Debugging Docker: Common Pitfalls for Java EE Developers
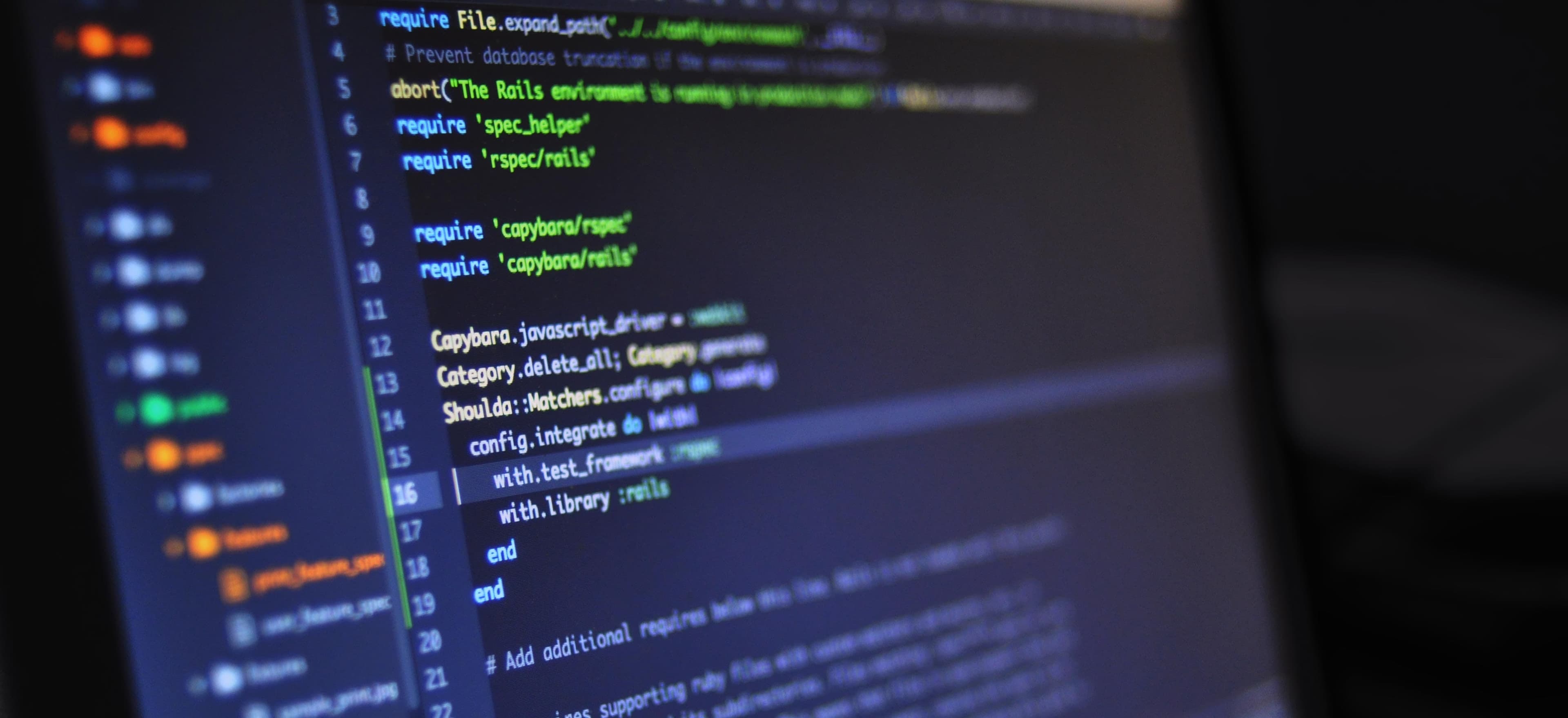
- Published on
Debugging Docker: Common Pitfalls for Java EE Developers
Docker has revolutionized the way software is developed and deployed, offering unparalleled flexibility and significant efficiency gains. For Java EE developers, this integration of Docker into the development workflow can lead to outstanding improvements. However, with these advancements come potential pitfalls, especially during the debugging phase. In this post, we will explore common pitfalls Java EE developers may encounter when debugging applications in Docker and provide solutions to overcome them.
Understanding the Docker Ecosystem
Before diving into specific pitfalls, it's worth understanding the Docker ecosystem. Docker enables developers to package applications along with their dependencies into containers. Each container is isolated, providing consistency across different environments.
Key Docker Concepts:
- Images: Read-only templates to create containers. They contain everything needed to run an application.
- Containers: Running instances of Docker images.
- Dockerfile: A script containing a series of instructions on how to build an image.
For an in-depth understanding of Docker, check out Docker's official documentation.
Common Pitfalls in Debugging Java EE Applications in Docker
1. Misconfigured Environment Variables
One common pitfall is not properly configuring environment variables. Environment variables are often used to configure the application settings in a Java EE application.
Example Issue:
If an application relies on a database URL set via an environment variable but it's not properly defined in the Docker container, this can lead to a failure during runtime.
Solution:
Make sure to specify environment variables in your docker-compose.yml
file or via the Docker CLI:
version: '3'
services:
app:
image: my-java-app
environment:
- DATABASE_URL=jdbc:mysql://db:3306/mydb
By ensuring proper setup, the application can smoothly connect to the database.
2. Inadequate Logging
Java EE applications running in Docker can suffer from inadequate logging. When containers run in isolation, accessing logs isn't straightforward.
Example Issue:
You might find that exceptions are occurring, yet tracing logs inside the container becomes cumbersome.
Solution:
Use Docker's logging features and map the log files outside the container to your local file system. Modify your Dockerfile
to mount a volume:
VOLUME /app/logs
This allows you to inspect log files in a dedicated directory on your host machine. Moreover, consider integrating a centralized logging system like ELK Stack (Elasticsearch, Logstash, Kibana) for better log management.
3. Incorrect Networking Configuration
Docker containers communicate over networks. Misconfigured networks can lead to communication issues between your Java EE application and other services, such as databases or microservices.
Example Issue:
If your application cannot reach the database service due to incorrect network settings, you will face significant roadblocks.
Solution:
Define appropriate network configurations in docker-compose.yml
:
version: '3'
services:
app:
networks:
- mynetwork
db:
networks:
- mynetwork
networks:
mynetwork:
Using defined networks ensures that containers can communicate with each other seamlessly.
4. Overlooking the Container's Lifespan
Another common oversight is manipulating long-running applications versus short-lived processes. Java EE applications, ideally housed in application servers like WildFly or TomEE, should maintain their state across requests.
Example Issue:
Restarting a container will reset the application state, potentially leading to lost user sessions or cached data.
Solution:
You can utilize Docker's feature of persistent storage. By mounting volumes, you can store the application state separately from the container lifecycle.
services:
app:
volumes:
- myapp_data:/app/data
volumes:
myapp_data:
Leveraging volumes ensures that important data persists even when containers are stopped or restarted.
5. Inefficient Resource Handling
Java EE applications can be resource-intensive, and inefficient resource handling can lead to performance issues. When running in Docker, it is crucial to allocate resources properly.
Example Issue:
You may encounter OutOfMemory errors if the container is configured with inadequate memory limits.
Solution:
Make sure to define resource limits in your Docker configuration.
services:
app:
deploy:
resources:
limits:
cpus: '0.5'
memory: 512M
This setup helps manage how much CPU and memory your application can access, improving stability under load.
6. Ignoring the Benefits of Multi-Stage Builds
Java developers often use build tools like Maven or Gradle to package applications. Sticking to single-stage builds leads to larger images, possibly containing unnecessary files.
Example Issue:
Large images take longer to build and pull, leading to inefficient deployment processes.
Solution:
Use Docker's multi-stage build feature to reduce image sizes by separating the build phase from the runtime phase.
# First Stage: Build
FROM maven:3.6.3-jdk-11 as builder
WORKDIR /app
COPY . .
RUN mvn clean install
# Second Stage: Run
FROM openjdk:11
COPY --from=builder /app/target/my-java-app.jar /app/my-java-app.jar
CMD ["java", "-jar", "/app/my-java-app.jar"]
This approach results in smaller final images and faster deployments.
The Bottom Line
Debugging Dockerized Java EE applications can be fraught with challenges. However, by understanding common pitfalls and how to mitigate them, developers can significantly improve their development workflows and application reliability.
Incorporating proper logging, configuring environment variables, ensuring effective resource management, and minimizing image sizes will not only aid in debugging but also enhance the performance of applications running in Docker containers.
For further reading, consider these resources:
- The Twelve-Factor App for best practices in building applications.
- Docker Official Documentation, a vital resource for new and seasoned developers alike.
By mastering these aspects, Java EE developers can ensure their applications run smoothly in Docker, leading to a more efficient and effective development lifecycle. Happy coding!
Checkout our other articles