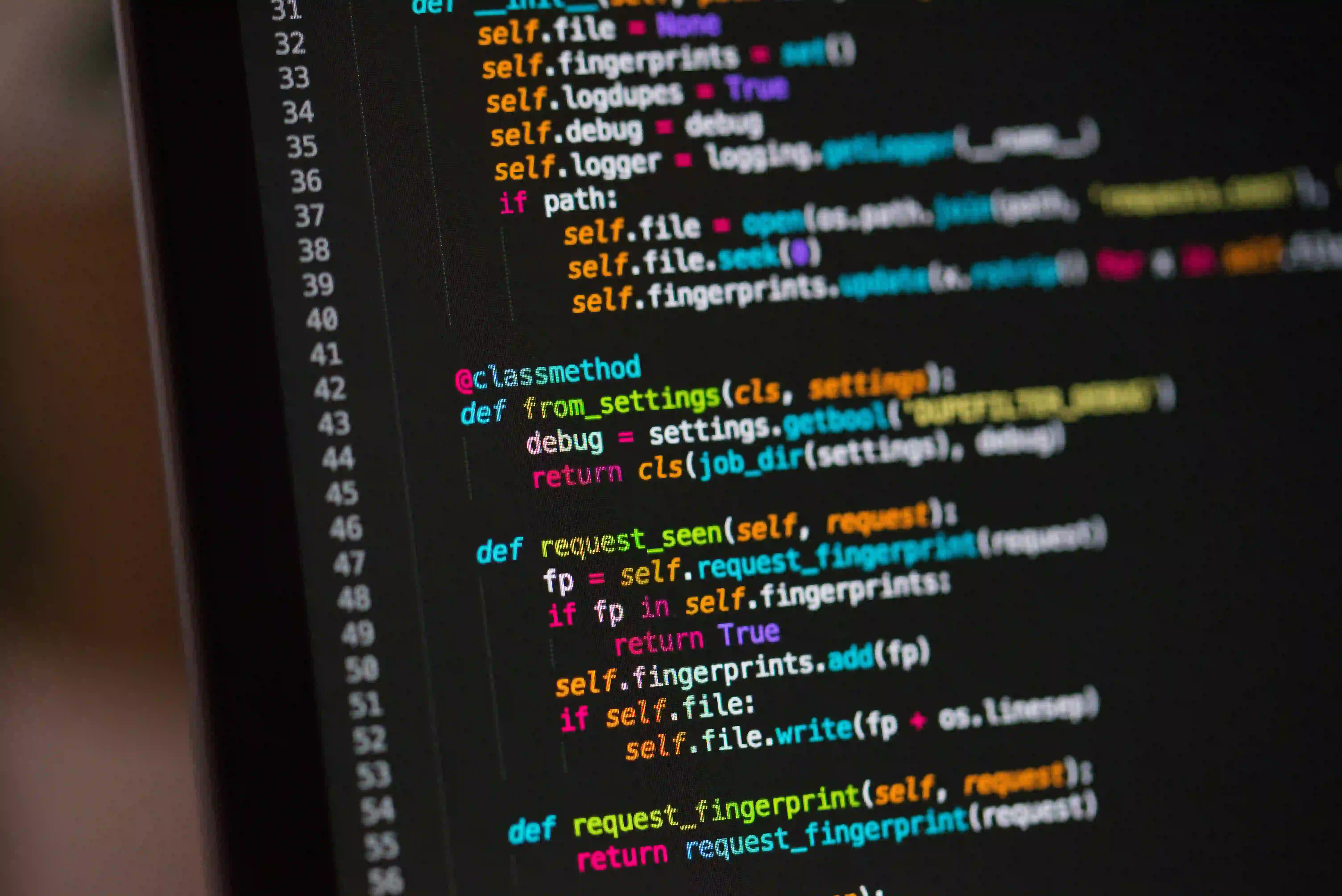
Mastering CRUD Validation in Java EE 7 and AngularJS
In the ever-evolving world of web applications, mastering Create, Read, Update, and Delete (CRUD) operations is crucial for any developer. This blog post will focus on how to implement effective validation on these operations using Java EE 7 as a backend and AngularJS for the front end. By the end, you should have a solid understanding of how to ensure your CRUD operations are reliable and secure.
Table of Contents
- Introduction
- CRUD Operations Overview
- Validation in Java EE 7
- Front-End Validation with AngularJS
- Integrating Front-End and Back-End Validation
- Conclusion
- Additional Resources
1. Introduction
CRUD operations form the backbone of web applications. They allow users to interact with data in meaningful ways. While these operations seem straightforward, ensuring data integrity through validation is paramount. Validation helps in maintaining the quality and security of your applications. In this post, we will delve into both backend validation using Java EE 7 and frontend validation using AngularJS.
2. CRUD Operations Overview
CRUD stands for:
- Create: Adding new records to the database.
- Read: Fetching records from the database.
- Update: Modifying existing records.
- Delete: Removing records from the database.
Each of these operations requires robust validation to ensure that the data being processed is accurate and secure.
3. Validation in Java EE 7
Java EE 7 provides a robust framework for backend validation. The Bean Validation API offers a way to enforce validation rules directly on the data models. Here’s how to implement basic validation for a user entity:
User Entity Example
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
public class User {
@NotNull(message = "Username cannot be null")
@Size(min = 3, max = 30, message = "Username must be between 3 and 30 characters")
private String username;
@NotNull(message = "Email cannot be null")
@Email(message = "Must be a valid email")
private String email;
// Getters and Setters omitted for brevity
}
Commentary on the Code
-
Annotations: Here, we use
@NotNull
to ensure that the username and email are provided. The@Size
annotation restricts the username length. Any request with invalid data will throw a validation error, enhancing data integrity upfront. -
Control: By placing validation directly on the entity class, any time this entity is instantiated, you can ensure that the values conform to the rules specified.
Creating a REST Endpoint with Validation
Now, let’s integrate this user entity into a RESTful service:
import javax.ejb.Stateless;
import javax.validation.Valid;
import javax.ws.rs.Consumes;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.core.Response;
@Stateless
@Path("/users")
public class UserService {
@POST
@Consumes("application/json")
public Response createUser(@Valid User user) {
// Logic to save the user to the database
return Response.status(Response.Status.CREATED).build();
}
}
Explanation
- RESTful Endpoint: The
@POST
method will consume JSON data to create a new user. - @Valid: At the method parameter level,
@Valid
ensures that the user data coming through this endpoint is validated before any business logic is executed.
4. Front-End Validation with AngularJS
AngularJS provides powerful form validation capabilities out of the box. Using built-in directives, you can implement various validation checks directly in the frontend. Let’s see how to set this up for our User
form.
AngularJS User Form Example
<form name="userForm" novalidate ng-submit="submitForm(userForm)">
<div>
<label for="username">Username</label>
<input type="text" ng-model="user.username" name="username" required minlength="3" maxlength="30" />
<span ng-show="userForm.username.$error.required">Username is required.</span>
<span ng-show="userForm.username.$error.minlength">Username must be at least 3 characters long.</span>
<span ng-show="userForm.username.$error.maxlength">Username cannot exceed 30 characters.</span>
</div>
<div>
<label for="email">Email</label>
<input type="email" ng-model="user.email" name="email" required />
<span ng-show="userForm.email.$error.required">Email is required.</span>
<span ng-show="userForm.email.$error.email">Must be a valid email.</span>
</div>
<button type="submit" ng-disabled="userForm.$invalid">Create User</button>
</form>
Breakdown of the Code
- ngModel: Binds the input fields to the
user
object in the AngularJS scope. - Validation Messages: Angular allows for easy feedback through the
$error
object, which automatically validates based on defined criteria likerequired
,minlength
, andmaxlength
. - Submit Button: Using
ng-disabled
, the submit button gets automatically disabled if the form doesn't satisfy validation.
5. Integrating Front-End and Back-End Validation
Even with robust validations in place, relying solely on front-end validation can expose your application to security issues like JavaScript injection or bypass. It is crucial to ensure that backend validation complements front-end validation.
How to Handle Responses
In the AngularJS controller, you can implement logic to handle validation messages returned by the backend:
$scope.submitForm = function(form) {
if (form.$valid) {
$http.post('/api/users', $scope.user).then(
function(response) {
// Handle success
},
function(response) {
// Handle error
if (response.status === 400) {
$scope.errors = response.data.errors; // Assuming backend returns a structured error response
}
}
);
}
};
Explanation
- Error Handling: On submitting the form, if there are validation errors from the backend, they are captured in the
$scope.errors
variable, which can then be displayed to the user.
6. Conclusion
In this post, we explored how to implement CRUD validation using Java EE 7 and AngularJS. We examined the importance of validation not only for maintaining data integrity but also for ensuring the security of your application.
- Backend Validation: Use the Bean Validation API to enforce rules on your data models.
- Frontend Validation: Leverage AngularJS for a seamless user experience and immediate feedback.
- Integration: Ensure that both validations work in tandem to provide strong security and data integrity.
Validation is often an overlooked aspect of application development, but by understanding and implementing it properly, you can drastically improve the reliability of your applications.
7. Additional Resources
- Java EE Documentation
- AngularJS Documentation
- Bean Validation Specification
- RESTful Web Services Tutorial
By fully grasping these concepts, you’ll be well on your way to mastering CRUD validation in modern web applications!