Why You Should Migrate to JDK 8 Today
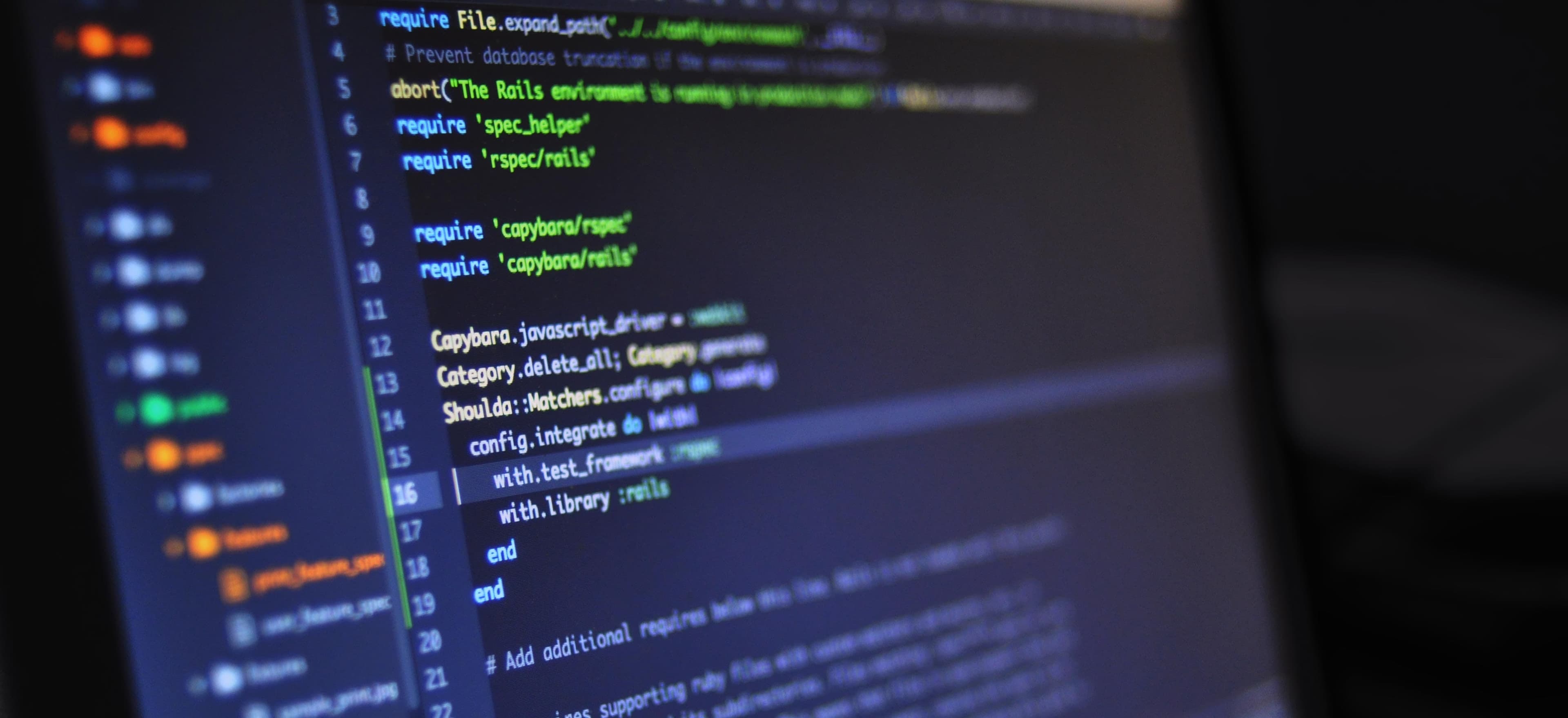
- Published on
Why You Should Migrate to JDK 8 Today
Java Development Kit (JDK) 8 has been a game-changer since its release. Despite being launched in March 2014, many organizations are still using older versions of Java, which not only limits their application's performance but also exposes them to various security vulnerabilities. In this blog post, we will explore the compelling reasons to migrate to JDK 8, illustrate some new features with code examples, and guide you through the advantages that JDK 8 brings.
The Importance of Keeping Up with Technology
Keeping your technology stack updated is crucial for several reasons:
- Performance Enhancements: Newer versions contain optimizations that can make your applications run faster.
- Security Improvements: Each release patches vulnerabilities, reducing the risk of security breaches.
- Access to New Features: You gain access to modern programming paradigms and libraries that enhance the coding experience.
In the case of JDK 8, it introduced several features that changed the way Java developers approach coding. Below are some core highlights.
Key Features of JDK 8
1. Lambda Expressions
Lambda expressions allow you to write concise code, especially when working with collections. They enable you to implement functional-style programming in Java.
import java.util.Arrays;
import java.util.List;
public class LambdaExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Using Lambda Expression to print names
names.forEach(name -> System.out.println(name));
}
}
Why Use It?
Lambda expressions reduce boilerplate code. In the example above, a simple lambda replaces the need for an entire class implementing the Consumer
interface. This leads to cleaner and more readable code.
2. Streams API
The Streams API revolutionizes how you handle collections. You can process data in a functional manner, increasing code efficiency and readability.
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using Streams to filter and print even numbers
numbers.stream()
.filter(n -> n % 2 == 0)
.forEach(System.out::println);
}
}
Why Use It?
Streams allow for complex data manipulations (like filtering and mapping) with simple, declarative code. This not only makes it easier to understand what the code does but also allows for parallel processing, improving performance.
3. Default Methods in Interfaces
JDK 8 allows you to define default methods in interfaces. This can prevent breaking existing code when new methods are added.
interface A {
default void display() {
System.out.println("Display from Interface A");
}
}
class B implements A {
public void display() {
System.out.println("Display from Class B");
}
}
public class DefaultMethodExample {
public static void main(String[] args) {
B b = new B();
b.display();
}
}
Why Use It?
Default methods provide backward compatibility in your interfaces, allowing newer functionalities without forcing all implementing classes to change. This feature promotes a more flexible design.
4. Optional Class
This class provides a way of dealing with null values without the risk of encountering NullPointerException
. It encourages you to write cleaner code.
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
String name = null;
Optional<String> optionalName = Optional.ofNullable(name);
// Using Optional to avoid NullPointerException
String result = optionalName.orElse("Default Name");
System.out.println(result);
}
}
Why Use It?
By using the Optional
class, you can eliminate the confusion around null checks. This leads to safer and more reliable code, encouraging programmers to think about the absence of values explicitly.
5. New Date and Time API
The introduction of the new Date and Time API addresses many issues that Java's old date and time libraries had.
import java.time.LocalDate;
public class DateExample {
public static void main(String[] args) {
// Using LocalDate for date manipulation
LocalDate today = LocalDate.now();
LocalDate birthday = LocalDate.of(2000, 1, 1);
System.out.println("Days until birthday: " + (birthday.toEpochDay() - today.toEpochDay()));
}
}
Why Use It?
The new API is more intuitive and handles complex time zone scenarios much better than the previous java.util.Date
and java.util.Calendar
classes. It promotes immutability, making it less prone to errors.
Benefits of Migrating to JDK 8
1. Modern Language Features
From lambda expressions to the Streams API, JDK 8 brings features that elevate Java coding from a procedural paradigm to a more functional style. This makes it easier to write concurrent code, among other benefits.
2. Strong Community Support
With JDK 8 being widely adopted, you will find extensive community support. The wealth of tutorials, forums, and libraries facilitates the migration process and helps in overcoming potential hurdles.
3. Cost-Effective
Upgrading from an older version of Java might seem daunting due to compatibility issues, but in the long run, JDK 8 allows for more efficient coding and less maintenance time, leading to cost savings.
4. Improved Security
Staying updated with JDK 8 means you will receive the latest security patches. Older versions are often left unsupported, leading to vulnerabilities that hackers may exploit.
5. Better Interoperability
JDK 8 offers better integration with other modern programming paradigms, facilitating the adoption of frameworks such as Spring and Hibernate, which are highly favored in the Java ecosystem.
Preparing for Migration
As with any technology upgrades, a planned approach to migration is essential:
-
Assess Current Applications
Identify which applications are running on older JDKs and evaluate their dependencies. -
Testing Environment
Set up a testing environment to monitor how existing applications behave on JDK 8. Ensure that any processes or libraries in use will work without significant modifications. -
Training Developers
Invest time in training sessions focused on new features in JDK 8, enhancing your team's skills and understanding. -
Gradual Rollout
Start with less critical applications to gain confidence before migrating more critical systems. -
Regular Backups
Ensure that data is backed up before starting the migration process.
For detailed documentation on JDK 8, you can visit Oracle's JDK 8 Documentation.
Closing the Chapter
Migrating to JDK 8 is no longer a decision; it is a necessity. With its plethora of features aimed at reducing complexity while enhancing efficiency and security, the current landscape demands the adoption of newer technologies. If your organization has not yet made the leap, consider this your call to action. The longer you wait, the more you're leaving your systems vulnerable and missing out on enhanced capabilities. Make the switch to JDK 8 today!
By staying engaged with current technologies, you ensure that your projects can take full advantage of the advancements in software development. Happy coding!
Checkout our other articles