Common Pitfalls in Integer to Short Conversion in Java
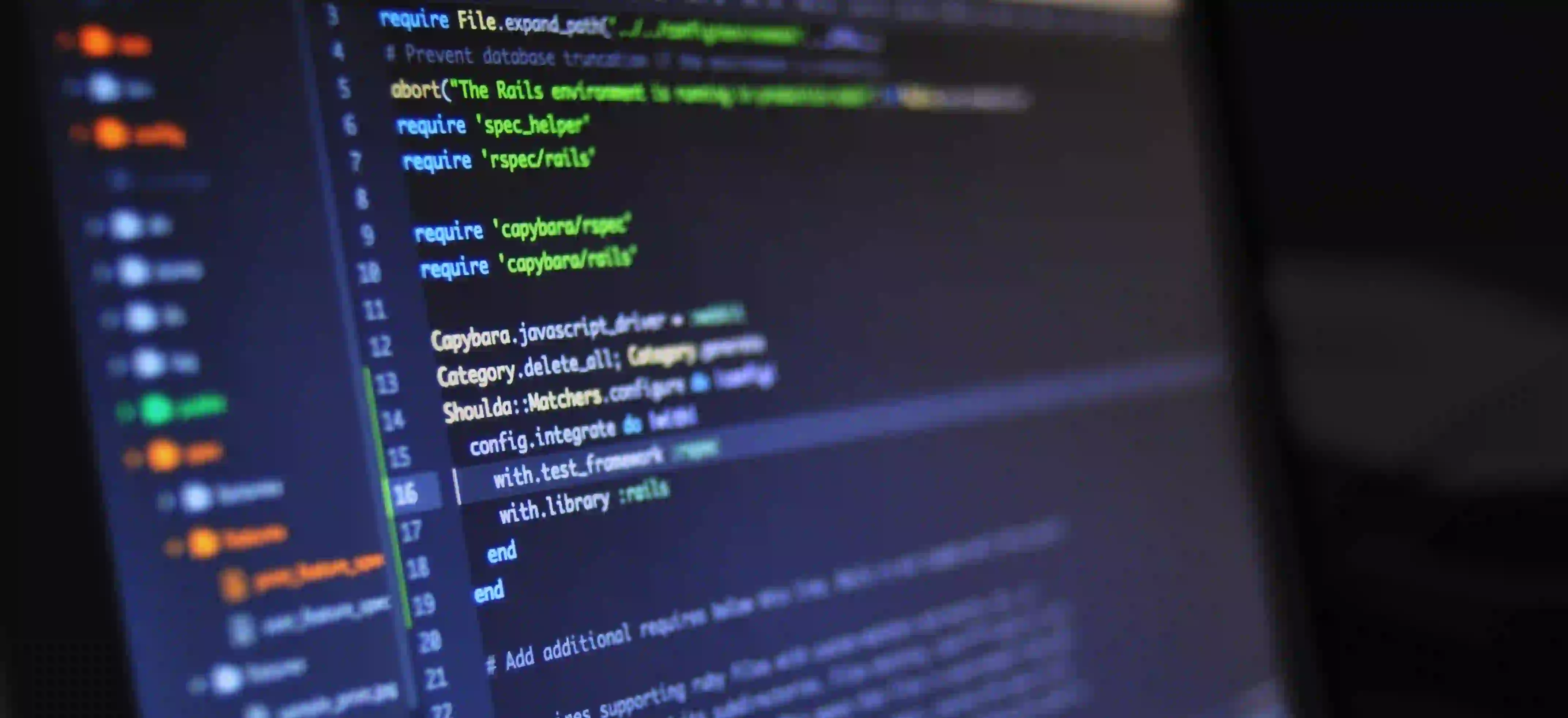
Common Pitfalls in Integer to Short Conversion in Java
When working with Java, you may often need to convert integers to shorts. While it's a straightforward process, there are several common pitfalls that developers might encounter during integer to short conversion. Understanding these pitfalls can help you write more robust and error-free code.
Overview of Integer and Short in Java
Before we dive into the potential pitfalls, let's clarify what integer and short data types are in Java:
- Integer: In Java, the
int
data type is a 32-bit signed integer. It has a wide range (from -2,147,483,648 to 2,147,483,647). - Short: The
short
type is a 16-bit signed integer, with a smaller range (-32,768 to 32,767).
Given this discrepancy in size, direct conversion between these two types isn't safe without considering the possible loss of data.
Pitfall 1: Data Loss Due to Range Limitation
The Problem
The most significant issue arises when an integer exceeds the bounds of a short.
Example
int largeInt = 40000; // A value larger than the max range of short
short convertedShort = (short) largeInt;
System.out.println(convertedShort); // Output: -25536
Commentary
In the above example, the integer value 40000
is converted to a short. However, since 40000
is outside the range of a short, the result becomes -25536
. This occurs due to how the binary representation wraps around.
When converting, you should always check the value or handle the conversion carefully.
if (largeInt < Short.MIN_VALUE || largeInt > Short.MAX_VALUE) {
throw new IllegalArgumentException("Integer value out of range for short");
}
Solution
Always verify the range before performing the conversion. By implementing a range check, you can prevent unexpected behavior in your applications.
Pitfall 2: Implicit vs. Explicit Casting
The Problem
Another common issue is the misconception about casting. Java requires explicit casting when converting from a larger data type to a smaller one.
Example of Implicit Cast
int num = 12345;
short s = num; // Compilation error
Commentary
In the example, the code will not compile because the cast from int
to short
is considered narrowing. However, if you use explicit casting, it works fine.
Correct Way with Casting
int num = 12345;
short s = (short) num; // Explicit casting
Solution
Always use explicit casting when converting from larger to smaller types. This practice makes your intentions clear and helps avoid errors.
Pitfall 3: Overflow During Mathematical Operations
The Problem
Mathematical operations involving shorts that result in values beyond their range can lead to overflow.
Example
short a = 20000;
short b = 15000;
short result = (short) (a + b); // Watch out for overflow!
System.out.println(result); // Output: -15536
Commentary
In this example, adding 20000
and 15000
results in 35000
, which cannot be represented in a short, causing an overflow.
Solution
When performing calculations that could yield results outside the bounds of short, consider using a larger data type, such as int, for the calculations.
int sum = a + b; // Using int for calculation
short result = (short) sum; // Explicitly cast to short the final result
This ensures that you retain the correct values within the operations.
Pitfall 4: Type Inference and Conversational Confusion
The Problem
Type inference can sometimes lead to unexpected conversion behaviors, particularly when using arithmetic operations involving shorts.
Example
short a = 10;
short b = 20;
short result = a + b; // Compilation error
Commentary
In this example, the addition of two shorts promotion happens to int
, and thus the direct assignment back to short without casting leads to a compilation error.
Solution
To solve this, simply cast during assignment after performing the calculation:
short result = (short) (a + b); // Now works fine
By clearly casting the result back to short, you sidestep the compilation error.
Pitfall 5: Using Wrapper Classes
The Problem
When using wrapper classes (Integer
and Short
), auto-unboxing can sometimes lead to NullPointerExceptions
.
Example
Integer integerObject = null;
short shortValue = (short) integerObject; // NullPointerException
Commentary
If you attempt to convert a null
Integer instance to short, it will result in a NullPointerException
.
Solution
Always check that an object is not null before performing conversions:
if (integerObject != null) {
shortValue = (short) integerObject.intValue();
} else {
// Handle null case appropriately
}
The Last Word
Understanding these common pitfalls in integer to short conversion in Java will make you a more effective programmer. By applying the solutions outlined above, you can minimize data loss, avoid unexpected behavior, and write cleaner, more maintainable code.
For more information on type casting in Java, consider checking out Oracle's Casting Documentation and Java Tutorials.
By being aware of these challenges and incorporating preventive measures, you can work with numeric data types in Java confidently, ensuring that your applications run smoothly and reliably. Happy coding!