How Impure Functions in JavaScript Affect Your Java Apps
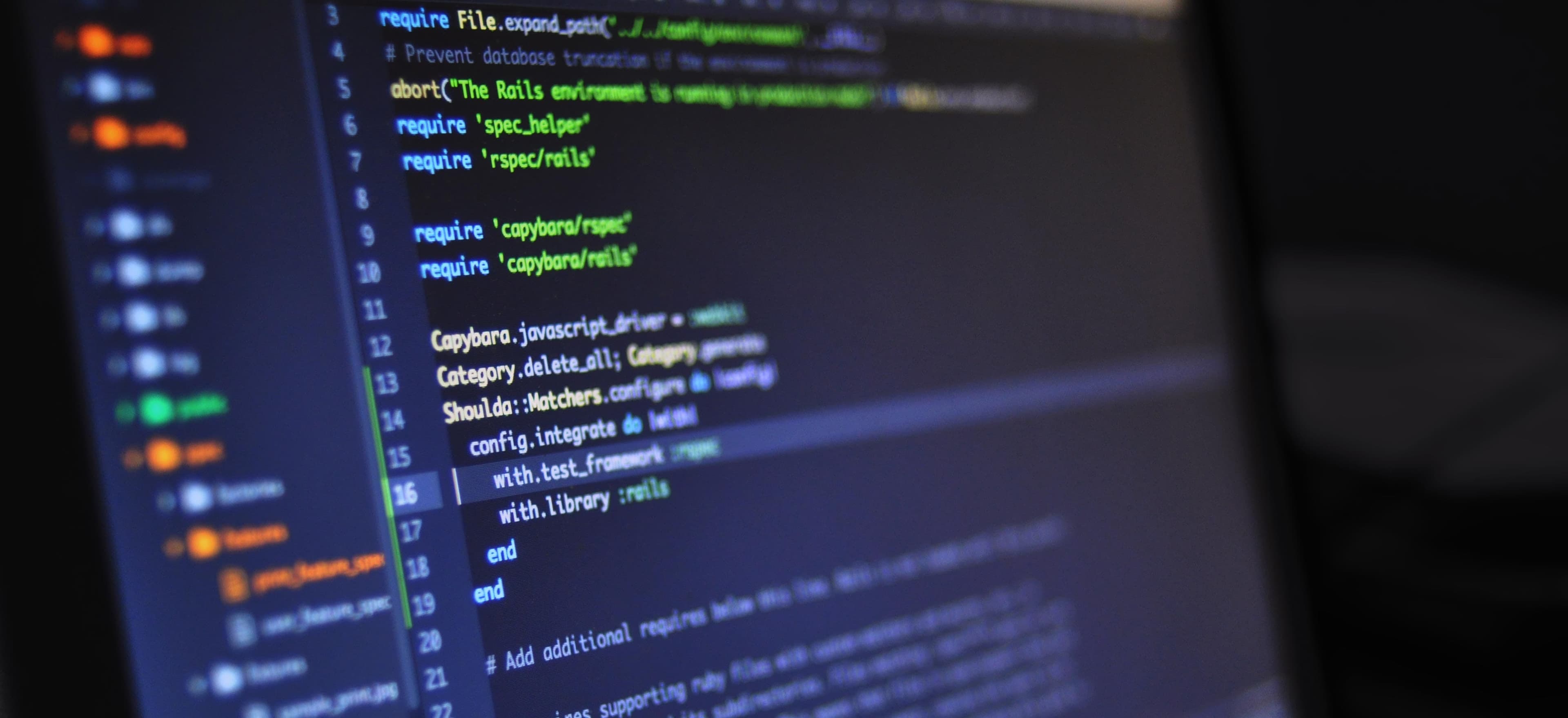
- Published on
How Impure Functions in JavaScript Affect Your Java Apps
Java and JavaScript are distinct programming languages with different paradigms. Java is known for its strict type system and object-oriented features, while JavaScript is more flexible and is often associated with web development environments. However, developers increasingly leverage both languages in modern development ecosystems. Understanding the implications of function purity in JavaScript is crucial, especially as Java applications now frequently interface with JavaScript through frameworks like Node.js or by using APIs.
In this article, we'll explore how impure functions in JavaScript can lead to unexpected issues when interacting with Java-based applications. To start, let's define what we mean by impure functions.
What Are Impure Functions?
An impure function is one that has side effects or relies on external state. This contrasts with pure functions, which consistently return the same output for the same input and do not alter any external state.
Example of an Impure Function in JavaScript
let count = 0;
function increment() {
count++;
return count;
}
console.log(increment()); // 1
console.log(increment()); // 2
In the example above, the increment
function is impure because it modifies the external variable count
. Each time the function is called, it alters the external state, which can lead to unpredictable outcomes if not managed carefully.
The Risks of Impure Functions
The problems with impure functions surface in several ways:
-
Unpredictable Behavior: If the same input does not always produce the same output, debugging becomes incredibly challenging. This unpredictability can contribute to bugs in both JavaScript and Java environments.
-
State Management Chaos: Complex applications often involve multiple states. When impure functions alter states, it can lead to confusion about where and why changes are occurring.
-
Interoperability Issues: When Java communicates with JavaScript, surprises might await. If JavaScript impure functions update state or variables passed from Java, it can lead to out-of-sync behavior that Java can't easily anticipate or handle.
For a real-world context on the implications of impure functions, check out the article titled Why Impure Functions Can Lead to JavaScript Bugs, which highlights the common pitfalls developers encounter when using impure functions in JavaScript.
The Java and JavaScript Interface
When building Java applications that involve JavaScript, such as using frameworks like Spring Boot with Thymeleaf or Angular for front-end, developers must recognize the potential pitfalls arising from JavaScript's flexibility — primarily its use of impure functions.
Example Interaction Between Java and JavaScript
Let's consider a simple example where a Java API is called by a JavaScript front end to fetch user data.
Java Code:
@RestController
public class UserController {
@GetMapping("/user/{id}")
public User getUser(@PathVariable("id") String id) {
return userService.findUserById(id);
}
}
This Spring Boot endpoint fetches data using an id
. In a well-structured architecture, this data would remain unchanged unless explicitly modified by a call. However, suppose you have the JavaScript side pushing data to a UI, where an impure function modifies user states.
JavaScript Code:
let userState = {};
function updateUser(data) {
// Impure function: modifies external variable
userState[data.id] = data;
}
fetch('/user/1')
.then(response => response.json())
.then(user => updateUser(user));
In this case, if the updateUser
method were to call itself based on a timer or user action elsewhere, the userState would not reliably represent the current state of the user. Cross-pollination issues between Java and JavaScript arise, affecting state integrity in a potentially fractured user experience.
Strategies to Mitigate Issues
To handle these potential issues with JavaScript impure functions affecting Java applications, consider the following strategies:
1. Adopt Functional Programming Principles
Leverage functional programming patterns in your JavaScript coding practices. By favoring pure functions, you can generate consistent outputs and lessen unpredictability.
2. Encapsulate State Management
Utilizing powerful state management tools, such as Redux or MobX, can help centralize state in a way that remains predictable and modifiable in a controlled manner.
3. Write Integration Tests
Integration testing can reveal how impure functions are affecting your Java code. Since impure functions can create side effects, testing can help verify that the state remains as expected.
4. Clear Documentation
Clearly document the interactions between Java and JavaScript. This allows teams to understand where state changes occur and to identify which functions might cause unexpected behavior.
5. Use API Contracts
Create clear contracts for the API interfaces between Java and JavaScript. If a Java function expects data in a certain format, ensure the JavaScript adheres strictly to this structure to avoid and spot unexpected inputs early on.
Lessons Learned
The interactions between JavaScript and Java can lead to powerful applications. However, understanding how impure functions in JavaScript can negatively affect Java apps is a crucial aspect of modern development. By following best practices and maintaining clear communication between the two languages, we can safeguard against unpredictable behaviors, helping to foster reliability and increase code quality.
By recognizing and mitigating the risks posed by impure functions, you become not just a better Java or JavaScript developer, but a more holistic programmer, aware of the nuanced intricacies that make modern software development a challenging yet exciting field.
Explore more about the nuances and challenges of JavaScript development in the detailed article titled Why Impure Functions Can Lead to JavaScript Bugs.
With proper understanding and cautious practices, you can leverage both languages efficiently, thus widening your application scope while keeping bugs at bay. Happy coding!
Checkout our other articles