Avoiding Common Pitfalls in Spring Declarative Transactions
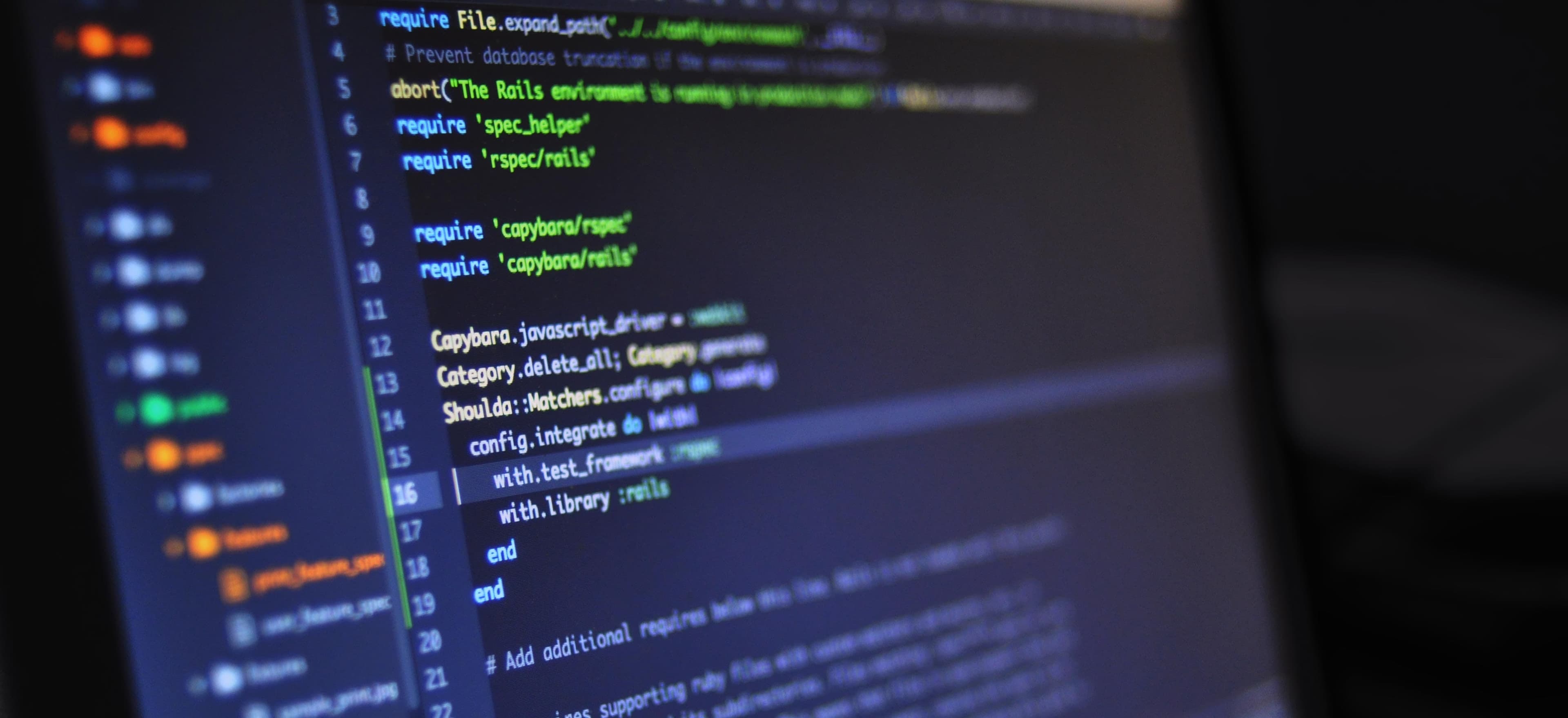
- Published on
Avoiding Common Pitfalls in Spring Declarative Transactions
In the world of enterprise applications, managing transactions effectively is paramount. Transaction management ensures data integrity and consistency, especially in systems interacting with databases. Spring Framework simplifies transaction management, particularly with its declarative transaction support. However, developers frequently encounter pitfalls. In this blog post, we will explore common issues in Spring Declarative Transactions and how to avoid them.
What are Spring Declarative Transactions?
Spring's declarative transaction management allows developers to define transaction boundaries without needing to tightly couple their business logic with transaction management code. Instead of handling transactions programmatically, annotations like @Transactional
are used to demarcate transactional behavior.
For instance:
import org.springframework.transaction.annotation.Transactional;
public class UserService {
@Transactional
public void registerUser(User user) {
userRepository.save(user);
emailService.sendConfirmation(user.getEmail());
}
}
The Importance of Understanding Transaction Management
Understanding transaction management in Spring is crucial for several reasons:
- Data Integrity: When an error occurs within a transaction, Spring can roll back changes to maintain data consistency.
- Performance: Properly configured transactions can improve performance by reducing database locking and contention.
- Readability: Using annotations enhances code readability, making it easier to understand transactional boundaries.
Common Pitfalls in Spring Declarative Transactions
1. Misusing the @Transactional
Annotation
One of the most common pitfalls is misunderstanding where to place the @Transactional
annotation. This annotation must be applied to public methods of a Spring-managed bean. Placing it on private or final methods will lead to unexpected behavior because Spring uses proxies to manage transactions.
Avoid:
@Transactional
private void doInternalWork() {
// This won't work as expected!
}
Better Approach:
@Transactional
public void performAction() {
doInternalWork(); // Transaction managed here
}
private void doInternalWork() {
// Implementation details
}
2. Transactions Not Propagating as Expected
Spring supports various transaction propagation settings— such as REQUIRED
, REQUIRES_NEW
, and more. Using the wrong propagation level can lead to problems, especially in nested transactions. For example, if a method annotated with REQUIRES_NEW
is called from a method that was already marked as @Transactional
, it may lead to unexpected behavior.
Avoid:
@Transactional(propagation = Propagation.REQUIRES_NEW)
public void createNewUser(User user) {
// Creates a new user in a new transaction
}
Better Approach:
Before marking methods with specific propagation settings, evaluate the business scenario. If you only need a single transaction, use the default REQUIRED
. If you need to maintain a separate transaction, ensure proper handling in the calling method.
3. Ignoring Exception Types
Spring's default behavior is to roll back for unchecked exceptions (subtypes of RuntimeException
) but does not roll back for checked exceptions. Ignoring this can lead to inconsistencies if your code throws a checked exception and fails silently.
Avoid:
@Transactional
public void updateUser(Long id, User user) throws IOException {
// Exception does not trigger rollback
userRepository.update(id, user);
}
Better Approach:
If rollbacks for checked exceptions are desired, consider elaborating on your methods to catch exceptions and rethrow them as unchecked:
@Transactional
public void updateUser(Long id, User user) {
try {
userRepository.update(id, user);
} catch (IOException e) {
throw new RuntimeException(e); // Forces rollback
}
}
4. Mixing Spring with JTA (Java Transaction API)
If you're using JTA transactions in addition to Spring's @Transactional
, it can lead to confusion. Spring can manage JTA transactions, but you must set up your data sources properly to avoid conflicts.
For example:
<bean id="jtaTransactionManager" class="org.springframework.transaction.jta.JtaTransactionManager">
<!-- Configuration for JTA transactions -->
</bean>
Make sure your configuration matches your transactional requirements. Mixing transaction management frameworks can cause unexpected behavior.
5. Forgetting to Handle Transactional Boundaries
It is essential to understand that if a method annotated with @Transactional
is called from another method in the same class, the transaction will not be applied. This occurs because Spring AOP (Aspect-Oriented Programming) does not intercept calls from within the same class.
Avoid:
@Transactional
public void process() {
// Transaction here
doWork();
}
private void doWork() {
// No transaction
}
Better Approach:
To ensure that transaction boundaries are maintained, separate the transactional call into another service class.
@Service
public class TransactionalService {
@Autowired
private UserService userService;
@Transactional
public void process() {
userService.doWork();
}
}
// UserService contains business logic without @Transactional
public class UserService {
public void doWork() {
// Business logic
}
}
Closing the Chapter
Spring's declarative transaction management streamlines handling transactions in your applications. However, as we discussed, several common pitfalls can lead to issues if not understood correctly. By employing best practices such as proper annotation placements, understanding propagation settings, managing transaction boundaries, and exception handling, you can ensure effective transaction management in your Spring applications.
For those looking to extend their learning, consider exploring:
- Spring Framework Documentation on Transactions
- Common Patterns for Transactional Services
By avoiding these pitfalls, developers can leverage the powerful features of Spring transactions to create robust, maintainable, and efficient applications. Happy coding!