Mastering Java: Find the Biggest of Three Numbers Easily
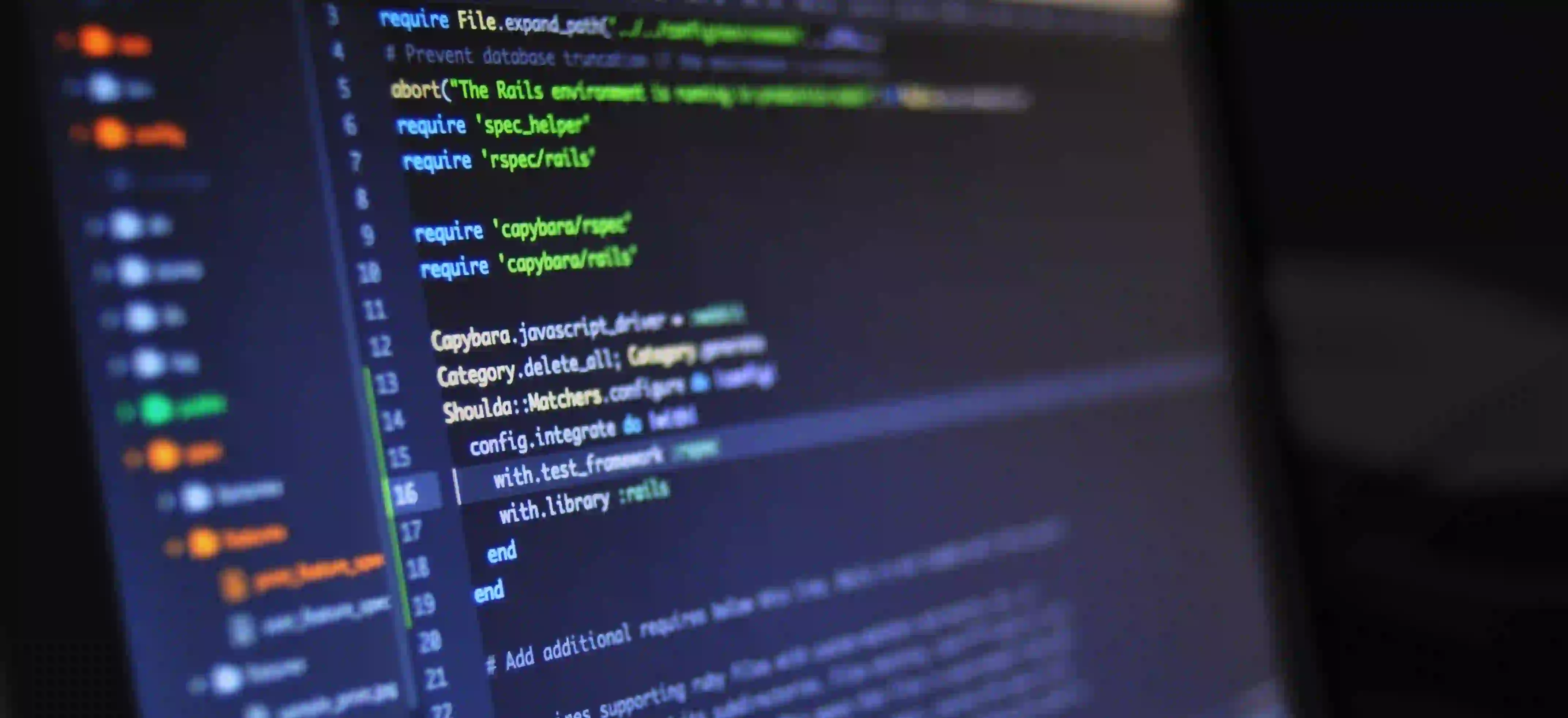
Mastering Java: Find the Biggest of Three Numbers Easily
Java has long been one of the most popular programming languages for developers worldwide. Its versatility and robustness make it ideal for various applications, from web development to mobile apps. In this blog post, we will focus on a straightforward yet fundamental exercise: finding the biggest of three numbers in Java.
This task may seem simple, but it encapsulates numerous essential concepts in Java programming. From conditional statements to basic input/output operations, this exercise will serve as a building block for more complex scenarios.
Why Learning How to Compare Numbers is Important
Understanding how to compare numbers is foundational in programming. Real-world applications often require making decisions based on different inputs. Whether it's selecting the highest score in a game or filtering out the maximum temperature from a weather dataset, knowing how to evaluate numbers against each other is crucial.
Setting Up Your Java Environment
Before diving into the code, ensure you have the following tools and environment:
- Java Development Kit (JDK): Make sure you have JDK 11 or newer installed. You can download it from Oracle's official website.
- IDE or Text Editor: You can use an Integrated Development Environment (IDE) like IntelliJ IDEA, Eclipse, or a simple text editor like Visual Studio Code.
Getting Started: The Code Structure
In this post, we will focus on getting user input, processing the data, and then using conditional statements to determine the largest number. Here’s a structured breakdown:
- Import the required packages.
- Initialize variables to store user input.
- Use a scanner to accept input.
- Perform comparisons.
- Output the result.
Here’s a simplified version of the entire code:
import java.util.Scanner;
public class LargestOfThree {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter the first number: ");
int num1 = scanner.nextInt();
System.out.println("Enter the second number: ");
int num2 = scanner.nextInt();
System.out.println("Enter the third number: ");
int num3 = scanner.nextInt();
int largest = findLargest(num1, num2, num3);
System.out.println("The largest number is: " + largest);
scanner.close();
}
// Function to find the largest of three numbers
public static int findLargest(int a, int b, int c) {
int largest = a; // Assume a is the largest
if (b > largest) {
largest = b; // b is the largest so far
}
if (c > largest) {
largest = c; // c is the largest
}
return largest; // Return the largest number
}
}
Explanation of the Code
-
Importing the Scanner: The line
import java.util.Scanner;
allows us to create an instance of theScanner
, which facilitates user input. -
Main Method: Every Java application starts with the
main
method. This method orchestrates the initial setup, gathers input, and invokes other methods. -
Reading User Input:
scanner.nextInt()
reads an integer from the console. We capture values fornum1
,num2
, andnum3
. -
Finding the Largest Number: The
findLargest
method implements our logic. Here’s a closer look at this method:- We initialize
largest
witha
, the first argument. - We compare
b
withlargest
. Ifb
is greater, we updatelargest
. - We repeat the comparison with
c
. - Finally, we return the value of
largest
.
- We initialize
Alternative Approach: Using the Math Class
If you prefer a more concise way to find the largest number, consider using the Math.max()
method. This approach reduces the lines of code as shown below:
import java.util.Scanner;
public class LargestOfThree {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter the first number: ");
int num1 = scanner.nextInt();
System.out.println("Enter the second number: ");
int num2 = scanner.nextInt();
System.out.println("Enter the third number: ");
int num3 = scanner.nextInt();
int largest = Math.max(Math.max(num1, num2), num3);
System.out.println("The largest number is: " + largest);
scanner.close();
}
}
Explanation of the Alternative Approach
- Math.max(): This method simplifies comparisons and allows for cleaner code. We nest
Math.max
calls to first comparenum1
andnum2
, then compare the result withnum3
. - Readability: Using
Math.max
makes the intention clearer, showcasing that we simply desire the maximum value.
Exploring Edge Cases
When working with any code, it’s crucial to consider edge cases. In this scenario, some relevant edge cases might include:
- All three numbers being equal.
- At least two of the numbers being the same.
- Negative integers.
You can test these cases by entering different sets of numbers when prompted.
Example Outputs
Here is how the program behaves with sample inputs:
- Input:
5
,3
,9
-> Output:The largest number is: 9
- Input:
-1
,-5
,-3
-> Output:The largest number is: -1
- Input:
10
,10
,10
-> Output:The largest number is: 10
Final Considerations
Finding the largest of three numbers in Java is a simple yet foundational exercise that reinforces the understanding of variables, data types, user input, and conditional logic. By building upon this basic example, you can tackle more complex programming challenges with confidence.
The approaches discussed—using conditional statements versus leveraging the Math
class—demonstrate different ways to arrive at the same result.
Whether you're a beginner or a seasoned programmer, mastering these fundamentals will set a solid foundation for your Java programming journey. For further learning, consider exploring more advanced topics such as sorting algorithms or data structures.
For more Java programming tutorials and tips, visit the following resources:
Feel free to experiment with the code provided in this blog post. Happy coding!