Troubleshooting Common Issues When Creating Your First Servlet
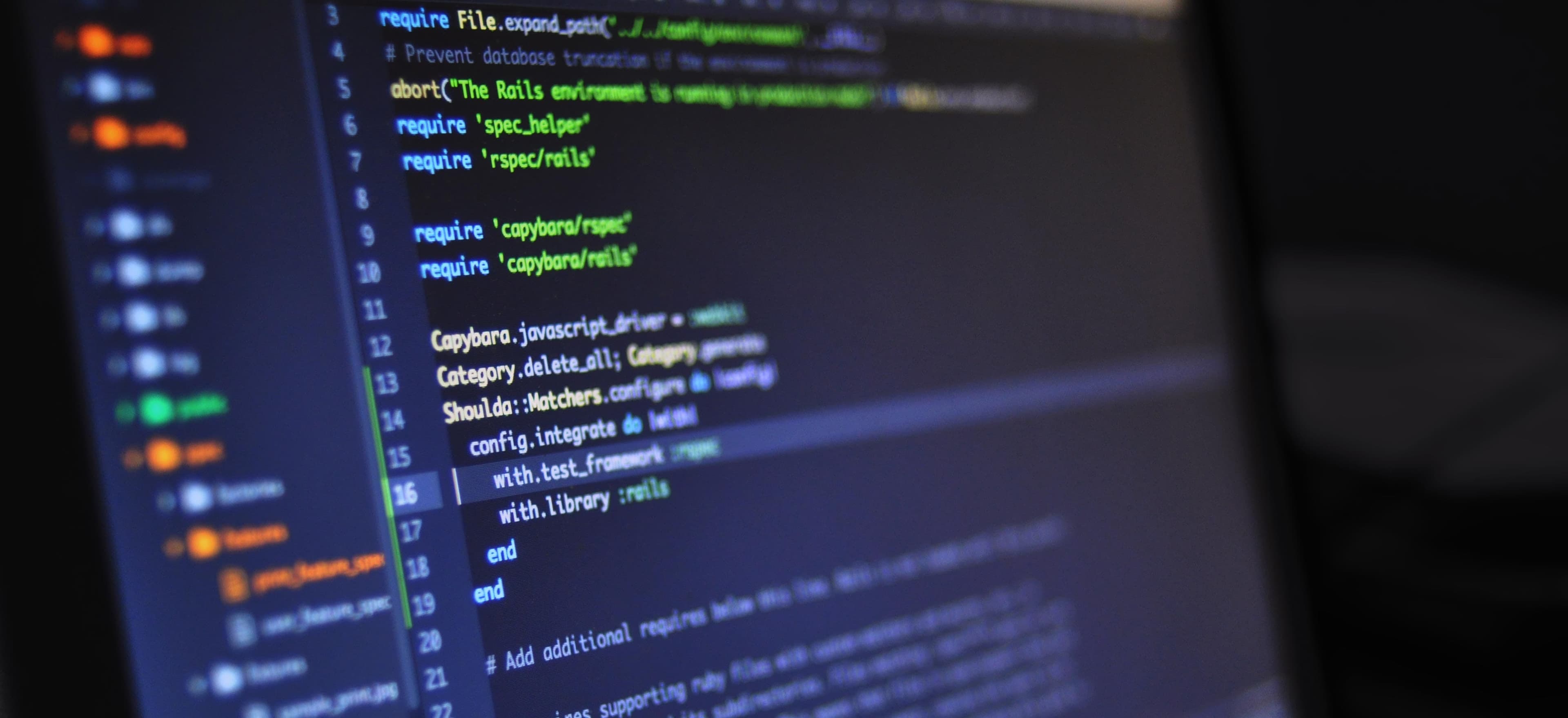
- Published on
Troubleshooting Common Issues When Creating Your First Servlet
Creating a servlet for the first time can be an exhilarating journey into the world of Java web applications. However, like all adventures, it can also come with its fair share of challenges. In this blog post, we'll delve into some common issues you might face when developing your first servlet, and we'll provide straightforward solutions to help you overcome them.
What is a Servlet?
Before we dive into troubleshooting, let's briefly summarize what a servlet is. A servlet is a Java program that runs on a server and extends the capabilities of a web server. It can respond to requests from web clients, usually web browsers, by processing the request and generating dynamic content such as HTML or JSON.
Setting Up the Environment
To get started with servlets, ensure that you have:
- The Java Development Kit (JDK) installed.
- A servlet container like Apache Tomcat.
- An IDE like Eclipse or IntelliJ IDEA to simplify coding.
Common Issues and Solutions
Now, let’s discuss some of the most common issues developers face when creating their first servlet.
1. Servlet Not Found (404 Error)
The most common issue beginner servlet developers encounter is the dreaded 404 error. This usually indicates that the server could not find the servlet you deployed.
Solution:
Make sure to configure your web.xml
correctly. This file is in the WEB-INF
directory of your project and is where you define the servlet mappings.
Here’s an example of how to map a servlet:
<servlet>
<servlet-name>MyFirstServlet</servlet-name>
<servlet-class>com.example.MyFirstServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>MyFirstServlet</servlet-name>
<url-pattern>/hello</url-pattern>
</servlet-mapping>
In this example, the servlet can be accessed via the URL pattern /hello
. If you change the name or the url-pattern, ensure these match in both the servlet definition and your request URL.
2. Class Not Found (ClassNotFoundException)
This error occurs when the servlet class cannot be found by the server.
Solution:
Make sure that your compiled servlet classes are in the correct location. Typically, compiled classes should be placed in the WEB-INF/classes
directory, mirroring your package structure.
Let’s say you have a com.example
package. The compiled class would be located at WEB-INF/classes/com/example/MyFirstServlet.class
.
Example Servlet Code
Here is a simple example servlet that displays "Hello, World!" in a web browser.
package com.example;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/hello")
public class MyFirstServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
response.getWriter().println("<h1>Hello, World!</h1>");
}
}
Commentary:
- The
@WebServlet
annotation simplifies the configuration by eliminating the need forweb.xml
configuration. It makes the servlet accessible at the/hello
URL. - In the
doGet
method, we set the content type of the response and write a simple HTML string to the output.
3. Server Port Issues
Sometimes, servers don't start properly due to port conflicts. If another service uses the default port used by your servlet container (usually 8080 for Tomcat), you won’t be able to deploy your servlet.
Solution:
Check if any other applications are using the port. You can change the port in the server.xml file in Tomcat under the conf
directory.
<Connector port="8080" protocol="HTTP/1.1"
connectionTimeout="20000"
redirectPort="8443" />
Simply change the port value to something else, like 8081, and restart your server.
4. Compilation Issues
You might face problems with compiling your servlet code, often due to missing libraries or incorrect Java version settings.
Solution:
Ensure you include the necessary servlet API libraries in your project’s build path. If you're using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
This allows you to utilize the servlet classes without packaging them with your application.
5. Deployment Descriptor Issues
If you find that your servlet isn’t executing even after confirming correct mapping, consider the deployment descriptor.
Solution:
Check for typos or incorrect XML structure in your web.xml
. XML syntax is unforgiving; even a misplaced tag can cause issues. Use an XML validator to ensure correctness.
Testing Your Servlet
To test your servlet, ensure that the server is running, and navigate to http://localhost:8080/your-web-app-name/hello
in your browser. If everything is set up correctly, you should see "Hello, World!" displayed on your screen.
Additional Resources
While this post provides an overview of common issues related to servlet development, you can find more thorough explanations and examples by checking out the following resources:
- Official Java EE Documentation
- Apache Tomcat Documentation
The Last Word
Building your first servlet can be daunting, but facing and overcoming these common issues will help you gain invaluable experience. Remember to pay close attention to your configurations and always test thoroughly. With practice, you’ll be able to create complex web applications using servlets with ease.
Happy coding!
Checkout our other articles