Making Java Applications Accessible: Tips for Developers
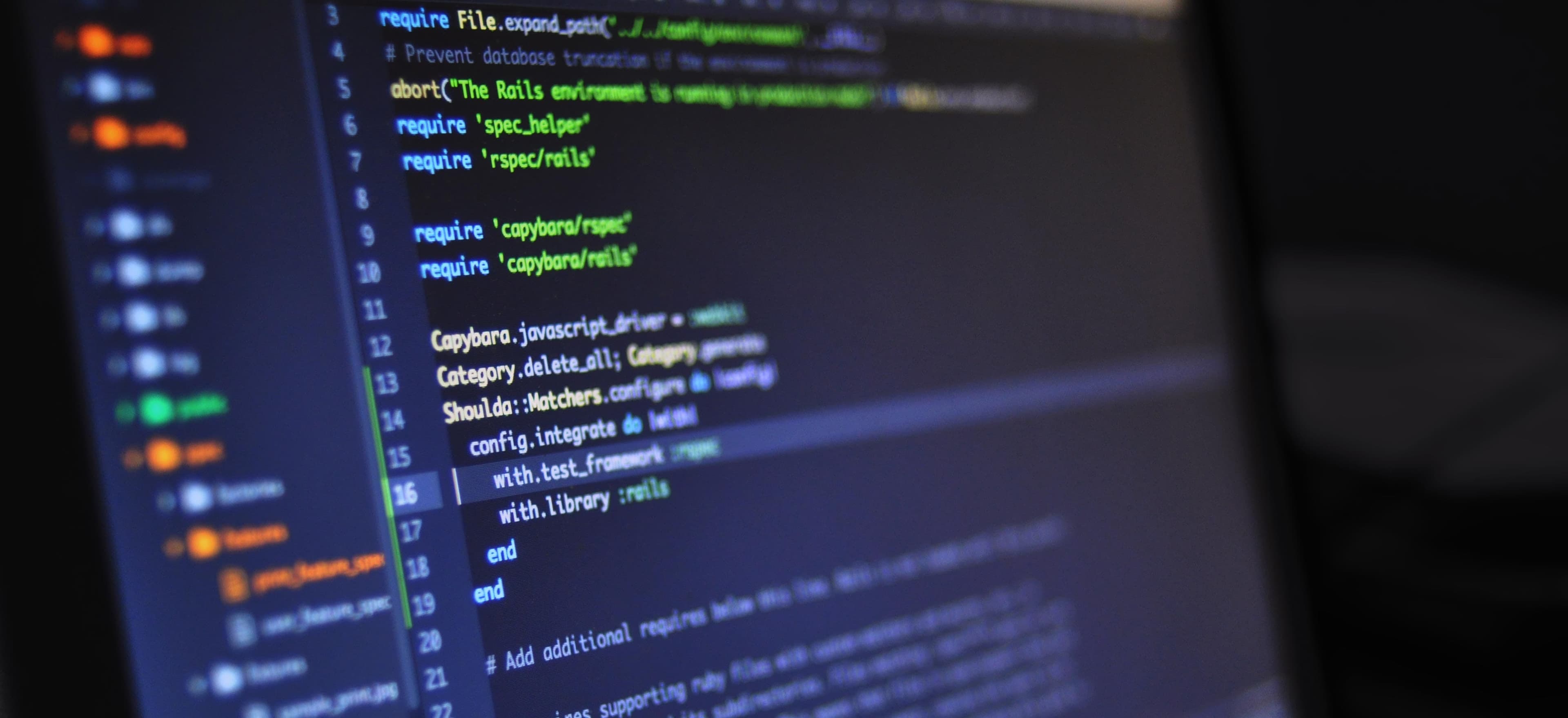
- Published on
Making Java Applications Accessible: Tips for Developers
In the digital age, accessibility is not just a nicety; it’s a necessity. For developers, ensuring that applications are usable by people with disabilities should be a primary concern. According to the article "Breaking Barriers: Enhancing Website Accessibility Now", inclusive design not only serves those who need assistance but improves the overall user experience for everyone. In this blog post, we will explore how Java developers can create accessible applications, as well as tips and strategies to implement accessibility effectively.
Understanding Accessibility in Java Applications
Accessibility means that applications are designed to be usable by people of all abilities and disabilities. This includes individuals with visual impairments, hearing disabilities, cognitive limitations, and physical disabilities. In Java applications, accessibility is often achieved through the implementation of various standards, including the Web Content Accessibility Guidelines (WCAG) and Section 508 of the Rehabilitation Act.
Why Accessibility Matters
-
Legal Compliance: Many jurisdictions require digital accessibility. Non-compliance can lead to legal implications.
-
Wider Audience: Making your application accessible expands your user base. With over 1 billion people worldwide experiencing some form of disability, accessibility can significantly impact your market reach.
-
Better User Experience: Accessible applications often provide a more usable experience for everyone, not just users with disabilities.
Practical Tips for Enhancing Accessibility in Your Java Applications
1. Use Java Accessibility API
The Java Accessibility API provides a framework for building accessible Java applications. By utilizing this API, you can expose the properties of GUI components (like buttons or text fields) to assistive technologies.
Code Example
Here is how to implement the Java Accessibility API in your application:
import javax.accessibility.Accessible;
import javax.accessibility.AccessibleContext;
import javax.accessibility.AccessibleRole;
import javax.swing.JButton;
public class AccessibleButton extends JButton {
@Override
public AccessibleContext getAccessibleContext() {
AccessibleContext context = super.getAccessibleContext();
if (context == null) {
context = new AccessibleButtonContext();
}
return context;
}
protected class AccessibleButtonContext extends AccessibleAbstractButton {
@Override
public AccessibleRole getAccessibleRole() {
return AccessibleRole.PUSH_BUTTON;
}
}
}
Why this matters: By implementing the Accessible
interface, you make it easier for screen readers and other assistive technologies to interact with your components. This enhances the user experience for those who rely on these technologies.
2. Provide Text Alternatives
Always provide alternative text for images and icons in your application. This can be accomplished using the setAccessibleDescription()
method.
Code Example
JButton myButton = new JButton(new ImageIcon("icon.png"));
myButton.setAccessibleDescription("This button opens the settings menu");
Why this matters: Text alternatives ensure that users who cannot see images will still understand the button's function, effectively removing barriers to information access.
3. Use Clear and Descriptive Labels
Make sure all interactive components have clear, descriptive labels. This is especially vital for form fields and buttons.
Code Example
JTextField nameField = new JTextField();
nameField.setAccessibleName("Name Input Field");
nameField.setToolTipText("Please enter your full name");
Why this matters: Clear labels help users understand the purpose of interactive elements. This is particularly crucial for users with cognitive disabilities.
4. Ensure Keyboard Navigation
Many users rely on keyboard navigation to interact with applications. Ensure that all components can be accessed using a keyboard alone.
Code Example
myButton.setFocusable(true); // Ensure the button can receive focus via keyboard
myButton.addActionListener(e -> {
// Button action here
});
Why this matters: By enabling keyboard navigation, you cater to users who may not be able to use a mouse due to motor difficulties.
5. Maintain Color Contrast
Ensure that text and background colors have sufficient contrast. Use tools like the WebAIM color contrast checker to verify this.
Code Example
JPanel panel = new JPanel();
panel.setBackground(Color.WHITE);
JLabel label = new JLabel("Accessible Text");
label.setForeground(Color.BLACK);
panel.add(label);
Why this matters: Poor contrast can make text unreadable for users with vision impairments. A good contrast ratio helps ensure that your text is legible.
6. Use Accessible Layout Managers
When laying out your user interface, use layout managers that are designed for accessibility. Avoid absolute positioning, which can lead to unpredictable results in assistive technologies.
Code Example
JPanel panel = new JPanel(new GridLayout(2, 2));
panel.add(new JLabel("Label 1:"));
panel.add(new JTextField(10));
panel.add(new JLabel("Label 2:"));
panel.add(new JTextField(10));
Why this matters: Layout managers automatically adapt the layout based on the elements you include. This compatibility enhances the overall experience for users accessing the interface with assistive technologies.
7. Test Your Application for Accessibility
Testing for accessibility is an ongoing process. Utilize both automated tools and human testers to evaluate your application’s accessibility.
Consider integrating tools like:
-
Accessibility Testing Tools: Such as Axe or Wave, which provide feedback on web accessibility.
-
Screen Readers: Test your applications with screen readers like NVDA or JAWS to identify areas of improvement.
Why this matters: Continuous testing helps identify and rectify potential accessibility issues, ensuring that your application remains inclusive.
Final Thoughts
Enhancing accessibility is not merely about compliance; it's about creating a better experience for all users. By following the tips and best practices outlined above, Java developers can create applications that are truly accessible.
For more in-depth insights into accessibility and its importance, check out the article "Breaking Barriers: Enhancing Website Accessibility Now".
Accessibility should be at the forefront of our efforts in software development, not an afterthought. As developers, we hold the responsibility to build applications that everyone can enjoy and utilize effectively. Let’s break barriers together, one line of code at a time!
Checkout our other articles