Maximizing Performance: ForkJoin vs Parallel Streams
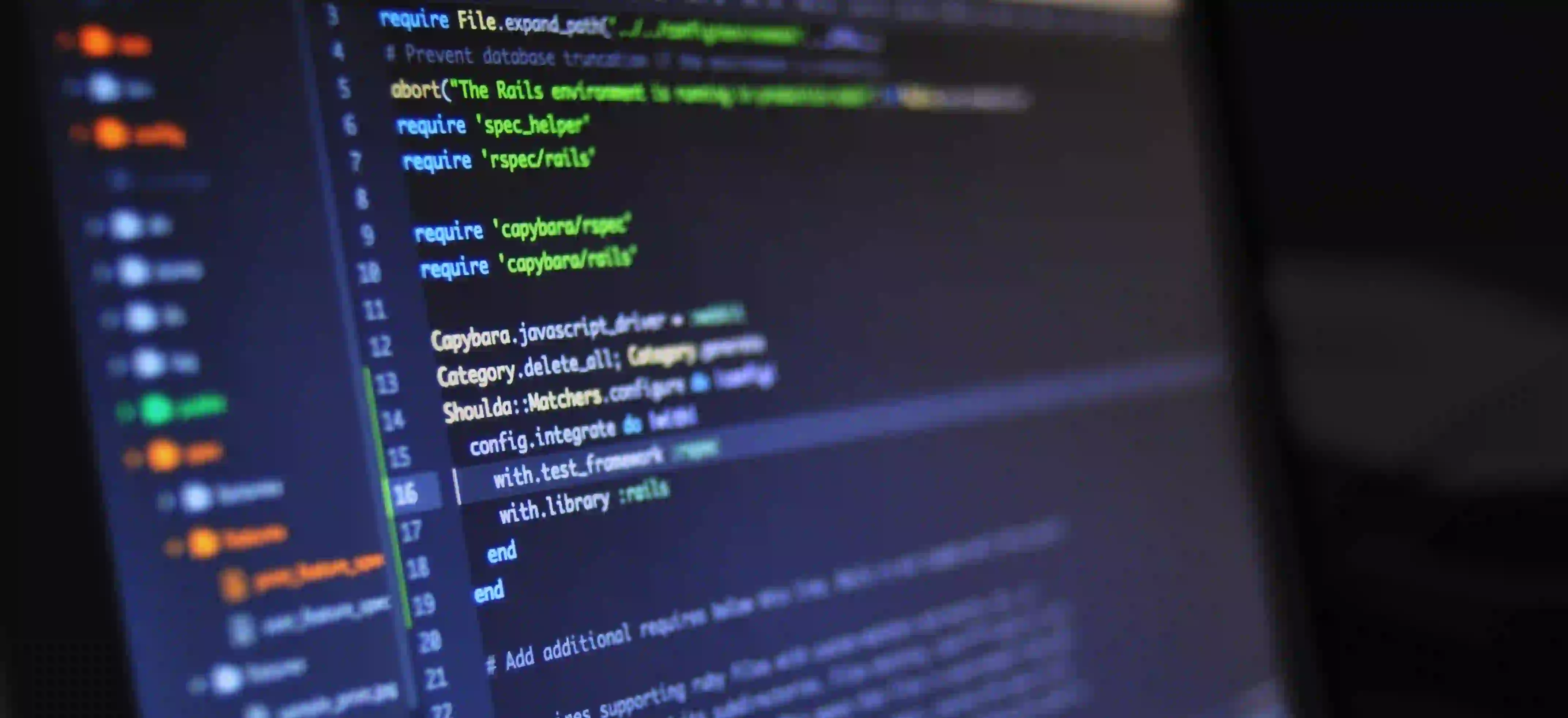
Maximizing Performance: ForkJoin vs Parallel Streams in Java
In modern Java programming, achieving optimal performance in concurrent and parallel processing is a prime concern for developers. The Fork/Join framework and Parallel Streams are two powerful utilities provided by Java to facilitate parallel computing. This article will dive deep into both paradigms, illustrating their differences, advantages, and use cases to help you decide which one to utilize in your projects.
Understanding Fork/Join Framework
What is the Fork/Join Framework?
The Fork/Join Framework was introduced in Java 7 as part of the java.util.concurrent
package. It is designed to take advantage of multi-core processors by enabling the execution of tasks in parallel. The framework divides large tasks into smaller subtasks (hence "fork"), processes these subtasks independently, and then combines the results (hence "join").
Key Components
- ForkJoinPool: The main executor service designed to manage threads and handle the execution of tasks.
- RecursiveTask: A task that returns a result. It is used when you need to compute a result from a given task.
- RecursiveAction: A task that does not return a result. Use it for scenarios where you want to perform some action without needing a value back.
Example Code
Here is a basic implementation using the Fork/Join framework to calculate the sum of an array:
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
public class ForkJoinExample extends RecursiveTask<Integer> {
private final int[] arr;
private final int low;
private final int high;
public ForkJoinExample(int[] arr, int low, int high) {
this.arr = arr;
this.low = low;
this.high = high;
}
@Override
protected Integer compute() {
if (high - low <= 10) { // Threshold of 10 elements
return computeDirectly(); // Sum directly
}
// Split the task
int mid = (low + high) / 2;
ForkJoinExample leftTask = new ForkJoinExample(arr, low, mid);
ForkJoinExample rightTask = new ForkJoinExample(arr, mid, high);
leftTask.fork(); // Fork the left task
int rightResult = rightTask.compute(); // Compute the right task directly
int leftResult = leftTask.join(); // Wait for the left task to complete
return leftResult + rightResult; // Combine the results
}
private Integer computeDirectly() {
int sum = 0;
for (int i = low; i < high; i++) {
sum += arr[i];
}
return sum;
}
public static void main(String[] args) {
int[] arr = new int[1000];
for (int i = 0; i < arr.length; i++) {
arr[i] = i + 1;
}
ForkJoinPool pool = new ForkJoinPool();
ForkJoinExample task = new ForkJoinExample(arr, 0, arr.length);
int result = pool.invoke(task);
System.out.println("Total sum: " + result);
}
}
Why Use Fork/Join?
- Fine-Grained Parallelism: Each task can be further subdivided, allowing for optimal workload distribution.
- Efficient Resource Utilization: It effectively utilizes available CPU cores.
- Flexibility: Fork/Join can optimize various types of computations, not just those that can be easily split.
Understanding Parallel Streams
What are Parallel Streams?
Parallel Streams were introduced in Java 8 and built upon the existing Stream API. They allow processing of sequences of elements in parallel without requiring detailed management of threads by the developer. When a Stream is marked as parallel, the underlying operations get distributed over multiple threads.
Example Code
Here’s how to calculate the sum of an array using Parallel Streams:
import java.util.Arrays;
public class ParallelStreamExample {
public static void main(String[] args) {
int[] arr = new int[1000];
for (int i = 0; i < arr.length; i++) {
arr[i] = i + 1;
}
int sum = Arrays.stream(arr)
.parallel() // Convert the stream to parallel
.sum(); // Sum the elements
System.out.println("Total sum using Parallel Stream: " + sum);
}
}
Why Use Parallel Streams?
- Simplicity: They require less boilerplate code than Fork/Join and allow the developer to focus on high-level problem-solving rather than managing threads.
- Optimized Operations: The Stream API takes care of efficiently dividing and processing tasks internally.
- Declarative Syntax: It promotes a cleaner and more functional style of programming.
Fork/Join vs Parallel Streams: A Comparative Analysis
Performance Considerations
-
Task Granularity:
- Fork/Join: Best when tasks can be moderately fine-grained and recursive in nature.
- Parallel Streams: Ideal for handling larger data sets with operations that can be easily parallelized.
-
Overheads:
- Fork/Join: May incur overhead from managing tasks and threads, but this can be offset by significant parallel gains when the tasks are suitably complex.
- Parallel Streams: They may be less efficient with highly granular operations due to the overhead of maintaining a thread pool.
Ease of Use
- Fork/Join requires more code and design considerations but allows for more customized control over how tasks are broken down and processed.
- Parallel Streams offer a straightforward way to achieve parallel processing without worrying about task decomposition.
Use Cases
- Fork/Join: Use this framework for applications requiring more intricate parallel processing and recursive algorithms, such as sorting, searching large data sets, or complex mathematical computations.
- Parallel Streams: Utilize Parallel Streams for data processing tasks like filtering, mapping, and aggregating collections with less overhead.
Best Practices
- Measure and Benchmark: Always benchmark to analyze performance gains when switching to parallel processing. Tools like JMH (Java Microbenchmark Harness) can assist in accurately measuring performance.
- Don't Force Parallelism: Not all tasks benefit from parallel execution. Use it judiciously when the overhead of task division and coordination is justified by substantial computation savings.
- Understand System Limitations: Monitor thread utilization and CPU load. Parallel processing is limited by available CPU cores, and oversubscription can lead to diminished performance.
To Wrap Things Up
In the realm of concurrent and parallel processing in Java, both the Fork/Join framework and Parallel Streams have their unique advantages and intended use cases. Fork/Join shines when dealing with complex, recursive tasks requiring fine-grained control, while Parallel Streams offer simplicity and performance for bulk operations on collections.
By understanding the nuances and leveraging the right tool for the job, you can significantly enhance the performance of your Java applications. Happy coding!
For further reading, consider exploring:
- Java Concurrency in Practice by Brian Goetz.
- Stream API Documentation for more examples and in-depth details.