Mastering the Strategy Pattern in Spring Applications
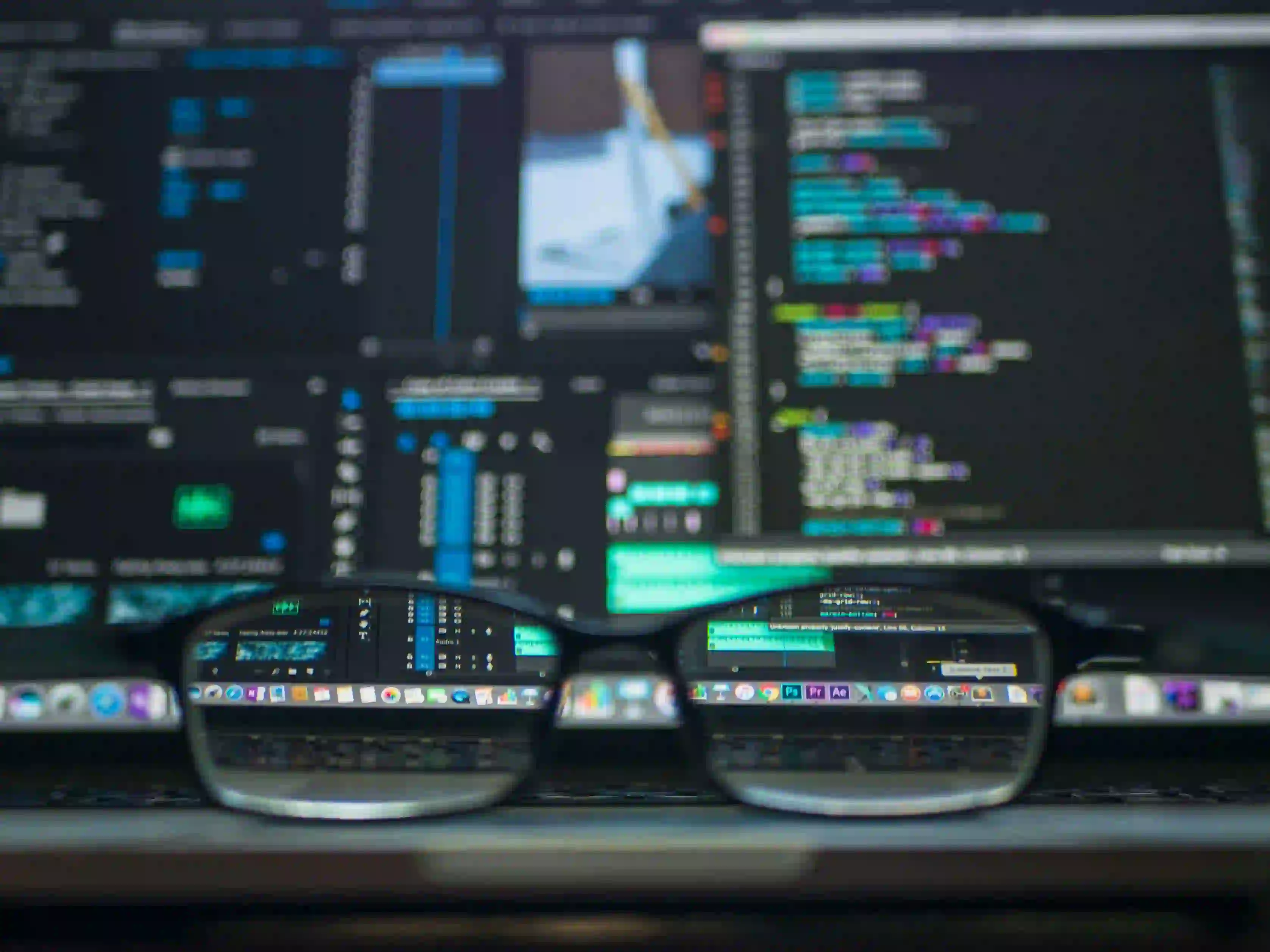
Mastering the Strategy Pattern in Spring Applications
The Strategy Pattern is a behavioral design pattern that enables selecting an algorithm's behavior at runtime. This pattern is particularly useful in Spring applications, which often prioritize flexibility, maintainability, and reusability. This blog post dives into the Strategy Pattern, exploring its principles, benefits, and implementation in Spring applications.
What is the Strategy Pattern?
The Strategy Pattern allows developers to define a family of algorithms, encapsulate each one, and make them interchangeable. This enables a client to choose the algorithm to use at runtime, which promotes the Open/Closed Principle—a key tenet of SOLID design principles.
Key Components
- Context: Maintains a reference to the Strategy.
- Strategy Interface: Declares a common interface for all strategies.
- Concrete Strategies: Implements the Strategy interface to provide specific algorithms.
Why Use the Strategy Pattern?
- Flexibility: Easily switch between algorithms or behaviors at runtime.
- Separation of Concerns: Keep your codebase clean by separating the algorithm from the context.
- Maintainability: Changes to algorithms require fewer alterations to the context class.
- Interchangeability: Easily add new strategies without modifying existing code.
Implementing the Strategy Pattern in a Spring Application
Example Scenario
Imagine we are constructing an e-commerce application where we must calculate shipping costs based on various methods—Standard, Express, and Overnight. Each method varies in cost calculation, which makes it a perfect candidate for the Strategy Pattern.
Step 1: Define the Strategy Interface
We'll start by creating a strategy interface named ShippingStrategy
.
public interface ShippingStrategy {
double calculateShippingCost(Order order);
}
Step 2: Implement Concrete Strategies
Next, we'll create concrete implementations for each shipping method.
Standard Shipping
public class StandardShipping implements ShippingStrategy {
@Override
public double calculateShippingCost(Order order) {
return order.getWeight() * 5.0;
}
}
Express Shipping
public class ExpressShipping implements ShippingStrategy {
@Override
public double calculateShippingCost(Order order) {
return order.getWeight() * 10.0;
}
}
Overnight Shipping
public class OvernightShipping implements ShippingStrategy {
@Override
public double calculateShippingCost(Order order) {
return order.getWeight() * 20.0;
}
}
Step 3: The Context Class
The context class interacts with the chosen strategy. In our case, we can create a ShippingContext
.
public class ShippingContext {
private ShippingStrategy strategy;
public void setShippingStrategy(ShippingStrategy strategy) {
this.strategy = strategy;
}
public double calculateShippingCost(Order order) {
return strategy.calculateShippingCost(order);
}
}
Step 4: Putting It All Together
Now we can use our strategy in a Spring application. Here's how it can be done in a service layer.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class ShippingService {
private ShippingContext shippingContext;
@Autowired
public ShippingService(ShippingContext shippingContext) {
this.shippingContext = shippingContext;
}
public double calculateShipping(Order order, String shippingMethod) {
switch (shippingMethod) {
case "STANDARD":
shippingContext.setShippingStrategy(new StandardShipping());
break;
case "EXPRESS":
shippingContext.setShippingStrategy(new ExpressShipping());
break;
case "OVERNIGHT":
shippingContext.setShippingStrategy(new OvernightShipping());
break;
default:
throw new IllegalArgumentException("Invalid shipping method");
}
return shippingContext.calculateShippingCost(order);
}
}
Step 5: Testing the Implementation
Let's write a JUnit test case to verify our strategy implementation.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class ShippingServiceTest {
private ShippingService shippingService = new ShippingService(new ShippingContext());
@Test
void testStandardShipping() {
Order order = new Order(10); // weight of 10kg
double cost = shippingService.calculateShipping(order, "STANDARD");
assertEquals(50.0, cost);
}
@Test
void testExpressShipping() {
Order order = new Order(10); // weight of 10kg
double cost = shippingService.calculateShipping(order, "EXPRESS");
assertEquals(100.0, cost);
}
@Test
void testOvernightShipping() {
Order order = new Order(10); // weight of 10kg
double cost = shippingService.calculateShipping(order, "OVERNIGHT");
assertEquals(200.0, cost);
}
}
Lessons Learned
The Strategy Pattern is a powerful tool that can significantly enhance the flexibility and maintainability of your applications. By encapsulating algorithms within their own classes, you allow the client to dynamically choose which behavior to use.
By implementing this pattern in your Spring applications, you can ensure that your code adheres to SOLID principles, making it easier to extend and modify in the future.
For further reading on design patterns in Java, I highly recommend Design Patterns: Elements of Reusable Object-Oriented Software or exploring spring's official documentation on Spring Framework.
Implementing design patterns like the Strategy Pattern can lead to cleaner, more efficient code that meets the evolving demands of modern software development. Happy coding!