Mastering Java Exceptions: Common Mistakes to Avoid
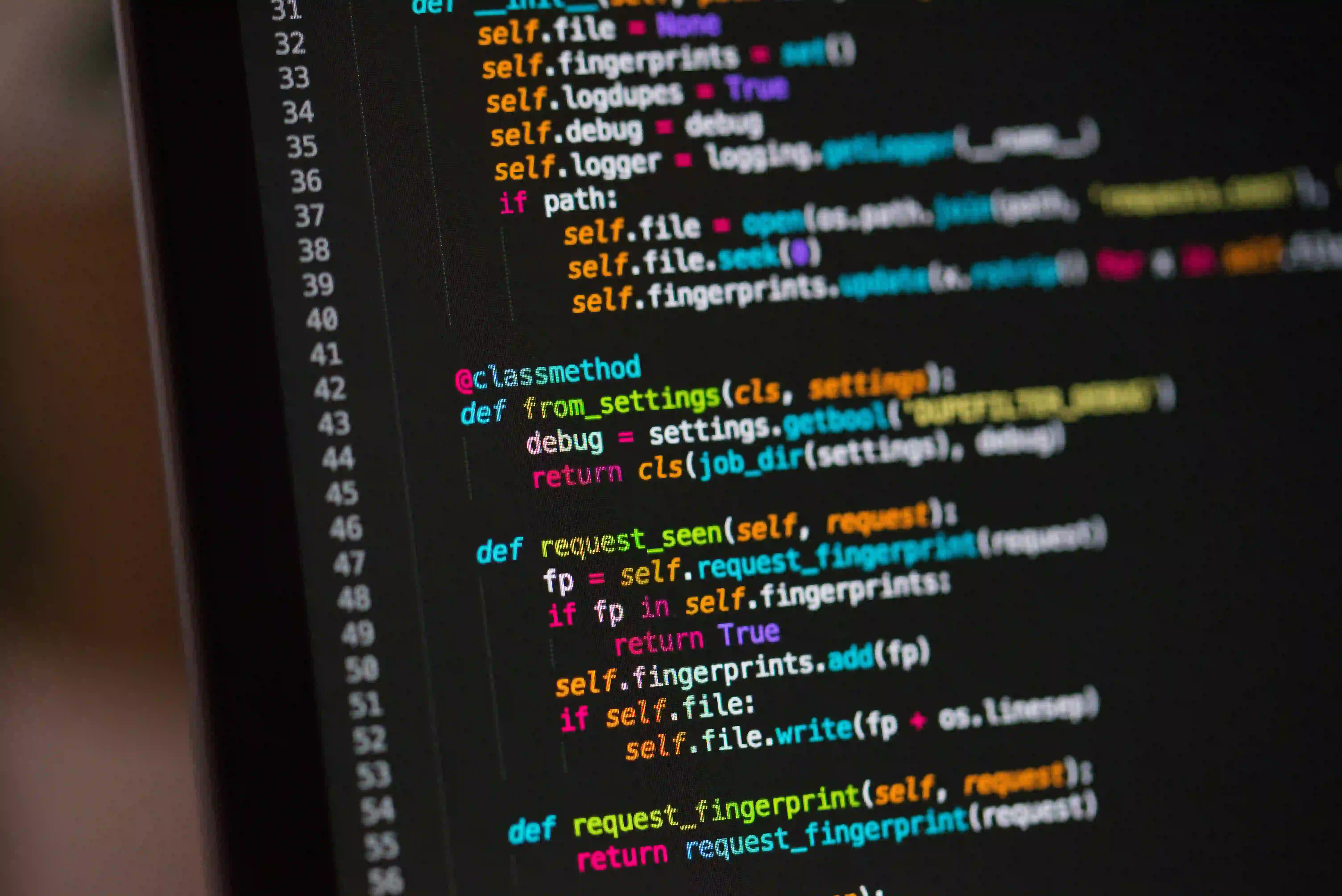
Mastering Java Exceptions: Common Mistakes to Avoid
Java is an object-oriented programming language that offers robust exception handling capabilities. Exception handling is critical because it allows developers to write code that can gracefully handle unexpected issues, ensuring the application continues to run smoothly. However, even experienced Java developers can make several common mistakes when it comes to exception handling. In this blog post, we will explore these pitfalls and the ways you can avoid them.
Understanding Java Exceptions
Before diving into common mistakes, let's take a moment to revisit what exceptions are in Java. An exception is an event that disrupts the normal flow of the application. In Java, exceptions fall into two categories:
-
Checked exceptions: These are checked at compile time. The compiler forces the programmer to handle them. For example, FileNotFoundException is a checked exception because it could occur while trying to open a file that does not exist.
-
Unchecked exceptions: These include runtime exceptions, which are not checked at compile time. Examples include NullPointerException and ArrayIndexOutOfBoundsException. While you can choose to catch these exceptions, you are not obligated to do so.
Understanding the distinctions between these two categories is vital for effective exception handling.
Common Mistakes in Java Exception Handling
1. Catching Generic Exceptions
One of the most prevalent mistakes in Java exception handling is catching very general exceptions such as Exception
or Throwable
. While this may seem convenient, it can hinder your ability to debug the program effectively.
Example:
try {
// Some code that may throw an exception
} catch (Exception e) {
e.printStackTrace(); // Too generic
}
Why Avoid This: By catching a generic exception, you're essentially masking the specific issue, making debugging much harder. Instead, catch specific exceptions.
Better Approach:
try {
// Some code that may throw an exception
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
} catch (IOException e) {
System.out.println("IO exception occurred: " + e.getMessage());
}
2. Ignoring Exceptions
Another common mistake is simply ignoring exceptions when they occur, often by using an empty catch block.
Example:
try {
// Code that could throw an exception
} catch (IOException e) {
// Ignoring the exception
}
Why Avoid This: Ignoring exceptions can lead to unpredictable behavior in your application. This act suppresses information about what went wrong, leading to difficult-to-trace bugs.
Better Approach:
Instead of ignoring it, log the exception or notify the user, depending on the context:
try {
// Some code that may throw an exception
} catch (IOException e) {
logger.error("IO error occurred: ", e); // Log the exception for further analysis
}
3. Throwing Exceptions Without Context
Throwing an exception without providing detailed information can lead to confusion.
Poor Example:
throw new IOException();
Why Avoid This: Without context, the user has no idea what went wrong. A lack of information can result in more debugging work later.
Better Approach:
Include a descriptive message that provides context about the error:
throw new IOException("Failed to read the configuration file due to missing file path.");
4. Using Exceptions for Control Flow
Some developers fall into the trap of using exceptions to manage normal control flow. While Java permits this, it is generally considered bad practice.
Example:
try {
// Code that may take a long time
} catch (TimeoutException e) {
// Managing what should be a control flow
}
Why Avoid This: Using exceptions for control flow can degrade performance and make your code harder to understand. Exceptions are meant for exceptional situations, not routine operations.
Better Approach:
Utilize conditional checks instead of relying on control flow through exceptions:
if (someCondition) {
// Normal control flow
} else {
// Handle the "exceptional" situation
}
5. Not Cleaning Up Resources
Resources such as files, database connections, or even network sockets need to be closed properly. Failing to do this can lead to resource leaks.
Example:
try {
FileInputStream fileInputStream = new FileInputStream("file.txt");
// Working with the file
} catch (IOException e) {
e.printStackTrace();
// Resource not closed!
}
Why Avoid This: Not cleaning up resources can exhaust available file handles or memory in your application, leading to crashes or undesirable states.
Better Approach:
Always ensure resources are cleaned up properly, preferably using a finally
block or the try-with-resources statement introduced in Java 7:
try (FileInputStream fileInputStream = new FileInputStream("file.txt")) {
// Working with the file
} catch (IOException e) {
e.printStackTrace();
}
6. Overusing throws
Clause
When writing methods, it's common to signal that an exception may occur using the throws
clause. However, overusing it can clutter your code.
Example:
public void myMethod() throws IOException, SQLException {
// Method implementation
}
Why Avoid This: Propagating multiple exceptions can make method signatures complex and reduce readability.
Better Approach:
Catch exceptions internally when possible. Use custom exception classes when it makes sense to aggregate several exceptions into a single type.
public void myMethod() {
try {
// Implementation
} catch (IOException | SQLException e) {
throw new MyCustomException("An error occurred", e);
}
}
Closing the Chapter
Mastering Java exceptions takes time and practice. Avoiding the common mistakes outlined above will enhance your code's quality, maintainability, and overall efficiency. As you continue programming, always aim for clarity when handling exceptions.
For further reading on exception handling in Java, consider checking out Oracle's Java Exception Handling Documentation or the Java Tutorials for detailed insights.
By implementing these best practices, you will bolster your Java programming skills and create more robust applications that can handle errors gracefully and efficiently.