Navigating the Challenges of Monolith to Microservices Transition
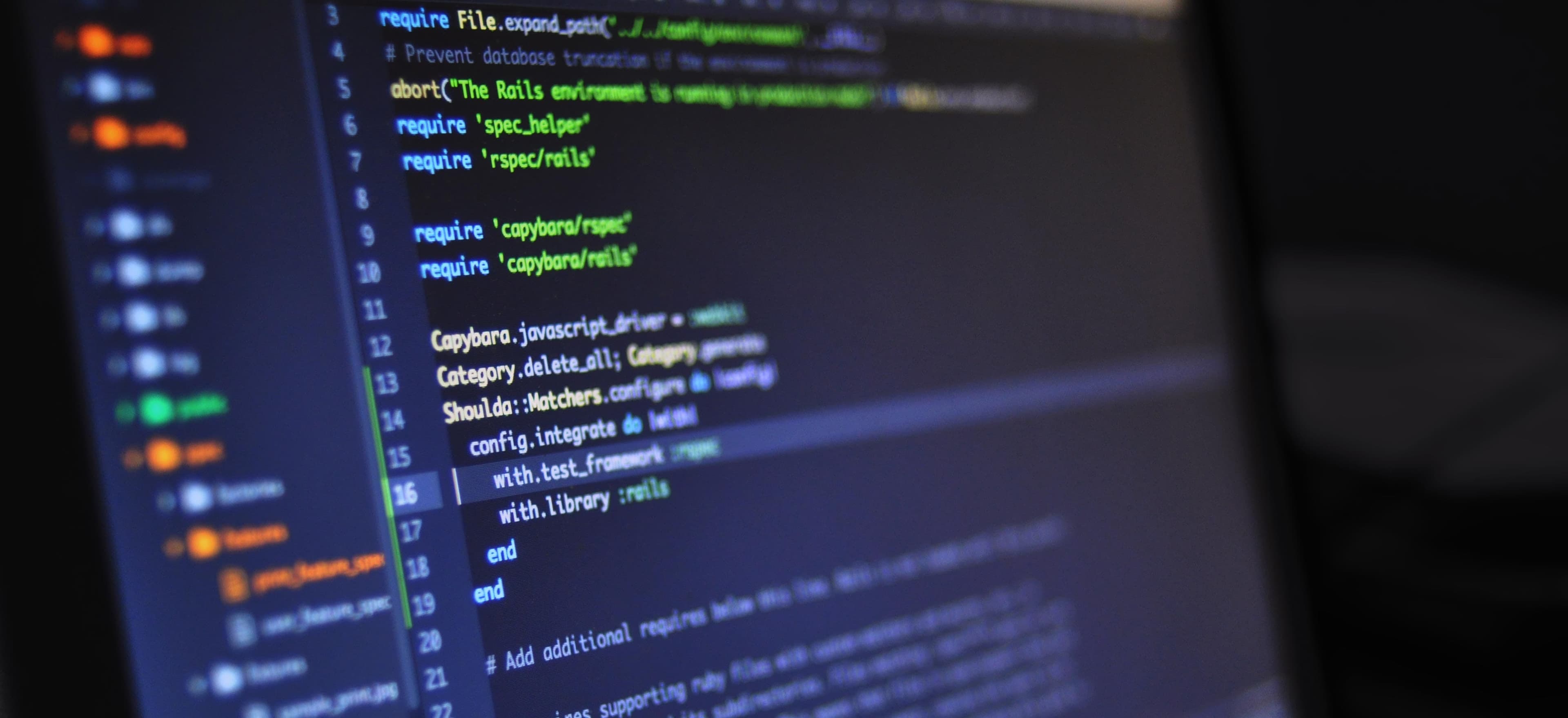
- Published on
Navigating the Challenges of Monolith to Microservices Transition
Transitioning from a monolithic architecture to microservices can be an exciting journey for developers and businesses alike. As organizations aim to improve scalability, maintainability, and deployment velocity, they often discover the challenges that come along the way. This blog post will delve into these challenges and provide actionable strategies to navigate this transformative transition effectively.
Understanding Monolith vs. Microservices
Monolithic Architecture
A monolithic architecture entails building a single large application where all components run as a single service. This approach offers several advantages, such as simplicity in development, easier testing, and straightforward deployment. However, as applications grow larger, several challenges arise.
Drawbacks of a Monolithic Architecture
- Tight Coupling: Components are interdependent, making changes difficult without affecting the entire application.
- Scalability: Scaling the application often means scaling the entire system, leading to resource inefficiencies.
- Deployment Issues: A bug in one part can lead to deploying the entire application, increasing downtime and risk.
Microservices Architecture
In contrast, microservices architecture breaks the application into smaller, independent services that communicate through APIs. This modularity improves flexibility and allows teams to work on different services simultaneously.
Advantages of Microservices
- Scalability: Individual microservices can be scaled independently, optimizing resource utilization.
- Flexibility in Technology Stack: Teams can choose different tech stacks for different services based on their requirements.
- Improved Deployment: Smaller, independent deployments reduce the risk of a full system failure.
Key Challenges of Transitioning from Monolith to Microservices
Despite the advantages that microservices offer, the transition is not a walk in the park. Let's outline the primary challenges developers may encounter:
1. Understanding Domain-Driven Design (DDD)
DDD is crucial for structuring microservices effectively. Each service should be designed around a specific business capability. Without a deep understanding of DDD, you may end up with poorly defined microservices that do not effectively solve your business needs.
Actionable Tip: Take the time to map out your domain and identify bounded contexts. This exercise will guide you in service segmentation.
2. Managing Data
With a monolithic architecture, data is often housed in a single database. In microservices, each service should ideally have its own database to enable independence. This leads to challenges in data consistency and management.
Example Code Snippet: Database Connection in Microservices
Here's a simple representation of how different microservices might connect to their own databases:
package com.example.service;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.jdbc.datasource.DriverManagerDataSource;
import javax.sql.DataSource;
public class MyDatabaseService {
private JdbcTemplate jdbcTemplate;
public MyDatabaseService() {
this.jdbcTemplate = new JdbcTemplate(createDataSource());
}
private DataSource createDataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/myservice_db");
dataSource.setUsername("user");
dataSource.setPassword("password");
return dataSource;
}
public void fetchData() {
String sql = "SELECT * FROM my_table";
jdbcTemplate.queryForList(sql).forEach(row -> System.out.println(row));
}
}
Why This Matters: Each microservice needs its own database to maintain independence, thereby enabling teams to innovate and deploy without disrupting others.
3. Inter-Service Communication
Microservices need to communicate with each other, which can lead to significant complexity if not managed properly. Asynchronous messaging or synchronous calls via REST APIs can be employed, leading to performance variations and potential bottlenecks.
Actionable Tip: Investigate using message brokers (such as RabbitMQ, Kafka, etc.) to handle inter-service communication effectively.
4. DevOps and Continuous Integration/Continuous Deployment (CI/CD)
Microservices deploy independently, necessitating robust DevOps practices. Failure to integrate these practices can lead to inconsistencies in deployment and operational challenges.
Example Snippet: Dockerfile for Setting Up CI/CD
Here’s how you might structure a Dockerfile to prepare for continuous deployments:
# Use the official Java image
FROM openjdk:17-jdk-alpine
# Set the working directory
WORKDIR /app
# Copy the compiled jar file into the container
COPY target/myservice.jar myservice.jar
# Run the application
ENTRYPOINT ["java", "-jar", "myservice.jar"]
Why This Matters: Containerization through tools like Docker simplifies deployments, making microservices manageable within a CI/CD pipeline.
5. Monitoring and Logging
When transitioning to microservices, troubleshooting becomes more complex as the system is distributed across various services. Effective monitoring and logging strategies are essential for maintaining system health.
Actionable Tip: Implement centralized logging solutions (e.g., ELK stack) and define metrics for performance monitoring (e.g., Prometheus with Grafana).
6. Team Structure and Management
Shifting to microservices often requires changes in team structure. Cross-functional teams that can operate independently are essential in ensuring the success of microservices.
Actionable Tip: Foster a culture of collaboration. Use Agile methodologies to enable parallel development and shortened release cycles.
Putting It All Together
Transitioning from a monolithic to a microservices architecture is undoubtedly complex, but with careful planning and execution, teams can reap substantial benefits. There are notable considerations:
- Understand and implement Domain-Driven Design to define service boundaries effectively.
- Address data management through established patterns to maintain independence across microservices.
- Optimize inter-service communications using asynchronous messaging where feasible.
- Develop strong DevOps practices for seamless CI/CD operations.
- Centralize monitoring and logging to maintain oversight over a distributed system.
- Reassess team structures to align with microservices principles.
The Closing Argument
The journey from monolith to microservices is fraught with challenges, but it is also an opportunity for numerous benefits, such as improved scalability, flexibility, and speed of deployment. By understanding the key issues and employing the strategies outlined in this post, organizations can navigate this transition more smoothly.
For further reading on effective microservices architecture, check out Microservices Best Practices for Developers and A Guide to Microservices with Spring. Embrace the journey, invest time in understanding the nuances, and your organization will thrive in a microservices world.