Overcoming Serialization Challenges with Google Protocol Buffers
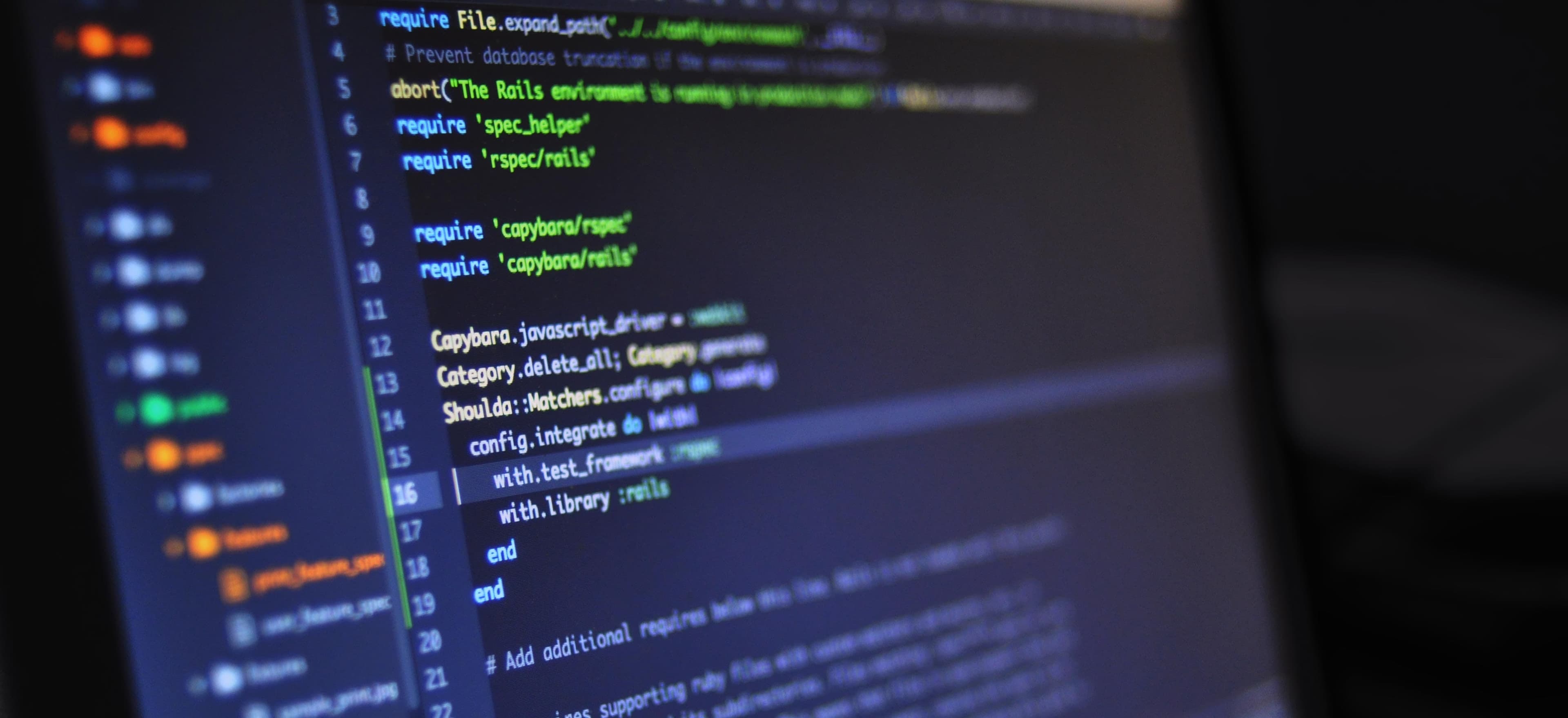
- Published on
Overcoming Serialization Challenges with Google Protocol Buffers
Serialization is a crucial aspect of modern software development. It allows us to convert complex data structures into a format that can be easily stored or transmitted across networks. While there are numerous strategies for serialization, many developers often encounter various challenges that can hinder efficiency and scalability. Among the most potent tools available for addressing these challenges is Google Protocol Buffers (Protobuf).
In this blog post, we will explore the intricacies of serialization, identify common challenges, and illustrate how Protobuf elegantly solves these issues. You will also find actionable code snippets along with thorough explanations to grasp how Protobuf can be integrated into your Java applications.
Understanding Serialization
What is Serialization?
Serialization is the process of converting an object into a byte stream. This transformation makes it easy to save the object to a storage medium or send it over a network. Deserialization, on the other hand, is the reverse process.
Why Serialization Matters
- Data Perpetuation: Enables data persistence in databases.
- Data Transmission: Facilitates communication between microservices or APIs.
- Cross-Platform Compatibility: Supports interactions between different programming languages.
While serialization serves these purposes, it can often lead to performance bottlenecks and challenges related to data integrity.
Common Serialization Challenges
-
Size and Performance: Traditional serialization methods like Java Serialization can lead to large data sizes, which can slow down transmission and processing time.
-
Compatibility: As applications evolve, schema changes may cause compatibility issues. How to ensure backward and forward compatibility in newer versions of data structures?
-
Complexity: Managing nested structures and complex types often becomes cumbersome, specifically when multiple services are involved.
Exploring Google Protocol Buffers (Protobuf)
Google Protocol Buffers is a method developed by Google for serializing structured data. Protobuf addresses the common challenges mentioned above with efficiency, ease of use, and robust support for different programming languages.
Key Features of Protobuf
- Compact Serialization: Protobuf uses a binary format which is significantly smaller compared to XML or JSON.
- Strongly Typed: Protobuf provides compile-time validation which minimizes runtime errors.
- Backward and Forward Compatibility: Schema changes don’t break existing applications if done correctly.
Setting Up Google Protocol Buffers in Java
Before diving into code snippets, let's make sure you have all the prerequisites in place. Ensure you have the Protocol Buffers Compiler (protoc
) set up and Java Plugin installed.
Maven Dependency
If you're using Maven, include the following dependencies in your pom.xml
:
<dependency>
<groupId>com.google.protobuf</groupId>
<artifactId>protobuf-java</artifactId>
<version>3.21.0</version> <!-- Check for newer versions -->
</dependency>
<dependency>
<groupId>com.google.protobuf</groupId>
<artifactId>protobuf-java-util</artifactId>
<version>3.21.0</version>
</dependency>
Defining Your Protobuf Schema
Protobuf uses .proto
files to define the structure of data. Below is an example of a simple .proto
file defining a Person
message:
syntax = "proto3";
message Person {
string name = 1;
int32 id = 2;
string email = 3;
}
Why Use Protobuf?
When you define your data with .proto
, you benefit from strong type-checking and a clear schema definition. Each field has a unique identifier, ensuring consistent serialization and deserialization.
Compiling the .proto
File
Once your schema is defined, compile it using the Protocol Buffers Compiler:
protoc --java_out=. person.proto
This command generates a Person.java
file that contains the necessary code for serialization and deserialization.
Using the Generated Class
With the generated classes, you can easily serialize and deserialize objects. Here’s how to do it in Java.
Serialization Example
import com.example.PersonProto.Person; // Adjust the package accordingly
import java.io.FileOutputStream;
import java.io.IOException;
public class SerializeExample {
public static void main(String[] args) {
Person person = Person.newBuilder()
.setName("John Doe")
.setId(123)
.setEmail("john.doe@example.com")
.build();
try (FileOutputStream outputStream = new FileOutputStream("person.bin")) {
person.writeTo(outputStream);
System.out.println("Serialized data written to file.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Explanation:
- We create a
Person
object using the builder pattern. - The
writeTo
method then serializes the object and writes it to a binary file, which is compact and efficient.
Deserialization Example
Now, let’s read the previously saved data back into a Person
object:
import com.example.PersonProto.Person; // Adjust the package accordingly
import java.io.FileInputStream;
import java.io.IOException;
public class DeserializeExample {
public static void main(String[] args) {
Person person;
try (FileInputStream inputStream = new FileInputStream("person.bin")) {
person = Person.parseFrom(inputStream);
System.out.println("Deserialized data: " + person);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why This Matters:
- The
parseFrom
method reads from the binary file and reconstructs thePerson
object. - This approach is efficient, allowing for easy data retrieval and use without requiring complex parsing logic.
Handling Schema Evolution
One of the prominent strengths of Protobuf is its ability to handle schema evolution without breaking existing implementations. When modifying your .proto
file, consider the following guidelines:
- Never change existing field numbers: Instead, add new fields with unique numbers.
- Default values: New fields should have default values to handle deserialization of older data.
- Use optional fields: Mark new fields as optional to maintain compatibility.
For more in-depth information on best practices, you can check the official Protobuf Guidelines here.
The Closing Argument
Google Protocol Buffers offers an elegant solution to common serialization challenges. Its compact form, type-safety, and ease of use can significantly enhance data handling within Java applications. As applications grow and require complex data exchanges, Protobuf stands out as a robust mechanism to maintain both performance and compatibility.
Embracing Protobuf not only results in better performance but also simplifies how you manage your data schemas. If you are embarking on a new project or seeking to improve an existing one, consider integrating Google Protocol Buffers.
The world of serialized data may be complex, but with the right tools, you can navigate it seamlessly.
For additional resources and a deep dive into serialization techniques, check out the Protobuf Documentation.
Happy coding!
Checkout our other articles