Overcoming Common Pitfalls in Spring Cloud Microservices
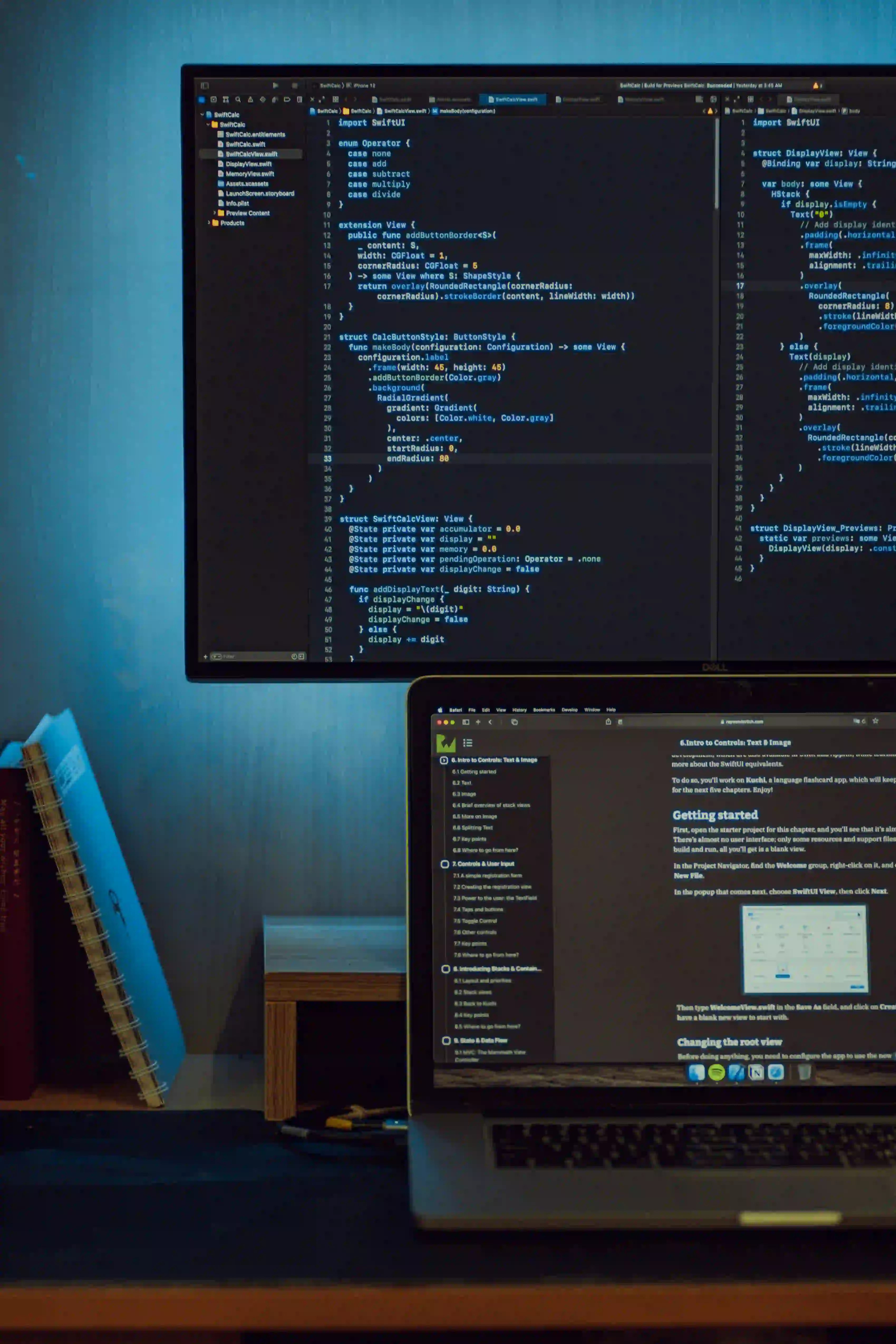
Overcoming Common Pitfalls in Spring Cloud Microservices
Microservices architecture has transformed the way we develop and deploy applications, allowing teams to build independent, scalable services. However, with the power of microservices comes a unique set of challenges, especially when using technologies like Spring Cloud. This blog post aims to highlight some of the most common pitfalls when working with Spring Cloud microservices and provide strategies to overcome them.
Understanding Spring Cloud
Before diving into common pitfalls, let's clarify what Spring Cloud is. Spring Cloud is a suite of tools and frameworks designed to aid in the development of distributed systems in the Spring ecosystem. It provides features such as service discovery, configuration management, circuit breakers, and more, which help manage microservices effectively.
However, with the conveniences it offers, developers can sometimes overlook best practices, leading to inefficient architectures and hard-to-maintain systems. Let's explore some of these common pitfalls and how to navigate around them effectively.
Pitfall 1: Overusing Service Discovery
Service discovery, facilitated by components like Netflix Eureka, is a powerful feature of Spring Cloud. It allows microservices to find each other without hardcoding IP addresses. While this is incredibly useful, over-reliance on service discovery can lead to performance overhead.
Solution: Implement Caching
To mitigate the performance costs, consider implementing a client-side caching mechanism. Spring Cloud load balancers (like Ribbon) can cache service instances, which reduces the number of requests to the service registry.
@RestController
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/users/{id}")
public User getUserById(@PathVariable String id) {
return userService.getUserById(id);
}
}
Using annotations like @LoadBalanced
with your RestTemplate can also help in making the service invocation more efficient.
@Bean
@LoadBalanced
public RestTemplate restTemplate() {
return new RestTemplate();
}
Why This Matters
Caching reduces the load on the service registry and speeds up service discovery, enabling your microservices to perform better under heavy loads.
Pitfall 2: Ignoring Configuration Management
A common mistake in Spring Cloud microservices is neglecting external configuration management, particularly with Spring Cloud Config. Hardcoding configuration parameters directly into services can lead to inconsistency and difficulty in managing deployments.
Solution: Leverage Spring Cloud Config
Utilize Spring Cloud Config Server to externalize your configuration. This allows you to manage various configurations for different environments (development, testing, production) all in one place.
# application.yml
spring:
cloud:
config:
uri: http://localhost:8888
By pulling configurations from a centralized location, you drastically simplify management processes and ensure that your microservices remain loosely coupled.
Why This Matters
Centralized configuration management boosts application reliability. It allows changes to be made without redeploying services, thus minimizing downtime.
Pitfall 3: Failing to Implement Circuit Breakers
Microservices are prone to failure. Network issues and service outages can cascade through the system if not managed properly. Failing to implement circuit breakers can lead to application instability.
Solution: Utilize Resilience4j or Hystrix
Resilience4j is a lightweight and robust library that provides circuit breaker, rate limiter, and other resilience patterns. If you haven’t yet transitioned from Hystrix, consider this modern alternative.
Here’s a simple circuit breaker example using Resilience4j:
@Service
public class PaymentService {
@CircuitBreaker
public PaymentResponse processPayment(PaymentRequest request) {
// Logic for processing payment
}
}
Why This Matters
Implementing a circuit breaker prevents a failing service from repeatedly executing requests that are destined to fail, conserving resources and improving overall system resilience.
Pitfall 4: Inadequate Monitoring and Logging
Microservices add layers of complexity to your applications. Failing to implement adequate logging and monitoring can obscure visibility into your application's health and performance.
Solution: Integrate Sleuth and Zipkin
Spring Cloud Sleuth and Zipkin can provide distributed tracing capabilities, enabling you to monitor your microservices' performance effectively.
In your application, you can annotate your service methods for automatic tracing:
@Service
public class OrderService {
@Autowired
private Tracer tracer;
public void createOrder(Order order) {
tracer.nextSpan().name("create-order").start();
// Logic to create and persist the order
tracer.currentSpan().finish();
}
}
Why This Matters
Comprehensive monitoring and logging empower developers to trace issues across microservices, enabling quicker troubleshooting and reducing downtime.
Pitfall 5: Neglecting API Gateway Patterns
When building microservices, another common pitfall is neglecting to implement an API Gateway. While individual services may work well, they can become cumbersome for clients when they have to communicate with multiple microservices directly.
Solution: Implement Spring Cloud Gateway
Spring Cloud Gateway serves as a single entry point to your services. It facilitates routing and basic authorization, allowing clients to make requests without having to find each service individually.
@EnableZuulProxy
@SpringBootApplication
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
}
And in your application.yml
, you can define routes:
spring:
cloud:
gateway:
routes:
- id: user-service
uri: lb://USER-SERVICE
predicates:
- Path=/users/**
Why This Matters
An API Gateway abstracts microservices from the client, improving security and decreasing client complexity.
Closing Remarks
Creating and managing Spring Cloud microservices provides enormous benefits, but it also comes with significant challenges. By recognizing and understanding common pitfalls such as an over-reliance on service discovery, improperly managing configurations, neglecting circuit breakers, inadequate monitoring, and failing to implement an API gateway, developers can proactively address these concerns.
Take the time to carefully assess your microservices architecture. Implement these solutions to improve resilience and maintainability, and ensure you harness the full power of Spring Cloud microservices.
For further reading on Spring Cloud, check out the official Spring Cloud Documentation and consider deep diving into Microservices Patterns to bolster your knowledge.
By learning from common pitfalls and applying best practices, you can create robust microservices that thrive in a distributed environment. Happy coding!