How Copy-Paste Can Stall Your Software Development Progress
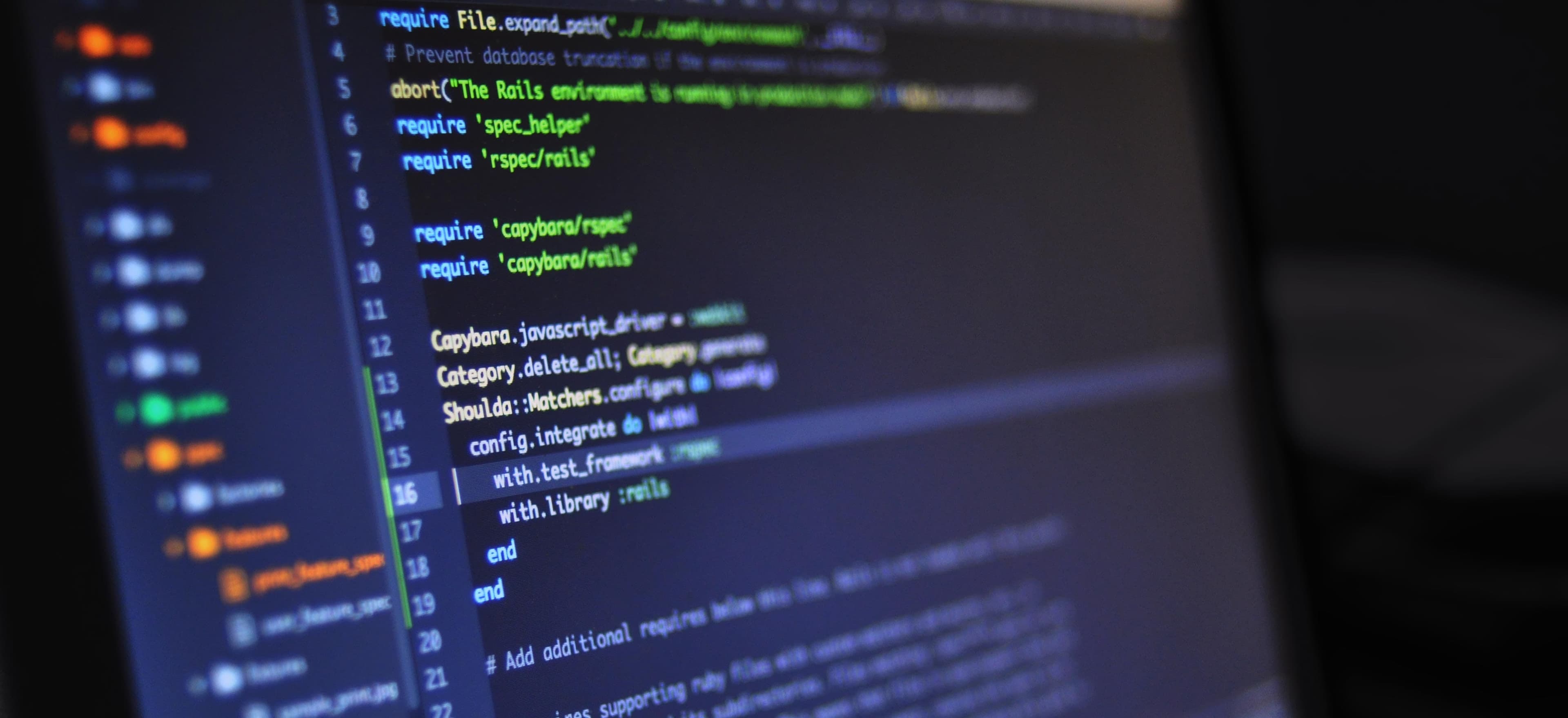
- Published on
How Copy-Paste Can Stall Your Software Development Progress
In the landscape of software development, the practice of copy-pasting code is as common as it is contentious. While it can save time and streamline certain tasks, reliance on this method can significantly stall your development progress over the long term. In this article, we will delve into the drawbacks of copying and pasting code, discuss better practices, and provide code snippets to illustrate our points.
The Allure of Copy-Paste
When faced with a daunting programming challenge, the temptation to copy and paste can be overwhelmingly appealing. After all, why reinvent the wheel? Many developers find that leveraging existing code can accelerate development timelines, reducing initial overhead. Here's a simple Java code snippet illustrating this point:
// This method calculates the area of a rectangle
public static int calculateArea(int length, int width) {
return length * width;
}
In the heat of development, a programmer may copy this snippet into another part of the application to reuse its functionality. On the surface, this seems efficient.
The Hidden Costs of Copy-Pasting
However, this seemingly harmless practice can lead to numerous pitfalls:
-
Code Duplication: When code is duplicated across your codebase, any changes or bug fixes must be implemented at multiple locations. This increases the risk of inconsistencies and errors.
For instance, if a developer later identifies that the calculation method needs to handle negative dimensions, they must remember to update every instance of that copied code, which can lead to oversight.
-
Technical Debt: By copying code, developers often overlook the opportunity to refactor or improve the logic. This festers into technical debt, where the code becomes harder and more costly to manage over time.
-
Reduced Readability: When code is copied and pasted, its context may be lost. Future developers (and even yourself) may struggle to understand why it was implemented that way, leading to more confusion and time spent deciphering the code.
-
Difficulty in Testing: With multiple instances of similar code scattered throughout your application, unit testing becomes cumbersome. You must create tests for each duplicate, rather than being able to write a single, comprehensive test for a method.
Better Practices Over Copy-Paste
While it might be tempting to copy-paste code to achieve short-term goals, there are better practices that can yield long-term benefits:
1. Code Reusability with Functions
Instead of duplicating code, extract common logic into functions or methods. This promotes reusability and enhances maintainability. Here's an example:
// Function to calculate the area of a rectangle
public static int calculateArea(int length, int width) {
return length * width;
}
You can call this single calculateArea
method wherever you need to compute the area.
2. Use Libraries and Frameworks
Utilizing established libraries can save you time while ensuring best practices. Java has a wealth of frameworks like Spring and Hibernate that can alleviate the need for redundant code.
For example, instead of writing your own data access logic repeatedly, consider using Hibernate:
import org.hibernate.Session;
import org.hibernate.Transaction;
public void saveProduct(Product product) {
Transaction transaction = null;
try (Session session = HibernateUtil.getSessionFactory().openSession()) {
transaction = session.beginTransaction();
session.save(product);
transaction.commit();
} catch (Exception e) {
if (transaction != null) {
transaction.rollback();
}
e.printStackTrace();
}
}
In this code snippet, you're leveraging Hibernate to handle the complexity of database transactions rather than reinventing the wheel with every save operation.
3. Adopt Design Patterns
Design patterns like Singleton, Factory, and Observer can provide guided solutions to common problems, minimizing the need for copy-paste solutions.
For instance, here's an implementation of the Singleton pattern:
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Using design patterns can not only help in reducing code duplication but also make your software more robust and easier to understand.
4. Use Code Snippet Managers
If you frequently find yourself using snippets, consider a snippet manager tool. Applications like SnippetsLab or Boostnote allow you to store and categorize reusable code segments. This avoids the need to copy from elsewhere while ensuring you have a library of reusable code at hand.
5. Refactor Regularly
Taking the time to periodically refactor your code is essential. By assessing your codebase regularly for duplicates and improving functionality, you can mitigate dependencies on copy-pasting.
The Last Word
While copy-pasting can provide short-term gains in software development, it often leads to long-term inefficiencies and frustrations. By embracing methods such as code reusability via functions, utilizing existing libraries, applying design patterns, employing snippet managers, and routinely refactoring, developers can foster a more productive and maintainable coding environment.
The next time you are tempted to copy and paste, take a moment to evaluate whether it might be better to create, refactor, or utilize existing code. The transition may be challenging at first, but in the end, it will undoubtedly pay off by improving the quality of your software and expediting your development progress.
For further reading on this topic, consider checking out resources from Stack Overflow Blog and Martin Fowler's Refactoring.
Happy coding, and remember: quality over quantity!
Checkout our other articles