Mastering Code Templates in NetBeans: Tackle Common Pitfalls
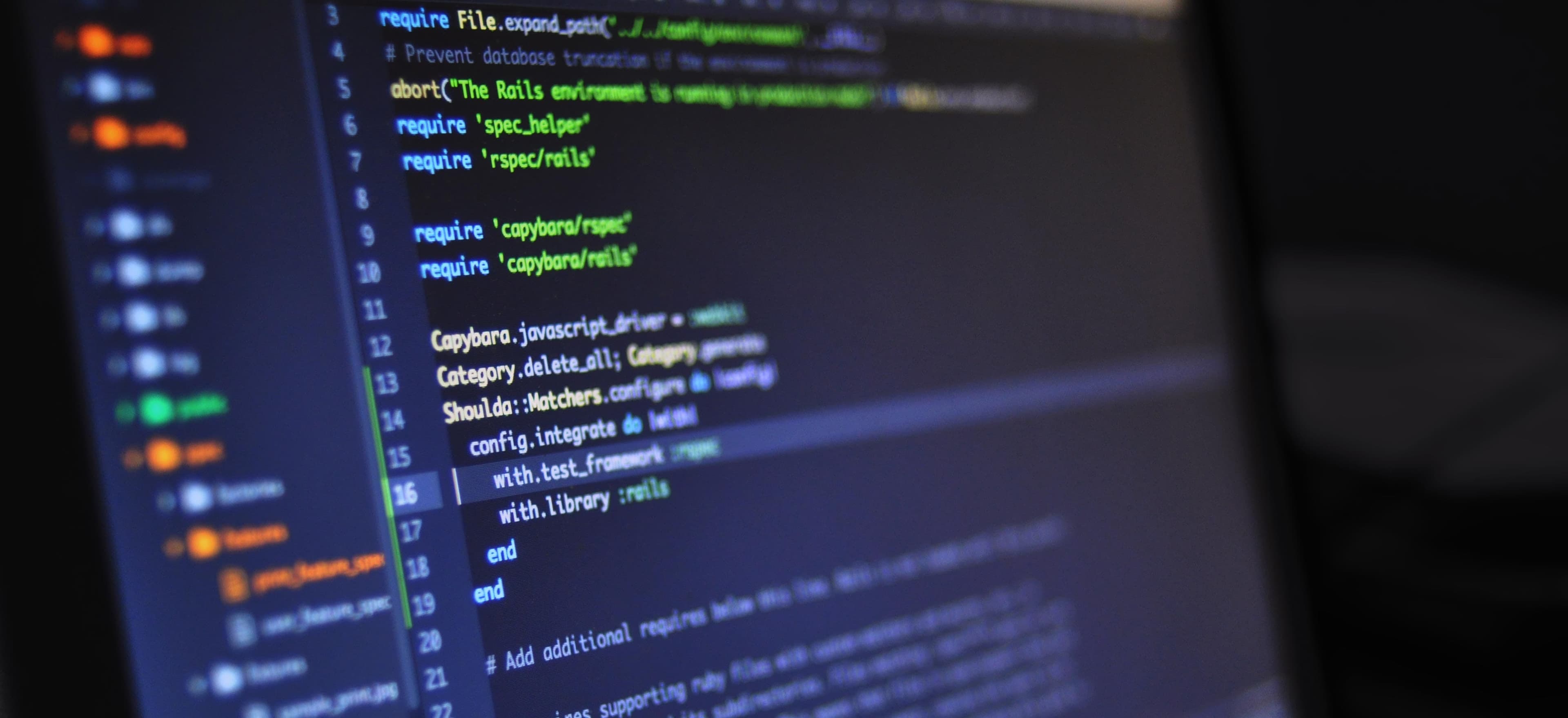
- Published on
Mastering Code Templates in NetBeans: Tackle Common Pitfalls
NetBeans is a powerful integrated development environment (IDE) widely used for Java development. Its extensive features streamline the coding process, making it easier for developers to write, debug, and test their applications. One valuable feature in NetBeans is code templates. These allow you to automate repetitive code snippets, saving time and ensuring consistency. However, like any tool, there are common pitfalls developers can encounter. In this blog post, we will explore how to master code templates in NetBeans and tackle these pitfalls effectively.
What are Code Templates?
Code templates are predefined snippets of code that developers can invoke with a few keystrokes. This feature increases coding efficiency, reduces errors, and fosters better coding practices. In NetBeans, code templates can be as simple as loops or conditions, or as complex as multi-line configurations.
Creating a Simple Code Template
Before diving deep into pitfalls, let’s first create a basic template in NetBeans.
-
Access Templates: Open NetBeans, navigate to Tools > Options > Editor and select the Code Templates tab.
-
Add New Template: Click on the Add button. You will be prompted to enter a name for your new template. Let's say we want to create a template for a simple for-loop.
-
Code Snippet: In the code area, input the following:
for (int ${index} = 0; ${index} < ${limit}; ${index}++) { ${cursor} }
This template allows you to insert a for-loop, where you can quickly replace
${index}
and${limit}
with your desired values. -
AssignShortcut: You can also assign a shortcut by choosing a key combination that you are comfortable with.
Why Use Code Templates?
Using templates like this not only saves time but also promotes consistency across your codebase. By allowing developers to adhere to certain coding styles, you reduce cognitive workload as you switch tasks.
Common Pitfalls of Code Templates
Now that we've covered the basics of creating templates, let’s discuss the pitfalls and how to avoid them.
1. Incomplete Snippets
One of the most frequent issues encountered with code templates is the creation of incomplete or incorrect snippets.
Solution: Always thoroughly test your templates in various scenarios before relying on them. For instance, if your template contains conditional statements, verify that it behaves correctly with different inputs.
if (${condition}) {
// your code here
}
Make sure there are no pieces of code that are open-ended. In the above example, fail to define ${condition}
will lead to issues in execution.
2. Overused Shortcuts
Developers often create shortcuts that are too common, leading to unintentional triggers.
Solution: Choose specific keywords or abbreviations as triggers for your templates. Instead of using a common word like for
, which could invoke your for-loop template accidentally, opt for something like myForLoop
to avoid clashes.
3. Not Utilizing Parameterization
Many fail to take full advantage of parameterization in their templates. With NetBeans, you can make your templates much more dynamic.
Example:
private void ${methodName}(${paramType} ${paramName}) {
${cursor}
}
This template allows you to replace ${methodName}
, ${paramType}
, and ${paramName}
when you invoke it, making it versatile for various methods.
4. Ignoring Readability
When coding quickly, it's easy to overlook the readability of your code templates. While code snippets can be fast, they may lead to maintenance issues later.
Solution: Always structure your templates logically. Consider the following snippet:
List<${type}> ${listName} = new ArrayList<>();
Make sure you include comments and descriptions in the template to remind others (and yourself) of its intended use.
5. Failing to Document
Documentation is often an afterthought in programming. However, neglecting to document your templates can create confusion in your team.
Solution: Include descriptive names and comment your templates where applicable:
// Template for initializing a list
List<${type}> ${listName} = new ArrayList<>();
For Additional Reading
If you're keen on exploring more about Java coding styles or navigating through IDE features, check out these resources:
Advanced Tips for Mastering Code Templates
-
Organize Your Templates: Group your templates into categories. For example, you can have sections for database operations, UI elements, or algorithms. This makes it easier to find the template you need during development.
-
Sharing Templates: If you work in a team, consider sharing your code templates. Establish a shared library, so everyone benefits from consistency in standards and practices.
-
Review and Update Regularly: As your project evolves, so should your templates. Regularly review and update them to satisfy new requirements or best practices.
Closing the Chapter
Mastering code templates in NetBeans can significantly boost your productivity, but it’s crucial to navigate the common pitfalls associated with them. By creating complete, readable, and well-documented templates, leveraging parameterization, and using unique shortcuts, you’ll set the groundwork for an efficient coding experience.
Through intentional practice and adjustment, code templates will become a powerful ally in your Java development toolkit.
Happy coding!
Checkout our other articles