Scaling Jenkins on AWS: Avoiding Common Pitfalls
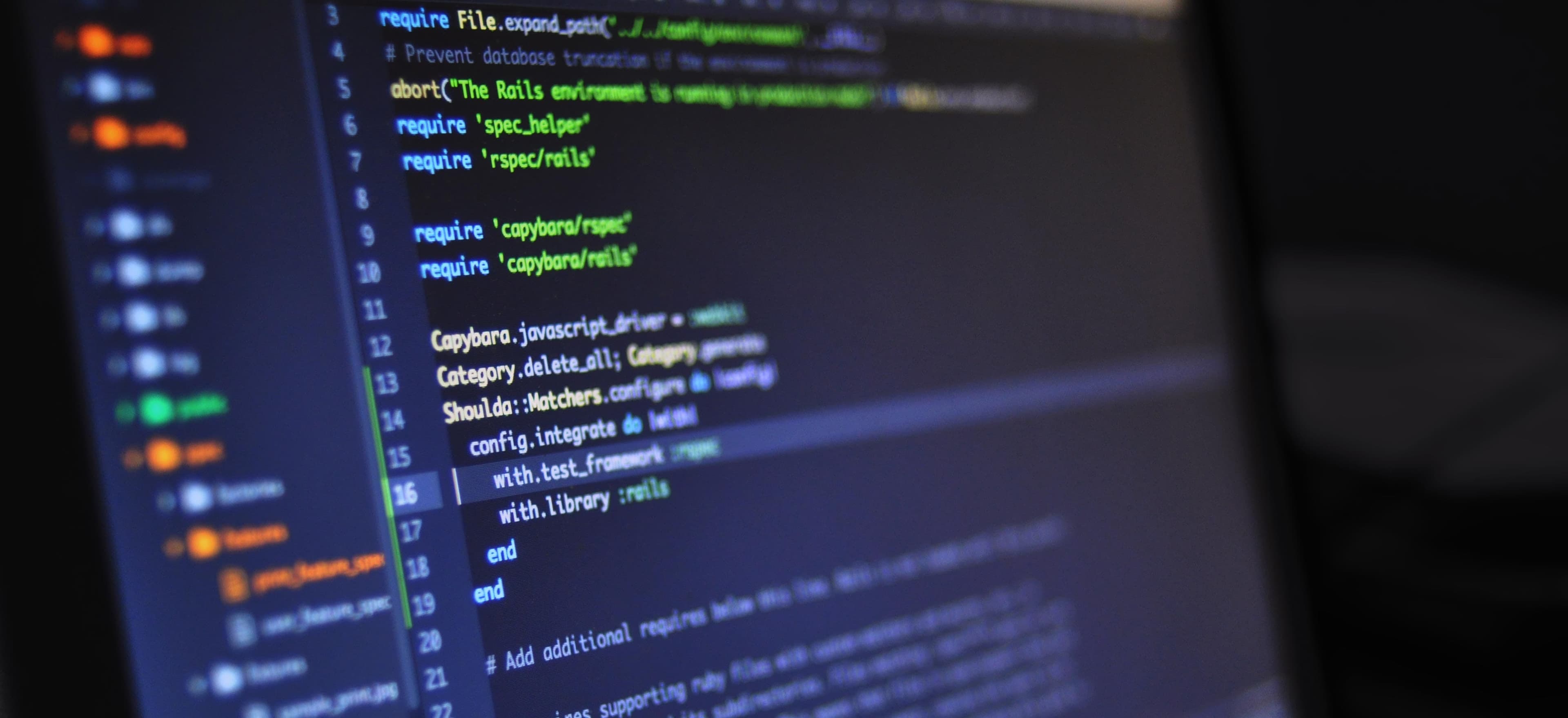
- Published on
Scaling Jenkins on AWS: Avoiding Common Pitfalls
Jenkins is one of the most popular open-source automation servers for continuous integration and continuous delivery (CI/CD). As your team grows in size and your project scales, you might find the need to scale Jenkins efficiently on Amazon Web Services (AWS). However, there are common pitfalls that teams often encounter while trying to scale. This blog post will guide you through these pitfalls while offering practical solutions and insightful code snippets.
Understanding Jenkins Architecture
Before diving into the scaling process, let’s understand the fundamental components of Jenkins. Jenkins operates under a master-agent architecture:
- Master: Orchestrates the execution of builds and serves the Jenkins interface.
- Agents: Execute the build tasks that the master assigns. Agents can be run on diverse platforms, making it highly flexible.
When scaling Jenkins, you essentially need to manage these components effectively to distribute your workload.
Why Scale Jenkins?
Scaling Jenkins provides multiple benefits:
- Increased Build Capacity: More tasks can be executed simultaneously.
- Reduced Build Time: Faster turnarounds lead to increased productivity.
- Improved Reliability: With a distributed setup, you mitigate the risks of a single point of failure.
Common Jenkins Scaling Pitfalls
1. Lack of Instance Right-Sizing
One of the most frequent mistakes is choosing the wrong instance type. Companies often opt for default configurations, leading to either underperformance or overspending.
Solution: Right-Size Your Instances
Utilize AWS's EC2's instance types that best match your build requirements. For instance, if your builds are CPU-intensive, consider using compute-optimized instance types like C5 or C6g.
# Example: Launching a C5 instance using AWS CLI
aws ec2 run-instances --image-id ami-0abcdef1234567890 --count 1 --instance-type c5.large --key-name MyKeyPair --security-groups MySecurityGroup
Why: Right-sizing ensures that you don't overpay for resources you don't utilize while still providing sufficient power for your builds.
2. No Automated Scaling
Scaling Jenkins without automation can lead to bottlenecks. A static configuration will struggle to keep up with demand.
Solution: Implement Auto Scaling Groups
Employ EC2 Auto Scaling to adjust the number of active agents based on demand.
{
"Version": "2012-10-17",
"Statement": [
{
"Action": "autoscaling:UpdateAutoScalingGroup",
"Effect": "Allow",
"Resource": "*"
}
]
}
Why: Auto-scaling allows Jenkins to dynamically allocate resources as workloads fluctuate, optimizing performance and cost.
3. Insufficient Storage Management
Another common pitfall is neglecting to manage disk space effectively. Jenkins builds can quickly consume available storage, leading to build failures.
Solution: Utilize S3 for Artifact Storage
By moving artifacts to Amazon S3, you can free up local disk space.
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'gradle build'
}
}
stage('Archive') {
steps {
script {
def artifactPath = 'build/libs/*.jar'
def s3Bucket = 'my-artifact-bucket'
sh "aws s3 cp ${artifactPath} s3://${s3Bucket}/"
}
}
}
}
}
Why: Storing artifacts externally keeps your Jenkins instance lean and focused on executing builds, ultimately enhancing reliability.
4. Ignoring Load Distribution
Without efficient load distribution, you may experience slower builds and longer wait times.
Solution: Use Jenkins' Built-in Load Balancing
Configure Jenkins to distribute builds across multiple agents efficiently. This requires setting up your agents properly.
agent {
label 'linux && docker'
}
Why: Properly labeled agents ensure the correct builds run on compatible infrastructure, speeding up the CI/CD pipeline.
5. Neglecting Security Best Practices
Failing to configure security properly can expose your Jenkins instance to various vulnerabilities.
Solution: Implement IAM Roles
Using AWS Identity and Access Management (IAM), you can enforce the principle of least privilege for your Jenkins agents.
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": ["s3:GetObject", "s3:PutObject"],
"Resource": "arn:aws:s3:::my-artifact-bucket/*"
}
]
}
Why: IAM roles limit access, ensuring only necessary permissions are granted and enhancing the overall security of the Jenkins environment.
Additional Considerations
-
Backup and Restore: Implement regular backup solutions for your Jenkins configurations and jobs. Use Amazon RDS or EFS for Jenkins home directories.
-
Monitoring: Tools like AWS CloudWatch and Grafana can aid in monitoring Jenkins' performance, helping you quickly identify and address issues.
In Conclusion, Here is What Matters
Scaling Jenkins on AWS can be a daunting task, but by avoiding common pitfalls, your experience can be smooth and rewarding.
Remember to right-size instances, automate scaling, manage storage, distribute loads efficiently, and enforce security best practices.
For more on Jenkins architecture, visit Jenkins Official Documentation. To explore AWS EC2 instances and pricing, check AWS EC2 pricing page.
By keeping these strategies in mind, you’ll not only scale Jenkins effectively but also improve the productivity of your development team. Happy scaling!
References
Feel free to share your own experiences with scaling Jenkins in the comments below!
Checkout our other articles