Troubleshooting Java JSch: Common Errors in Remote File Access
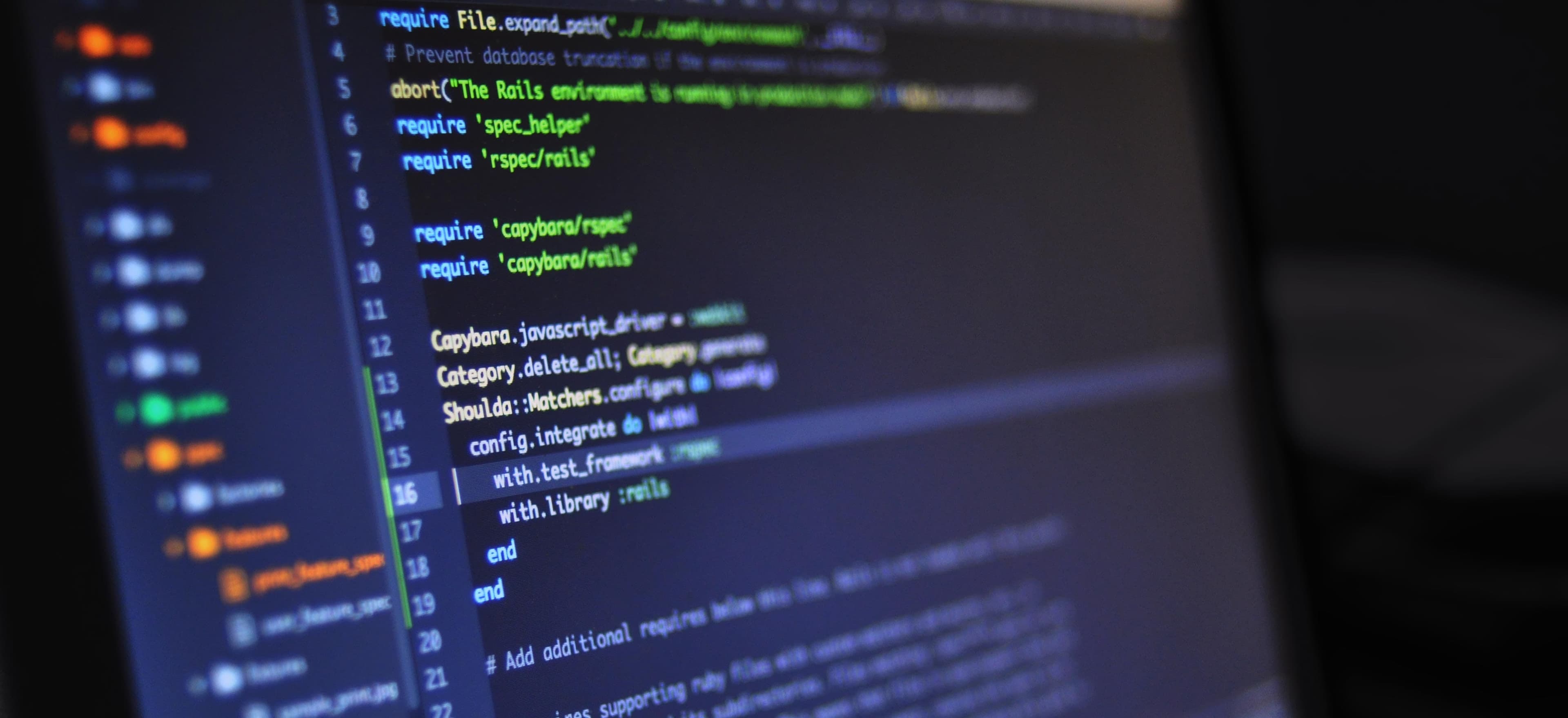
- Published on
Troubleshooting Java JSch: Common Errors in Remote File Access
Java Secure Channel (JSch) is a popular library used for SSH connectivity in Java applications. It allows developers to access remote servers and transfer files securely. However, like any other technology, you may encounter various issues while using JSch for remote file access. This blog post will discuss common errors when using JSch and how to troubleshoot them effectively.
Table of Contents
- Introduction to JSch
- Common Errors in Remote File Access
- Authentication Issues
- Connection Timeouts
- File Not Found Errors
- Permissions Denied Errors
- Protocol Errors
- Best Practices for Using JSch
- Conclusion
1. Introduction to JSch
JSch is a Java library that enables SSH and SFTP communication. It is lightweight and highly configurable, making it a great choice for developers. Whether you're transferring files, executing remote commands, or performing encrypted data exchanges, JSch can simplify these tasks.
Before diving into error troubleshooting, make sure you have the library added to your project. If you are using Maven, include the following dependency in your pom.xml
:
<dependency>
<groupId>com.jcraft</groupId>
<artifactId>jsch</artifactId>
<version>0.1.55</version>
</dependency>
You can find the latest version here.
2. Common Errors in Remote File Access
Understanding and identifying common errors is essential for efficient troubleshooting. Below are some frequent issues encountered when working with JSch.
Authentication Issues
Problem: The authentication process could fail, leading to an Auth fail
message.
Resolution:
- Check username and password: Ensure you are using the correct credentials.
- Key-based authentication: If you are using SSH keys, ensure the private key file is correct and accessible. Set it up as follows:
Session session = jsch.getSession(user, host, port);
jsch.addIdentity("/path/to/privateKey");
Using SSH keys enhances the security of your authentication process.
- Known hosts verification: If you're connecting to a new server, you may need to disable host key checking temporarily (not recommended for production):
session.setConfig("StrictHostKeyChecking", "no");
Connection Timeouts
Problem: The connection to the server fails, resulting in a Timeout
error.
Resolution:
- Ensure the remote server is up and reachable. Use
ping
to check its accessibility. - Increase timeout settings:
session.connect(30000); // wait for 30 seconds
- Verify your network configurations, such as firewall settings, that may block the SSH port (default is 22).
File Not Found Errors
Problem: When attempting to retrieve a file, you may encounter a File not found
error.
Resolution:
- Check file path: Ensure the file path is correctly specified with the appropriate directory structure.
- Verify file existence: Use SSH or SFTP commands directly in the terminal to check if the file exists:
ChannelSftp sftpChannel = (ChannelSftp) session.openChannel("sftp");
sftpChannel.connect();
sftpChannel.ls(directoryPath); // check files in the directory
Permissions Denied Errors
Problem: Permission denied errors occur when the user account does not have the required rights to access or modify the file.
Resolution:
- Check remote file permissions: Use the
ls -la
command to list files with permissions. You may need to change the permissions usingchmod
:
chmod 600 /path/to/privateKey
- Ensure correct user roles: Make sure you are using a user account that has access to the directory in question.
Protocol Errors
Problem: Protocol errors can occur due to mismatched settings or protocols.
Resolution:
- Validate the protocol: Ensure that you are using the correct protocol (SSH, SFTP) for your operations.
- Update JSch library: Check if you are using the latest version of JSch. An outdated library can sometimes lead to protocol issues.
3. Best Practices for Using JSch
To minimize errors and optimize performance in your JSch implementations, consider the following best practices:
Error Handling
Always implement robust error handling. Wrap your calls with try-catch blocks and log exceptions for easier troubleshooting later:
try {
session.connect();
} catch (JSchException e) {
System.err.println("Connection failed: " + e.getMessage());
}
Resource Management
Close your sessions and channels properly to avoid resource leaks. Here's an example:
sftpChannel.disconnect();
session.disconnect();
Maintain Security Standards
Use key-based authentication whenever possible. Avoid hardcoded credentials in your source code. Consider configuring environment variables to store sensitive data.
Regular Updates
Keep your libraries and dependencies updated. This not only includes JSch but any related library you use for security or connectivity. Keeping your software updated can mitigate many known issues.
For more in-depth knowledge about secure file transfer protocols, refer to this Securing File Transfers whitepaper.
4. Conclusion
In summary, JSch is a powerful library that simplifies SSH and SFTP operations in Java applications. However, troubleshooting common errors is a crucial part of using JSch effectively. By understanding the issues around authentication, connection, file access, permissions, and protocols, you can navigate through your development challenges more effectively.
Adhering to best practices like proper error handling, resource management, security standards, and regular updates will ensure that your application runs smoothly and securely.
For further reading and resources, don't hesitate to check the official JSch documentation for additional features and functionalities.
Having addressed these common errors and best practices, dive into your next JSch project with confidence! Happy coding!
Checkout our other articles