Avoid These Java Setup Mistakes for Smooth Development
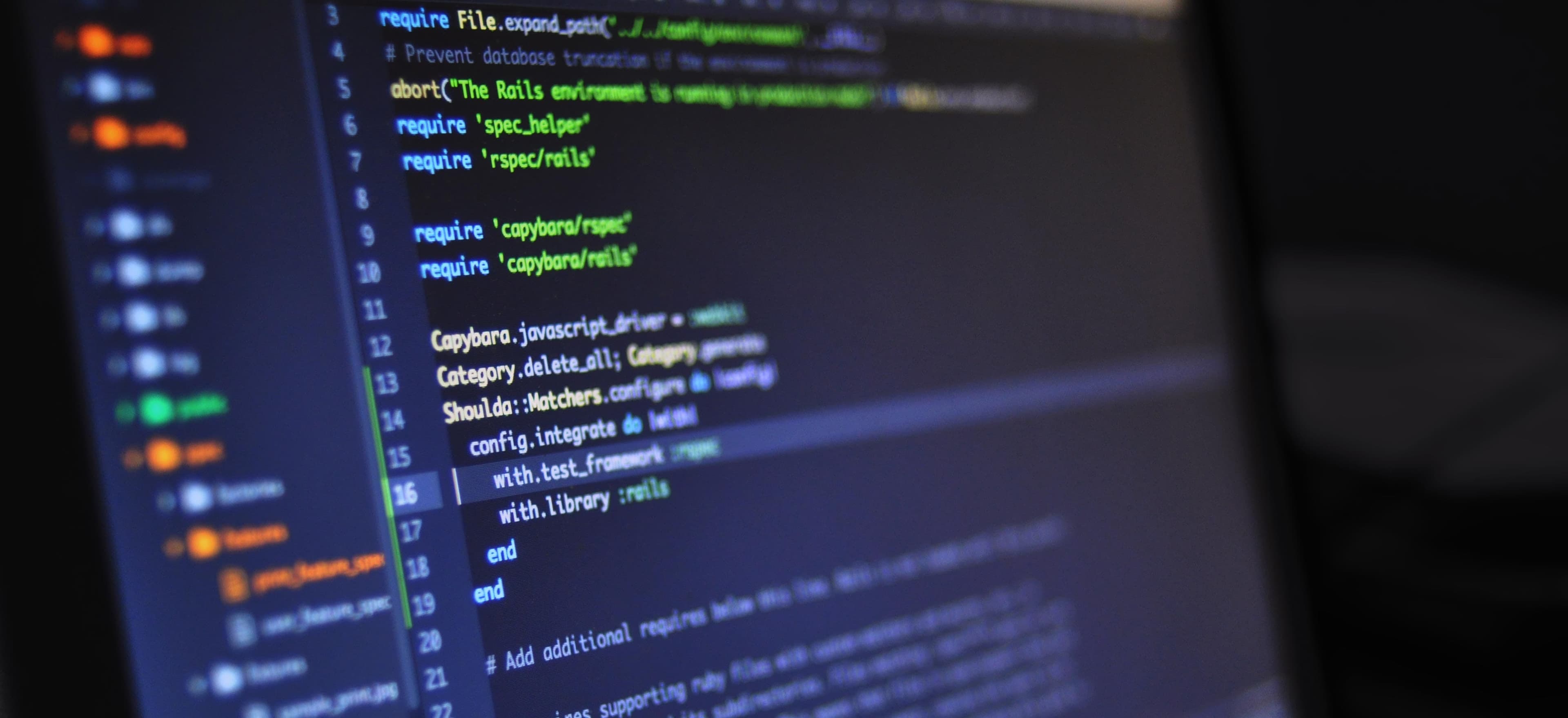
- Published on
Avoid These Java Setup Mistakes for Smooth Development
Setting up a Java development environment may seem straightforward, particularly for seasoned developers. However, the reality is that there are common missteps that, if ignored, can lead to frustration and wasted time down the line. In this blog post, we'll explore these pitfalls and provide practical tips for establishing a robust Java setup.
Before we dive in, you might want to check out 5 Common Pitfalls When Setting Up Your Dev Machine. This article outlines several critical setup errors that many developers overlook.
1. Ignoring Version Control
One of the first mistakes developers often make is neglecting version control systems (VCS). Not utilizing a VCS can lead to disorganized codebases and ineffective collaboration among team members.
Why Use Version Control?
Version control allows you to track changes, revert to previous versions, and collaborate seamlessly. Git is the most popular system for Java development. Here's a simple setup to initialize a Git repository:
# Navigate to your Java project directory
cd /path/to/your/java/project
# Initialize a new Git repository
git init
# Add your files to be tracked
git add .
# Commit your changes
git commit -m "Initial commit"
Commentary
By following these steps, you ensure every change is tracked. Think of this as a safety net. Should anything go wrong in the future, you can easily roll back to a stable version.
2. Mismanaging JDK and IDE Configurations
Another prevalent issue lies in mismanaging the Java Development Kit (JDK) and Integrated Development Environment (IDE). Each IDE has its process for selecting the JDK. For instance, if you are using IntelliJ IDEA, you can set the JDK by navigating to File → Project Structure → Project.
Setting JAVA_HOME
It's equally essential to set your JAVA_HOME environment variable to point to your JDK installation. This practice helps various tools recognize your Java environment.
# For Linux/Mac
export JAVA_HOME=/path/to/jdk
# For Windows
set JAVA_HOME=C:\path\to\jdk
Commentary
Failing to configure JDK correctly can lead to compilation errors and incompatibility issues. Additionally, ensuring that your IDE matches the JDK version is critical for taking advantage of new language features and optimizations.
3. Overlooking Dependency Management
In modern software development, managing dependencies effectively is paramount. Java projects often rely on various libraries. Not using a dependency management tool can lead to version conflicts and bloated libraries.
Using Maven
Maven is a popular build automation tool that can help manage dependencies. Here’s a basic pom.xml
configuration to get started:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>your.group.id</groupId>
<artifactId>your-artifact-id</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.8</version>
</dependency>
</dependencies>
</project>
Commentary
By managing dependencies effectively, you can maintain a cleaner, more organized project. Tools like Maven automatically handle transitive dependencies and ensure that you have the right versions installed.
4. Not Setting Up an Effective Build Process
A failing or absent build process is a pitfall that can impede productivity. Tools such as Maven and Gradle allow for streamlined builds, including testing and deployment.
Using Gradle
Here’s a simple Gradle build script (build.gradle
):
plugins {
id 'java'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.apache.commons:commons-lang3:3.12.0'
}
test {
useJUnitPlatform()
}
Commentary
Having an effective build process means that you can automate mundane tasks while ensuring consistency across builds. Continuous Integration/Continuous Deployment (CI/CD) pipelines can leverage this setup for seamless deployments.
5. Ignoring Effective Logging
Java applications can become complex, making it challenging to trace issues. By neglecting effective logging practices, you risk losing valuable debugging information.
Using SLF4J with Logback
Implementing SLF4J with Logback, a logging framework, allows for streamlined and maintainable logging. Here’s how to set it up:
- Include SLF4J and Logback dependencies in your
pom.xml
:
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.2.3</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
- Example of using SLF4J in your Java class:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyApp {
private static final Logger logger = LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
logger.info("Application Started");
try {
// Your code logic here
} catch (Exception e) {
logger.error("An error occurred", e);
}
}
}
Commentary
Effective logging allows you to monitor your application and diagnose issues efficiently. You can easily keep track of important events, errors, and application states.
6. Failing to Document Your Setup
Many developers underestimate the importance of documentation. Proper documentation eases the onboarding process for new team members and reduces confusion.
How to Document Efficiently
Create a README.md
file within your project directory detailing the setup instructions, dependencies, and configuration settings. Here’s an example structure:
# Project Title
## Getting Started
### Prerequisites
- JDK 11+
- Maven
### Setup Instructions
1. Clone the repository
2. Install Maven dependencies
3. Build the project
Commentary
Good documentation ensures continuity. It allows anyone to set the project up quickly, even if they are new or if the project maintains new contributors.
Closing Remarks
Establishing a solid Java development environment is crucial for efficiency and productivity. By avoiding these common pitfalls— version control neglect, mismanaging JDK and IDE configurations, poor dependency management, lack of an effective build process, ineffective logging, and failing to document—you set the foundations for a smooth development experience.
For further insights on setting up your development environment, don't forget to check out the previous post on 5 Common Pitfalls When Setting Up Your Dev Machine.
By carefully considering these aspects, you will create a more robust and efficient development workflow that allows you to focus on what really matters: writing great code.
Checkout our other articles