Troubleshooting Hazelcast Configuration in Hibernate Caching
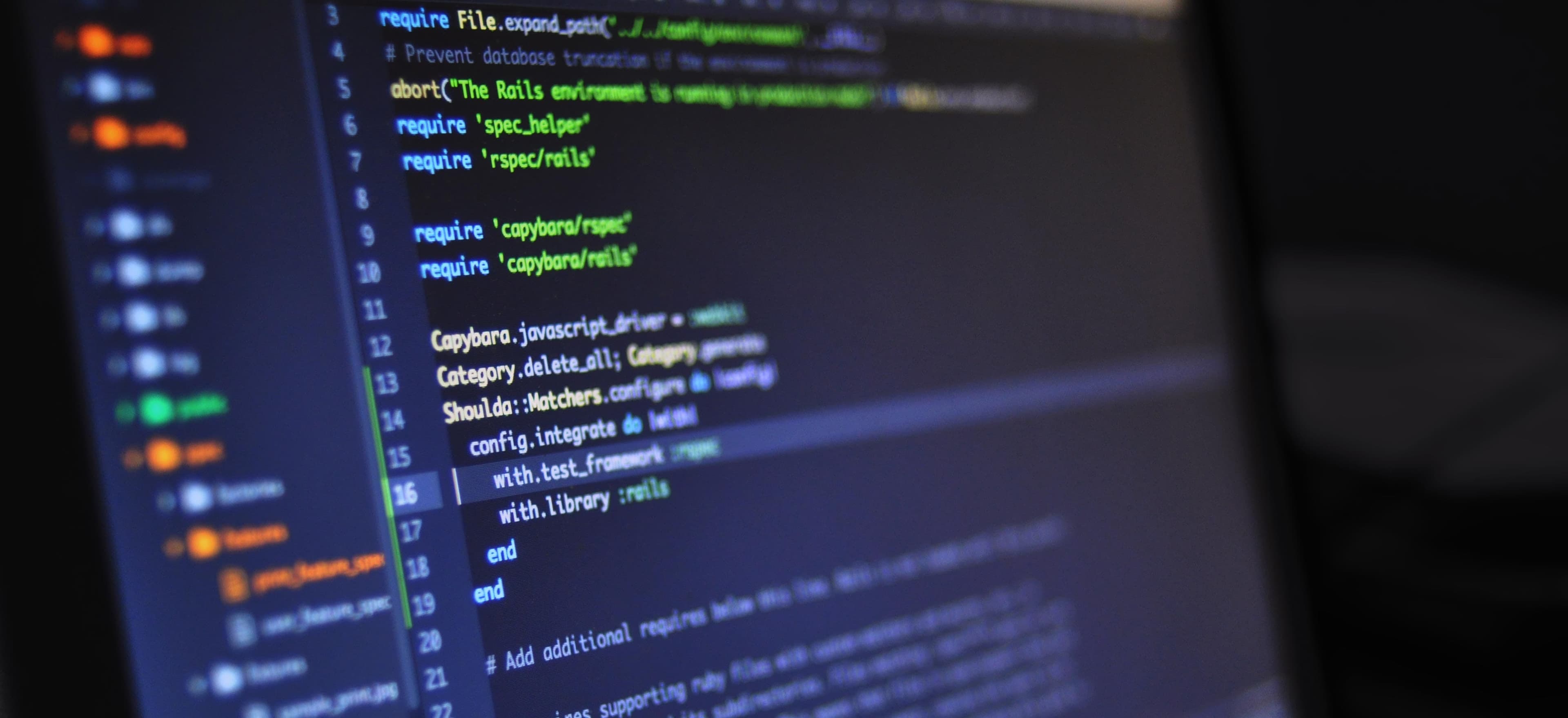
- Published on
Troubleshooting Hazelcast Configuration in Hibernate Caching
Caching is an essential optimization technique in web application development. It helps in dramatically reducing response times by storing frequently accessed data in memory. When using Hibernate, integrating Hazelcast as a distributed caching layer is a common approach. However, developers occasionally face issues in configuring Hazelcast correctly with Hibernate. This blog post will walk you through the process of troubleshooting Hazelcast configuration in Hibernate caching.
What is Hazelcast?
Hazelcast is an in-memory data grid that provides a distributed solution for caching data. It allows your application to scale out effortlessly by distributing data across a cluster of nodes. Unlike traditional caching solutions, Hazelcast is highly available and resilient, making it a popular choice amongst developers using Hibernate.
Why Use Hazelcast with Hibernate?
Integrating Hazelcast with Hibernate caching allows you to benefit from:
- Scalability: Automatically partitions data across nodes, enabling horizontal scaling.
- High Availability: Offers data replication and fault tolerance to ensure data availability.
- Performance: Reduces database load by returning results directly from the cache.
Basic Setup: Hibernate and Hazelcast
To begin troubleshooting, ensure you have a basic setup correctly configured. Here’s a sample configuration in your persistence.xml
or hibernate.cfg.xml
.
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">com.hazelcast.hibernate.HazelcastCacheRegionFactory</property>
<property name="hazelcast.config">hazelcast.xml</property>
This setup tells Hibernate to utilize Hazelcast as its second-level cache. The hazelcast.config
property points to the Hazelcast configuration file, typically named hazelcast.xml
, which needs to be in your classpath.
Common Issues and Troubleshooting Steps
1. Missing Hazelcast Dependency
First and foremost, ensure that your project has the necessary Maven dependencies for Hazelcast and Hibernate. Without them, your application will not run correctly.
Here’s how to include them in your pom.xml
:
<dependency>
<groupId>com.hazelcast</groupId>
<artifactId>hazelcast</artifactId>
<version>5.0</version> <!-- Use the latest stable version -->
</dependency>
<dependency>
<groupId>com.hazelcast</groupId>
<artifactId>hazelcast-hibernate52</artifactId>
<version>5.0</version> <!-- Match version with Hazelcast -->
</dependency>
2. Hazelcast Configuration File Issues
Verify that your hazelcast.xml
file is present under src/main/resources
. An incorrectly configured Hazelcast XML can cause your application to fail in setting up the cache.
Here’s a simple hazelcast.xml
configuration:
<hazelcast>
<network>
<join>
<multicast enabled="true">
<multicast-group>224.2.2.3</multicast-group>
</multicast>
<tcp-ip enabled="false" />
</join>
</network>
<map name="your-map-name">
<backup-count>1</backup-count>
<time-to-live-seconds>3600</time-to-live-seconds>
</map>
</hazelcast>
This sets up a multicast join for cluster formation, alongside a simple map configuration. Ensure your map name matches what you’ve defined in your Hibernate entity.
3. Entity Caching Strategies
In Hibernate, you can define caching strategies for your entities. Ensure that the entities you intend to cache are marked correctly. Here’s a sample entity caching setup:
@Entity
@Cacheable
@Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// getters and setters
}
Using @Cacheable
and specifying a cache strategy ensures that Hibernate knows to use the cache for this entity.
4. Avoid Cache Inconsistencies
Cache inconsistencies can occur in a distributed environment. Make sure to carefully handle the entity lifecycle. Use @CacheEvict
and related annotations judiciously to maintain cache integrity.
@Transactional
public void updateProduct(Product product) {
productRepository.save(product);
// Evict the cache for this entity
cacheManager.getCache("your-map-name").evict(product.getId());
}
5. Debugging
If you continue to face issues, you might want to enable debug logging for both Hibernate and Hazelcast.
In your log4j.properties
, enable debug-level logging for the relevant packages:
log4j.logger.org.hibernate=DEBUG
log4j.logger.com.hazelcast=DEBUG
This will provide verbose output that can help pinpoint the problem areas in your configuration.
Additional Resources
To deepen your understanding, consider exploring the following:
- Official Hazelcast Documentation
- Hibernate Documentation
Final Thoughts
Troubleshooting Hazelcast configuration in Hibernate can be tricky but is manageable with a structured approach. By ensuring all dependencies are included, the configuration files are correctly set up, and entities are configured for caching, most issues can be resolved. Remember to make use of logging to track down elusive problems.
Caching is a powerful tool. When employed correctly, it can vastly improve application performance and user experience. Happy coding!
Checkout our other articles