Overcoming Complexity: Mastering the Composite Design Pattern
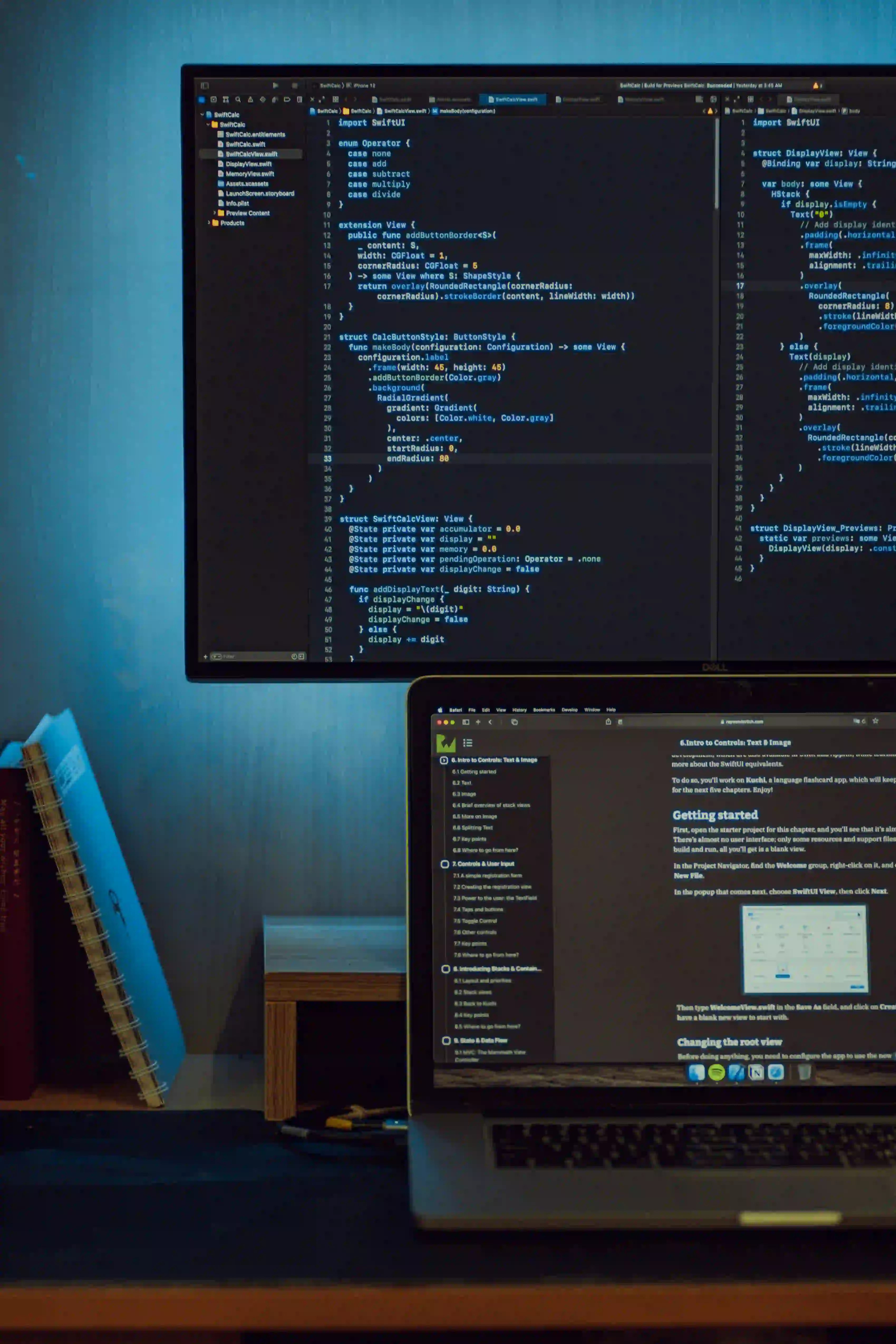
Overcoming Complexity: Mastering the Composite Design Pattern
When embarking on a journey through the world of software design, you will encounter a myriad of design patterns that can help structure your code efficiently. One pattern you might find particularly useful is the Composite Design Pattern. This pattern elegantly tackles situations where you need to treat individual objects and compositions of objects uniformly. In this blog post, we will delve into the Composite Design Pattern, exploring its core concepts, advantages, and practical implementations in Java.
What is the Composite Design Pattern?
The Composite Design Pattern is a structural pattern that allows clients to work with individual objects and compositions of objects in a uniform manner. It lets you create tree structures that organize objects into a hierarchy. This pattern is particularly useful when you want to represent part-whole hierarchies naturally.
Real-World Analogy
Consider a file system on your computer. A folder can contain files or other folders. You can manipulate both files and folders using the same interface. This uniformity provides a clear structure, simplifying operations like searching, deleting, or renaming items irrespective of their type.
Key Components
Before diving into code, it’s essential to understand the key components of the Composite Pattern:
- Component: An abstract class or interface that declares common methods for leaf and composite objects.
- Leaf: Represents the individual objects in the composition. These objects do not have any children.
- Composite: This class implements the component interface. It stores child components and implements methods to manipulate them.
UML Diagram
Here’s a simplified UML diagram for the Composite Design Pattern:
Component
/ \
Leaf Composite
/ \
Leaf Leaf
Advantages of the Composite Pattern
- Simplicity: The client code can work with simple and complex objects uniformly, enhancing readability.
- Flexibility: It allows you to add new components easily, as both leaves and composites implement the same interface.
- Easier Management: Hierarchical structures are easier to manage, supporting operations like addition and deletion naturally.
Implementing the Composite Pattern in Java
Let’s put our understanding into action. Below, we implement the Composite Design Pattern in Java with a simple example: A graphic drawing application that can manage shapes.
Step 1: Define the Component Interface
First, we define our Shape
component, which will be implemented by both Circle
and CompositeShape
.
// Component
public interface Shape {
void draw();
}
Step 2: Create Leaf Classes
Next, we implement the Circle
class, representing individual shape objects.
// Leaf
public class Circle implements Shape {
private String color;
public Circle(String color) {
this.color = color;
}
@Override
public void draw() {
System.out.println("Drawing a " + color + " circle.");
}
}
Step 3: Create the Composite Class
Now, let’s implement the CompositeShape
class, which can hold multiple Shape
objects.
// Composite
import java.util.ArrayList;
import java.util.List;
public class CompositeShape implements Shape {
private List<Shape> shapes = new ArrayList<>();
public void addShape(Shape shape) {
shapes.add(shape);
}
public void removeShape(Shape shape) {
shapes.remove(shape);
}
@Override
public void draw() {
System.out.println("Drawing Composite Shape:");
for (Shape shape : shapes) {
shape.draw();
}
}
}
Step 4: Putting It All Together
To see the Composite Design Pattern in action, we can use the following code in our main method.
public class Main {
public static void main(String[] args) {
Shape circle1 = new Circle("red");
Shape circle2 = new Circle("blue");
CompositeShape compositeShape = new CompositeShape();
compositeShape.addShape(circle1);
compositeShape.addShape(circle2);
compositeShape.draw();
// Adding more complex shape
Shape circle3 = new Circle("green");
compositeShape.addShape(circle3);
compositeShape.draw();
}
}
Why This Code Works
- Separation of Concerns: The
Shape
interface abstracts the concept of a shape. TheCircle
class implements drawing functionality specific to circles, whileCompositeShape
manages multiple shapes. - Flexibility: You can add more shapes easily. For instance, if you want to implement a
Rectangle
class, it simply needs to implement theShape
interface. - Maintainability: The hierarchical structure provides a clear framework for managing shapes and their operations.
When to Use the Composite Pattern
While powerful, the Composite Design Pattern should be used judiciously. It is most suitable in situations like:
- Situations where clients should be able to treat both individual and composite objects uniformly (e.g., graphical interfaces).
- Implementing tree-like structures such as file systems, organization structures, or even component-based systems.
The Bottom Line
The Composite Design Pattern serves as an invaluable tool when it comes to modeling relationships that involve part-whole hierarchies. It elegantly simplifies code management by allowing individual objects and composites to be treated uniformly.
You’ve seen how to implement the pattern in Java, highlighting not just the mechanics, but also the rationale behind each step. As you continue your journey into design patterns, remember that understanding the "why" can be just as critical as the "how."
To further enhance your understanding, consider exploring additional resources on design patterns or reviewing the Java documentation.
Happy coding!